Simple driver for the 20-bit ADC MAX1120x from Maxim
Embed:
(wiki syntax)
Show/hide line numbers
MAX1120x.h
00001 #ifndef __MAX1120X_H__ 00002 #define __MAX1120X_H__ 00003 00004 #include "mbed.h" 00005 00006 // Status flag 00007 #define NOT_READY 1 00008 #define READY 0 00009 00010 // Start bit 00011 #define START (1<<7) 00012 00013 // Mode selection 00014 #define MODE_BIT 6 00015 #define REGISTER_OP_BIT 0 00016 #define mode_register_write(x) ((START | x | (1<<MODE_BIT)) & (~(1<<REGISTER_OP_BIT)) ) 00017 #define mode_register_read(x) (START | x | (1<<MODE_BIT) | (1<<REGISTER_OP_BIT)) 00018 #define mode_action(x) ((START | x) & (~(1<<MODE_BIT)) ) 00019 00020 // Mode 0 (action) Actions table 00021 #define ACT_SELF_CAL 0x10 00022 #define ACT_SYS_OFF_CAL 0x20 00023 #define ACT_SYS_GAN_CAL 0x30 00024 #define ACT_POWERDOWN 0x08 00025 #define ACT_CONV_1SPS 0x00 00026 #define ACT_CONV_2_5SPS 0x01 00027 #define ACT_CONV_5SPS 0x02 00028 #define ACT_CONV_10SPS 0x03 00029 #define ACT_CONV_15SPS 0x04 00030 #define ACT_CONV_30SPS 0x05 00031 #define ACT_CONV_60SPS 0x06 00032 #define ACT_CONV_120SPS 0x07 00033 00034 // Mode 1 (command) Registers table 00035 #define RS3 4 00036 #define RS2 3 00037 #define RS1 2 00038 #define RS0 1 00039 #define REG_STAT1 (0x00) // Status flags 00040 #define REG_CTRL1 ((1<<RS0)) // Converter operation settings 00041 #define REG_CTRL2 ((1<<RS1)) // GPIO pins control 00042 #define REG_CTRL3 ((1<<RS0) | (1<<RS1)) // Gain & Calibration settings 00043 #define REG_DATA ((1<<RS2)) // Sample result 00044 #define REG_SOC ((1<<RS2) | (1<<RS0)) // Offset Sys Calibration value 00045 #define REG_SGC ((1<<RS2) | (1<<RS1)) // Gain Sys Calibration value 00046 #define REG_SCOC ((1<<RS2) | (1<<RS1) | (1<<RS0)) // Offset Self-Calibration value 00047 #define REG_SCGC ((1<<RS3)) // Gain Self-Calibration value 00048 00049 // Registers' bits 00050 #define STAT1_RDY (1<<0) 00051 #define STAT1_MSTAT (1<<1) 00052 #define STAT1_UR (1<<2) 00053 #define STAT1_OR (1<<3) 00054 #define STAT1_RATE0 (1<<4) 00055 #define STAT1_RATE1 (1<<5) 00056 #define STAT1_RATE2 (1<<6) 00057 #define STAT1_SYSOR (1<<7) 00058 00059 #define CTRL1_SCYCLE (1<<1) 00060 #define CTRL1_FORMAT (1<<2) 00061 #define CTRL1_SIGBUF (1<<3) 00062 #define CTRL1_REFBUF (1<<4) 00063 #define CTRL1_EXTCLK (1<<5) 00064 #define CTRL1_UNIP_BIP (1<<6) 00065 #define CTRL1_LINEF (1<<7) 00066 00067 #define CTRL2_DIR_MASK 0xF0 00068 #define CTRL2_DIO_MASK 0x0F 00069 00070 #define CTRL3_DGAIN_MASK 0xE0 00071 #define CTRL3_NOSYSG (1<<4) 00072 #define CTRL3_NOSYSO (1<<3) 00073 #define CTRL3_NOSCG (1<<2) 00074 #define CTRL3_NOSCO (1<<1) 00075 00076 // SPI interface configuration 00077 #define MAX1120x_SPI_MODE 3 00078 00079 00080 typedef unsigned int uint; 00081 00082 union UI32toC_t 00083 { 00084 char bytes[sizeof(unsigned int)]; 00085 unsigned int value; 00086 }; 00087 00088 class MAX1120x 00089 { 00090 SPI *spi; 00091 DigitalOut *cs; 00092 DigitalIn *rdy_dout; 00093 00094 public: 00095 MAX1120x (SPI *, DigitalIn *, DigitalOut *); 00096 00097 // Low-level operations 00098 void do_self_calibration (); 00099 void calibrate_system_zero (); 00100 void calibrate_system_gain (); 00101 char get_status (); 00102 void set_control_1 (char); 00103 char get_control_1 (); 00104 void set_control_2 (char); 00105 char get_control_2 (); 00106 void set_control_3 (char); 00107 char get_control_3 (); 00108 unsigned int get_single_sample (); 00109 unsigned int get_cal_register (char); 00110 00111 // High-level operations 00112 void init_singlecycle_unipolar_nosyscal (); 00113 }; 00114 00115 #endif /*__MAX1120X_H__*/ 00116
Generated on Thu Jul 14 2022 21:19:12 by
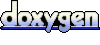