Extended library to include a screensaver
Fork of 4DGL-uLCD-SE by
Embed:
(wiki syntax)
Show/hide line numbers
uLCD_Screensaver.cpp
00001 /** 00002 Screensaver functions for the uLCD_4DGL 00003 */ 00004 #include "mbed.h" 00005 #include "uLCD_4DGL.h" 00006 00007 /** Determine if the screensaver should be shown */ 00008 void uLCD_4DGL :: checkActiveScreenTime() { 00009 time(&now); 00010 if (difftime(now, lastDisplayActivityTime) >= max_active_screen_interval) { 00011 if (!inScreensaverMode) { 00012 enableScreensaverMode(); 00013 } else if (inScreensaverHysteresis) { 00014 inScreensaverHysteresis = false; 00015 } 00016 } 00017 } 00018 00019 /** Whenever a change is made to the LCD screen that is not part of the 00020 screensaver graphics method, it is reported and the last screen 00021 activity time is updated */ 00022 void uLCD_4DGL :: reportScreenInteraction() { 00023 time(&lastDisplayActivityTime); // indicate that the screen is still active 00024 if (inScreensaverMode) { 00025 exitScreensaverMode(); 00026 } 00027 } 00028 00029 /** Initialize the screen saver */ 00030 void uLCD_4DGL :: enableScreensaverMode() { 00031 inScreensaverMode = true; 00032 backupStateOfScreen(); 00033 inScreensaverHysteresis = true; 00034 cls(); 00035 #if MYDEBUG 00036 pc.printf("Going into screensaver mode\r\n"); 00037 #endif 00038 } 00039 00040 /** Close the screen saver */ 00041 void uLCD_4DGL :: exitScreensaverMode() { 00042 inScreensaverMode = false; 00043 restoreStateOfScreen(); 00044 inScreensaverHysteresis = false; 00045 #if MYDEBUG 00046 pc.printf("Going out of screensaver mode\r\n"); 00047 #endif 00048 } 00049 00050 /** Set the timeout interval for active screen */ 00051 void uLCD_4DGL :: setActiveScreenInterval(int newInterval) { 00052 max_active_screen_interval = newInterval; 00053 } 00054 00055 /** Get the timeout interval for active screen */ 00056 int uLCD_4DGL :: getActiveScreenInterval() { 00057 return max_active_screen_interval; 00058 } 00059 00060 /**Return whether the LCD is currently in screen saver mode */ 00061 bool uLCD_4DGL :: isInScreensaverMode() { 00062 return inScreensaverMode; 00063 } 00064 00065 /** Backup the current pixels of the display to the sd card */ 00066 void uLCD_4DGL :: backupStateOfScreen() { 00067 FILE* data = fopen("/sd/pixelData.txt","w"); 00068 fclose(data); 00069 data = fopen("/sd/pixelData.txt","a"); 00070 if (data == NULL) { 00071 pc.printf("Data file failed to open!\r\n"); 00072 } 00073 for (int x = 0; x < 127; x+=2) { 00074 for (int y = 0; y < 127; y+=2) { 00075 int color = read_pixel(x, y); 00076 if (color > 0 && color != 1536 && color != 1538) { 00077 fprintf(data, "%i %i %i\n", x, y, color); 00078 } 00079 00080 } 00081 } 00082 fclose(data); 00083 } 00084 00085 // Retrive the backed-up pixel display 00086 // from the sd card 00087 void uLCD_4DGL :: restoreStateOfScreen() { 00088 cls(); 00089 const int CHARS = 16; 00090 const int TOKENS = 4; 00091 const char* const delim = " "; 00092 00093 std::ifstream fin; 00094 fin.open("/sd/pixelData.txt"); 00095 00096 while(!fin.eof()) { 00097 // int char int char int \n 00098 // 4 + 1 + 4 + 1 + 4 + 1 00099 char buf[CHARS]; 00100 fin.getline(buf, CHARS); 00101 const char* token[TOKENS] = {}; 00102 00103 int i=0; 00104 int color; 00105 token[0] = strtok(buf, delim); 00106 if(token[0]) { 00107 for(i=1; i<TOKENS; i++) { 00108 token[i] = strtok(0, delim); 00109 if(!token[i]) break; 00110 color = atoi(token[2]); 00111 if (color > 0 && color != 1536 && color != 1538) { 00112 int red5 = (color >> 11) & 0x1F; 00113 int green6 = (color >> 5) & 0x1F; 00114 int blue5 = (color >> 0) & 0x1F; 00115 color = 0; 00116 color |= blue5 << 0 + 3; 00117 color |= green6 << 2 + 8; 00118 color |= red5 << 16 + 3; 00119 pixel(atoi(token[0]), atoi(token[1]), color); 00120 } else { 00121 pixel(atoi(token[0]), atoi(token[1]), 0); 00122 } 00123 } 00124 } 00125 } 00126 fin.close(); 00127 }
Generated on Fri Jul 15 2022 01:44:25 by
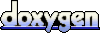