
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "i2s.h" 00003 #include "type.h" 00004 #include "dma.h" 00005 00006 extern volatile uint8_t *I2STXBuffer, *I2SRXBuffer; 00007 extern volatile uint32_t I2SReadLength; 00008 extern volatile uint32_t I2SWriteLength; 00009 extern volatile uint32_t I2SRXDone, I2STXDone; 00010 extern volatile uint32_t I2SDMA0Done, I2SDMA1Done; 00011 00012 DigitalOut myled(LED1); 00013 00014 00015 int main() { 00016 uint32_t i; 00017 /* Configure temp register before reading */ 00018 for ( i = 0; i < BUFSIZE; i++ ) { /* clear buffer */ 00019 I2STXBuffer[i] = i; 00020 I2SRXBuffer[i] = 0; 00021 } 00022 00023 if ( I2SInit() == FALSE ) { /* initialize I2S */ 00024 while ( 1 ); /* Fatal error */ 00025 } 00026 00027 #if I2S_DMA_ENABLED 00028 /* USB RAM is used for test. 00029 Please note, Ethernet has its own SRAM, but GPDMA can't access 00030 that. GPDMA can access USB SRAM and IRAM. Ethernet DMA controller can 00031 access both IRAM and Ethernet SRAM. */ 00032 LPC_SC->PCONP |= (1 << 29); /* Enable GPDMA clock */ 00033 00034 LPC_GPDMA->DMACIntTCClear = 0x03; 00035 LPC_GPDMA->DMACIntErrClr = 0x03; 00036 00037 LPC_GPDMA->DMACConfig = 0x01; /* Enable DMA channels, little endian */ 00038 while ( !(LPC_GPDMA->DMACConfig & 0x01) ); 00039 00040 /* on DMA channel 0, Source is memory, destination is I2S TX FIFO, 00041 on DMA channel 1, source is I2S RX FIFO, Destination is memory */ 00042 /* Enable channel and IE bit */ 00043 DMA_Init( 0, M2P ); 00044 LPC_GPDMACH0->DMACCConfig |= 0x18001 | (0x00 << 1) | (0x05 << 6) | (0x01 << 11); 00045 DMA_Init( 1, P2M ); 00046 LPC_GPDMACH1->DMACCConfig |= 0x08001 | (0x06 << 1) | (0x00 << 6) | (0x02 << 11); 00047 00048 NVIC_EnableIRQ(DMA_IRQn); 00049 00050 I2SStart(); 00051 00052 LPC_I2S->I2SDMA2 = (0x01<<0) | (0x08<<8); /* Channel 2 is for RX, enable RX first. */ 00053 LPC_I2S->I2SDMA1 = (0x01<<1) | (0x01<<16);/* Channel 1 is for TX. */ 00054 00055 /* Wait for both DMA0 and DMA1 to finish before verifying. */ 00056 while ( !I2SDMA0Done || !I2SDMA1Done ); 00057 #else 00058 /* Not DMA mode, enable I2S interrupts. */ 00059 /* RX FIFO depth is 1, TX FIFO depth is 8. */ 00060 I2SStart(); 00061 LPC_I2S->I2SIRQ = (8 << 16) | (1 << 8) | (0x01 << 0); 00062 00063 while ( I2SWriteLength < BUFSIZE ) { 00064 while (((LPC_I2S->I2SSTATE >> 16) & 0xFF) == TXFIFO_FULL); 00065 LPC_I2S->I2STXFIFO = I2STXBuffer[I2SWriteLength++]; 00066 } 00067 00068 I2STXDone = 1; 00069 /* Wait for RX and TX complete before comparison */ 00070 while ( !I2SRXDone || !I2STXDone ); 00071 #endif 00072 00073 /* Validate TX and RX buffer */ 00074 for ( i=1; i<BUFSIZE; i++ ) { 00075 if ( I2SRXBuffer[i] != I2STXBuffer[i-1] ) { 00076 while ( 1 ); /* Validation error */ 00077 } 00078 } 00079 return 0; 00080 }
Generated on Thu Jul 14 2022 10:56:38 by
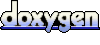