
A neopixel light painting strip
Dependencies: NeoStrip PinDetect mbed
BitmapFile.h
00001 /**********************************************************/ 00002 /*BitmapFile.h */ 00003 /**********************************************************/ 00004 00005 #include "mbed.h" 00006 00007 /* Class: BitmapFile 00008 * A parser for bitmap files. 00009 */ 00010 class BitmapFile 00011 { 00012 private: 00013 FILE *m_pFile; 00014 char *m_fileName; 00015 int m_rowsize; 00016 00017 enum headerType { BITMAPCOREHEADER = 12, 00018 BITMAPCOREHEADER2 = 64, 00019 BITMAPINFOHEADER = 40, 00020 BITMAPV4HEADER = 108, 00021 BITMAPV5HEADER = 124}; 00022 00023 enum compressType { BI_RGB, 00024 BI_RLE8, 00025 BI_RLE4, 00026 BI_BITFIELDS, 00027 BI_JPEG, 00028 BI_PNG}; 00029 00030 /* Struct: BMPHeader 00031 * 00032 * The BMP header of the bitmap is read into this. 00033 * 00034 * b - the first byte of the header. Should equal 'B'. 00035 * m - the second byte of the header. Should equal 'M'. 00036 * filesize - the size of the whole file, in bytes. 00037 * reserved 1 and 2 - data specific to the applicaton which created the bitmap. 00038 * offset - the offset at which the actual bitmap begins 00039 */ 00040 00041 __packed struct 00042 { 00043 char b:8; 00044 char m:8; 00045 int filesize:32; 00046 int reserved1:16; 00047 int reserved2:16; 00048 int offset:32; 00049 00050 } BMPHeader; 00051 00052 00053 /* Struct: DIBHeader 00054 * 00055 * The DIB header of the bitmap is read into this. 00056 * 00057 * headerlength - the length of the header. Should equal 40. 00058 * height - the height of the bitmap. 00059 * width - the width of the bitmap. 00060 * cplanes - the number of color planes. Should equal 1. 00061 * colordepth - the number of bits per pixel. 00062 * compression - the compression method used. 00063 * datasize - the size of the bitmap data, in bytes. 00064 */ 00065 00066 int m_headerlength; 00067 __packed struct 00068 { 00069 int width:32; 00070 int height:32; 00071 int cplanes:16; 00072 int colordepth:16; 00073 int compression:32; 00074 int datasize:32; 00075 int hres:32; 00076 int vres:32; 00077 int numpalettecolors:32; 00078 int importantcolors:32; 00079 } DIBHeader; 00080 00081 00082 public: 00083 /* Constructor: BitmapFile 00084 * Create the BitmapFile class, and call <Initialize> 00085 * 00086 * Parameters: 00087 * fname - The path of the file to open. 00088 */ 00089 BitmapFile(char* fname); 00090 ~BitmapFile(); 00091 00092 /* Function: Initialize 00093 * Parses the headers of the bitmap. 00094 * 00095 * Returns: 00096 * Whether the bitmap is valid. 00097 */ 00098 bool Initialize(); //parses the header 00099 00100 /* Function: open 00101 * Opens the bitmap for reading, if not already open. 00102 */ 00103 void open(); 00104 00105 /* Function: close 00106 * Closes the bitmap. 00107 */ 00108 void close(); 00109 00110 /***BMP Header gets begin***/ 00111 int getFileSize(); 00112 int getReserved1(); 00113 int getReserved2(); 00114 int getOffset(); 00115 00116 /***DIB Header gets begin***/ 00117 int getHeaderType(); 00118 int getHeight(); 00119 int getWidth(); 00120 int getCPlanes(); 00121 int getColorDepth(); 00122 int getCompression(); 00123 int getDataSize(); 00124 int getHRes(); 00125 int getVRes(); 00126 int getNumPaletteColors(); 00127 int getImportantColors(); 00128 /****DIB Header gets end****/ 00129 00130 /******Data gets begin******/ 00131 /* Function: getPixel 00132 * Gets the color of a pixel 00133 * 00134 * Parameters: 00135 * x - The x coordinate of the pixel. 00136 * y - The y coordinate of the pixel. 00137 * closefile - if specified, close the file after reading 00138 * 00139 * Returns: 00140 * the color of the pixel, in hexadecimal. 00141 */ 00142 int getPixel(int x, int y, bool closefile = true); 00143 /* Function: getRow 00144 * Gets the colors of a row 00145 * 00146 * Parameters: 00147 * row - The number of the row.. 00148 * closefile - if specified, close the file after reading 00149 * 00150 * Returns: 00151 * An array of the colors of the pixels, in hexadecimal. 00152 */ 00153 int *getRow(int row, bool closefile = true); 00154 int *getRowBW(int row, bool closefile = true); 00155 char *getRowBitstream(int row, bool closefile = true); 00156 /*******Data gets end*******/ 00157 int getRowSize(); 00158 };
Generated on Fri Jul 22 2022 22:17:39 by
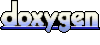