
For BU9480F D-A Conv.
Embed:
(wiki syntax)
Show/hide line numbers
_bitio.cpp
00001 #include "mbed.h" 00002 #include "string" 00003 #include "_bitio.h" 00004 00005 00006 FILE *infp, *outfp; 00007 int getcount, putcount; 00008 Uint bitbuf; 00009 00010 void init_bit_o(void) 00011 { 00012 putcount = 8; bitbuf = 0; 00013 } 00014 00015 void init_bit_i(void) 00016 { 00017 getcount = 0; bitbuf = 0; 00018 } 00019 00020 00021 Uint getbit(void) 00022 { 00023 int flag; 00024 if (--getcount >= 0) return (bitbuf >> getcount) & 1U; 00025 getcount = 7; 00026 if((flag = fgetc(infp))==EOF)return OVERRUN; 00027 else bitbuf = flag; 00028 return (bitbuf >> 7) & 1U; 00029 } 00030 00031 Uint getbits(int n) 00032 { 00033 Uint x = 0; 00034 if (n < 1 || 25 < n) return 0; 00035 while (n > getcount) { 00036 n -= getcount; 00037 x |= rightbits(getcount, bitbuf) << n; 00038 bitbuf = fgetc(infp); getcount = 8; 00039 } 00040 getcount -= n; 00041 return x | rightbits(n, bitbuf >> getcount); 00042 } 00043 00044 00045 void putbit(Uint bit) 00046 { 00047 putcount--; 00048 if (bit != 0) bitbuf |= (1 << putcount); 00049 if (putcount == 0) { 00050 if (fputc(bitbuf, outfp) == EOF) error("can't write\r\n"); 00051 bitbuf = 0; putcount = 8; 00052 } 00053 } 00054 00055 00056 void putbits(int n, Uint x) 00057 { 00058 if (n < 1 || 25 < n) return; 00059 while (n >= putcount) { 00060 n -= putcount; 00061 bitbuf |= rightbits(putcount, x >> n); 00062 if (fputc(bitbuf, outfp) == EOF) error("cant write\r\n"); 00063 bitbuf = 0U; putcount = 8; 00064 } 00065 putcount -= n; 00066 bitbuf |= rightbits(n, x) << putcount; 00067 }
Generated on Sat Jul 16 2022 20:06:49 by
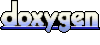