
OneWireCRC
Fork of OneWireCRC by
Embed:
(wiki syntax)
Show/hide line numbers
DebugTrace.cpp
00001 /* 00002 * DebugTrace. Allows dumping debug messages/values to serial or 00003 * to file. 00004 * 00005 * Copyright (C) <2009> Petras Saduikis <petras@petras.co.uk> 00006 * 00007 * This file is part of DebugTrace. 00008 * 00009 * DebugTrace is free software: you can redistribute it and/or modify 00010 * it under the terms of the GNU General Public License as published by 00011 * the Free Software Foundation, either version 3 of the License, or 00012 * (at your option) any later version. 00013 * 00014 * DebugTrace is distributed in the hope that it will be useful, 00015 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00016 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00017 * GNU General Public License for more details. 00018 * 00019 * You should have received a copy of the GNU General Public License 00020 * along with DebugTrace. If not, see <http://www.gnu.org/licenses/>. 00021 */ 00022 00023 #include "DebugTrace.h" 00024 #include <mbed.h> 00025 #include <stdarg.h> 00026 #include <string.h> 00027 00028 Serial logSerial(USBTX, USBRX); 00029 LocalFileSystem local("local"); 00030 00031 const char* FILE_PATH = "/local/"; 00032 const char* EXTN = ".bak"; 00033 00034 DebugTrace::DebugTrace(eLog on, eLogTarget mode, const char* fileName, int maxSize) : 00035 enabled(on), logMode(mode), maxFileSize(maxSize), currentFileSize(0), 00036 logFileStatus(0) 00037 { 00038 // allocate memory for file name strings 00039 int str_size = (strlen(fileName) + strlen(FILE_PATH) + strlen(EXTN) + 1) * sizeof(char); 00040 logFile = (char*)malloc(str_size); 00041 logFileBackup = (char*)malloc(str_size); 00042 00043 // add path to log file name 00044 strcpy(logFile, FILE_PATH); 00045 strcat(logFile, fileName); 00046 00047 // create backup file name 00048 strcpy(logFileBackup, logFile); 00049 strcpy(logFileBackup, strtok(logFileBackup, ".")); 00050 strcat(logFileBackup, EXTN); 00051 } 00052 00053 DebugTrace::~DebugTrace() 00054 { 00055 // dust to dust, ashes to ashes 00056 if (logFile != NULL) free(logFile); 00057 if (logFileBackup != NULL) free(logFileBackup); 00058 } 00059 00060 void DebugTrace::clear() 00061 { 00062 // don't care about whether these fail 00063 remove(logFile); 00064 remove(logFileBackup); 00065 } 00066 00067 void DebugTrace::backupLog() 00068 { 00069 // delete previous backup file 00070 if (remove(logFileBackup)) 00071 { 00072 // standard copy stuff 00073 char ch; 00074 FILE* to = fopen(logFileBackup, "wb"); 00075 if (NULL != to) 00076 { 00077 FILE* from = fopen(logFile, "rb"); 00078 if (NULL != from) 00079 { 00080 while(!feof(from)) 00081 { 00082 ch = fgetc(from); 00083 if (ferror(from)) break; 00084 00085 if(!feof(from)) fputc(ch, to); 00086 if (ferror(to)) break; 00087 } 00088 } 00089 00090 if (NULL != from) fclose(from); 00091 if (NULL != to) fclose(to); 00092 } 00093 } 00094 00095 // now delete the log file, so we are ready to start again 00096 // even if backup creation failed - the show must go on! 00097 logFileStatus = remove(logFile); 00098 } 00099 00100 void DebugTrace::traceOut(const char* fmt, ...) 00101 { 00102 if (enabled) 00103 { 00104 va_list ap; // argument list pointer 00105 va_start(ap, fmt); 00106 00107 if (TO_SERIAL == logMode) 00108 { 00109 vfprintf(logSerial, fmt, ap); 00110 } 00111 else // TO_FILE 00112 { 00113 if (0 == logFileStatus) // otherwise we failed to remove a full log file 00114 { 00115 // Write data to file. Note the file size may go over limit 00116 // as we check total size afterwards, using the size written to file. 00117 // This is not a big issue, as this mechanism is only here 00118 // to stop the file growing unchecked. Just remember log file sizes may 00119 // be some what over (as apposed to some what under), so don't push it 00120 // with the max file size. 00121 FILE* fp = fopen(logFile, "a"); 00122 if (NULL == fp) 00123 { 00124 va_end(ap); 00125 return; 00126 } 00127 int size_written = vfprintf(fp, fmt, ap); 00128 fclose(fp); 00129 00130 // check if we are over the max file size 00131 // if so backup file and start again 00132 currentFileSize += size_written; 00133 if (currentFileSize >= maxFileSize) 00134 { 00135 backupLog(); 00136 currentFileSize = 0; 00137 } 00138 } 00139 } 00140 00141 va_end(ap); 00142 } 00143 }
Generated on Sat Jul 16 2022 12:23:48 by
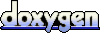