Dallas' 1-Wire bus protocol library using mbed debug logs
Fork of OneWire by
OneWire.h
00001 #ifndef OneWire_h 00002 #define OneWire_h 00003 00004 #include <inttypes.h> 00005 #include <mbed.h> 00006 00007 // You can exclude certain features from OneWire. In theory, this 00008 // might save some space. In practice, the compiler automatically 00009 // removes unused code (technically, the linker, using -fdata-sections 00010 // and -ffunction-sections when compiling, and Wl,--gc-sections 00011 // when linking), so most of these will not result in any code size 00012 // reduction. Well, unless you try to use the missing features 00013 // and redesign your program to not need them! ONEWIRE_CRC8_TABLE 00014 // is the exception, because it selects a fast but large algorithm 00015 // or a small but slow algorithm. 00016 00017 // you can exclude onewire_search by defining that to 0 00018 #ifndef ONEWIRE_SEARCH 00019 #define ONEWIRE_SEARCH 1 00020 #endif 00021 00022 // You can exclude CRC checks altogether by defining this to 0 00023 #ifndef ONEWIRE_CRC 00024 #define ONEWIRE_CRC 1 00025 #endif 00026 00027 class OneWire 00028 { 00029 private: 00030 DigitalInOut wire; 00031 00032 #if ONEWIRE_SEARCH 00033 // global search state 00034 unsigned char ROM_NO[8]; 00035 uint8_t LastDiscrepancy; 00036 uint8_t LastFamilyDiscrepancy; 00037 uint8_t LastDeviceFlag; 00038 #endif 00039 00040 public: 00041 OneWire(PinName pin); 00042 00043 // Perform a 1-Wire reset cycle. Returns 1 if a device responds 00044 // with a presence pulse. Returns 0 if there is no device or the 00045 // bus is shorted or otherwise held low for more than 250uS 00046 uint8_t reset(void); 00047 00048 // Issue a 1-Wire rom select command, you do the reset first. 00049 void select(const uint8_t rom[8]); 00050 00051 // Issue a 1-Wire rom skip command, to address all on bus. 00052 void skip(void); 00053 00054 // Write a byte. If 'power' is one then the wire is held high at 00055 // the end for parasitically powered devices. You are responsible 00056 // for eventually depowering it by calling depower() or doing 00057 // another read or write. 00058 void write(uint8_t v, uint8_t power = 0); 00059 00060 void write_bytes(const uint8_t *buf, uint16_t count, bool power = 0); 00061 00062 // Read a byte. 00063 uint8_t read(void); 00064 00065 void read_bytes(uint8_t *buf, uint16_t count); 00066 00067 // Write a bit. The bus is always left powered at the end, see 00068 // note in write() about that. 00069 void write_bit(uint8_t v); 00070 00071 // Read a bit. 00072 uint8_t read_bit(void); 00073 00074 // Stop forcing power onto the bus. You only need to do this if 00075 // you used the 'power' flag to write() or used a write_bit() call 00076 // and aren't about to do another read or write. You would rather 00077 // not leave this powered if you don't have to, just in case 00078 // someone shorts your bus. 00079 void depower(void); 00080 00081 #if ONEWIRE_SEARCH 00082 // Clear the search state so that if will start from the beginning again. 00083 void reset_search(); 00084 00085 // Setup the search to find the device type 'family_code' on the next call 00086 // to search(*newAddr) if it is present. 00087 void target_search(uint8_t family_code); 00088 00089 // Look for the next device. Returns 1 if a new address has been 00090 // returned. A zero might mean that the bus is shorted, there are 00091 // no devices, or you have already retrieved all of them. It 00092 // might be a good idea to check the CRC to make sure you didn't 00093 // get garbage. The order is deterministic. You will always get 00094 // the same devices in the same order. 00095 uint8_t search(uint8_t *newAddr); 00096 #endif 00097 00098 #if ONEWIRE_CRC 00099 // Compute a Dallas Semiconductor 8 bit CRC, these are used in the 00100 // ROM and scratchpad registers. 00101 static uint8_t crc8(const uint8_t *addr, uint8_t len); 00102 00103 #if ONEWIRE_CRC16 00104 // Compute the 1-Wire CRC16 and compare it against the received CRC. 00105 // Example usage (reading a DS2408): 00106 // // Put everything in a buffer so we can compute the CRC easily. 00107 // uint8_t buf[13]; 00108 // buf[0] = 0xF0; // Read PIO Registers 00109 // buf[1] = 0x88; // LSB address 00110 // buf[2] = 0x00; // MSB address 00111 // WriteBytes(net, buf, 3); // Write 3 cmd bytes 00112 // ReadBytes(net, buf+3, 10); // Read 6 data bytes, 2 0xFF, 2 CRC16 00113 // if (!CheckCRC16(buf, 11, &buf[11])) { 00114 // // Handle error. 00115 // } 00116 // 00117 // @param input - Array of bytes to checksum. 00118 // @param len - How many bytes to use. 00119 // @param inverted_crc - The two CRC16 bytes in the received data. 00120 // This should just point into the received data, 00121 // *not* at a 16-bit integer. 00122 // @param crc - The crc starting value (optional) 00123 // @return True, iff the CRC matches. 00124 static bool check_crc16(const uint8_t* input, uint16_t len, const uint8_t* inverted_crc, uint16_t crc = 0); 00125 00126 // Compute a Dallas Semiconductor 16 bit CRC. This is required to check 00127 // the integrity of data received from many 1-Wire devices. Note that the 00128 // CRC computed here is *not* what you'll get from the 1-Wire network, 00129 // for two reasons: 00130 // 1) The CRC is transmitted bitwise inverted. 00131 // 2) Depending on the endian-ness of your processor, the binary 00132 // representation of the two-byte return value may have a different 00133 // byte order than the two bytes you get from 1-Wire. 00134 // @param input - Array of bytes to checksum. 00135 // @param len - How many bytes to use. 00136 // @param crc - The crc starting value (optional) 00137 // @return The CRC16, as defined by Dallas Semiconductor. 00138 static uint16_t crc16(const uint8_t* input, uint16_t len, uint16_t crc = 0); 00139 #endif 00140 #endif 00141 }; 00142 00143 #endif
Generated on Sat Jul 16 2022 12:50:38 by
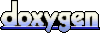