
a
Dependencies: BSP_B-L475E-IOT01
main.cpp
00001 00002 #include "mbed.h" 00003 #include <stdio.h> 00004 #include <errno.h> 00005 #include "stm32l475e_iot01_accelero.h" 00006 #include "nvstore.h" 00007 00008 // Block devices 00009 #if COMPONENT_SPIF 00010 #include "SPIFBlockDevice.h" 00011 #endif 00012 00013 #if COMPONENT_DATAFLASH 00014 #include "DataFlashBlockDevice.h" 00015 #endif 00016 00017 #if COMPONENT_SD 00018 #include "SDBlockDevice.h" 00019 #endif 00020 00021 #include "HeapBlockDevice.h" 00022 // File systems 00023 #include "LittleFileSystem.h" 00024 #include "FATFileSystem.h" 00025 00026 DigitalOut led1(LED1); 00027 DigitalOut led2(LED2); 00028 DigitalOut led3(LED3); 00029 Ticker toggle_led; 00030 00031 EventQueue queue(32 * EVENTS_EVENT_SIZE); 00032 Thread thread; 00033 int maximum( int, int, int); 00034 //int maximum( int a, int b, int c ); 00035 00036 // Physical block device, can be any device that supports the BlockDevice API 00037 /*SPIFBlockDevice bd( 00038 MBED_CONF_SPIF_DRIVER_SPI_MOSI, 00039 MBED_CONF_SPIF_DRIVER_SPI_MISO, 00040 MBED_CONF_SPIF_DRIVER_SPI_CLK, 00041 MBED_CONF_SPIF_DRIVER_SPI_CS);*/ 00042 00043 #define BLOCK_SIZE 512 00044 HeapBlockDevice bd(16384, BLOCK_SIZE); 00045 00046 // File system declaration 00047 LittleFileSystem fs("fs"); 00048 00049 // Set up the button to trigger an erase 00050 InterruptIn irq(BUTTON1); 00051 void erase() { 00052 printf("Initializing the block device... "); 00053 fflush(stdout); 00054 int err = bd.init(); 00055 printf("%s\n", (err ? "Fail :(" : "OK")); 00056 if (err) { 00057 error("error: %s (%d)\n", strerror(-err), err); 00058 } 00059 00060 printf("Erasing the block device... "); 00061 fflush(stdout); 00062 err = bd.erase(0, bd.size()); 00063 printf("%s\n", (err ? "Fail :(" : "OK")); 00064 if (err) { 00065 error("error: %s (%d)\n", strerror(-err), err); 00066 } 00067 00068 printf("Deinitializing the block device... "); 00069 fflush(stdout); 00070 err = bd.deinit(); 00071 printf("%s\n", (err ? "Fail :(" : "OK")); 00072 if (err) { 00073 error("error: %s (%d)\n", strerror(-err), err); 00074 } 00075 } 00076 00077 static FILE *file; 00078 volatile int counter = 0; 00079 00080 int rc; 00081 00082 uint16_t keyX = 1; 00083 uint16_t keyY = 2; 00084 uint16_t keyZ = 3; 00085 00086 uint32_t valueX; 00087 uint32_t valueX; 00088 uint32_t valueZ; 00089 void getDataFromSensors() { 00090 int16_t pDataXYZ[3] = {0}; 00091 BSP_ACCELERO_AccGetXYZ(pDataXYZ); 00092 00093 bool isHorizontal = pDataXYZ[2] > 900 || pDataXYZ[2] < -900; 00094 bool isOnLongEdge = pDataXYZ[1] > 900 || pDataXYZ[1] < -900; 00095 bool isOnShortEdge = pDataXYZ[0] > 900 || pDataXYZ[0] < -900; 00096 00097 if(isHorizontal) { 00098 fprintf(file, "%d\n", 1); 00099 } else if(isOnLongEdge) { 00100 fprintf(file, "%d\n", 2); 00101 } else if(isOnShortEdge) { 00102 fprintf(file, "%d\n", 3); 00103 } else { 00104 fprintf(file, "%d\n", 4); 00105 } 00106 fflush(file); 00107 fflush(stdout); 00108 } 00109 00110 void readFile() { 00111 00112 int countLed1 = 0; 00113 int countLed2 = 0; 00114 int countLed3 = 0; 00115 00116 fflush(stdout); 00117 fflush(file); 00118 00119 fseek(file, 0, SEEK_SET); 00120 int ledNumber; 00121 while(!feof(file)) { 00122 fscanf(file, "%d", &ledNumber); 00123 if(ledNumber == 1){ 00124 countLed1 += 1; 00125 } else if(ledNumber == 2){ 00126 countLed2 += 1; 00127 } else if(ledNumber == 3){ 00128 countLed3 += 1; 00129 } 00130 } 00131 00132 bool isLed1 = led1 >= led2 && led1 >= led3; 00133 bool isLed2 = led2 >= led1 && led2 >= led3; 00134 bool isLed3 = led3 >= led1 && led3 >= led2; 00135 00136 NVStore &nvstore = NVStore::get_instance(); 00137 00138 if(isLed1) { 00139 led1 = 1; 00140 led2 = 0; 00141 led3 = 0; 00142 valueX += 1; 00143 nvstore.set(keyX, sizeof(valueX), &valueX); 00144 } else if (isLed2) { 00145 led1 = 0; 00146 led2 = 1; 00147 led3 = 0; 00148 valueY += 1; 00149 nvstore.set(keyY, sizeof(valueY), &valueY); 00150 } else if (isLed3) { 00151 led1 = 0; 00152 led2 = 0; 00153 led3 = 1; 00154 valueZ += 1; 00155 nvstore.set(keyZ, sizeof(valueZ), &valueZ); 00156 } 00157 00158 00159 fflush(stdout); 00160 int err = fclose(file); 00161 printf("%s\n", (err < 0 ? "Fail :(" : "OK")); 00162 if (err < 0) { 00163 error("error: %s (%d)\n", strerror(err), -err); 00164 } 00165 err = fs.unmount(); 00166 printf("%s\n", (err < 0 ? "Fail :(" : "OK")); 00167 if (err < 0) { 00168 error("error: %s (%d)\n", strerror(-err), err); 00169 } 00170 00171 printf("Mbed OS filesystem example done!\n"); 00172 } 00173 00174 void toggleLed() { 00175 queue.call(readSensors); 00176 counter += 1; 00177 if(counter == 1000) { 00178 toggle_led.detach(); 00179 queue.call(readFile); 00180 } 00181 } 00182 00183 00184 // Entry point for the example 00185 int main() { 00186 thread.start(callback(&queue, &EventQueue::dispatch_forever)); 00187 BSP_ACCELERO_Init(); 00188 00189 NVStore &nvstore = NVStore::get_instance(); 00190 rc = nvstore.init(); 00191 uint16_t actual_len_bytes = 0; 00192 rc = nvstore.get(keyX, sizeof(valueX), &valueX, actual_len_bytes); 00193 00194 if(rc == NVSTORE_NOT_FOUND) { 00195 valueX = 0; 00196 valueY = 0; 00197 valueZ = 0; 00198 nvstore.set(keyX, sizeof(valueX), &valueX); 00199 nvstore.set(keyY, sizeof(valueY), &valueY); 00200 nvstore.set(keyZ, sizeof(valueZ), &valueZ); 00201 } else { 00202 nvstore.get(keyZ, sizeof(valueZ), &valueZ, actual_len_bytes); 00203 nvstore.get(keyY, sizeof(valueY), &valueY, actual_len_bytes); 00204 } 00205 00206 printf("Led1 Count: %d -- Led2 Count: %d -- Led3 Count: %d \n", valueX, valueY, valueZ); 00207 printf("--- Mbed OS filesystem example ---\n"); 00208 00209 // Setup the erase event on button press, use the event queue 00210 // to avoid running in interrupt context 00211 irq.fall(mbed_event_queue()->event(erase)); 00212 00213 // Try to mount the filesystem 00214 printf("Mounting the filesystem... "); 00215 fflush(stdout); 00216 int err = fs.mount(&bd); 00217 printf("%s\n", (err ? "Fail :(" : "OK")); 00218 if (err) { 00219 // Reformat if we can't mount the filesystem 00220 // this should only happen on the first boot 00221 printf("No filesystem found, formatting... "); 00222 fflush(stdout); 00223 err = fs.reformat(&bd); 00224 printf("%s\n", (err ? "Fail :(" : "OK")); 00225 if (err) { 00226 error("error: %s (%d)\n", strerror(-err), err); 00227 } 00228 } 00229 00230 // Open the numbers file 00231 printf("Opening \"/fs/numbers.txt\"... "); 00232 fflush(stdout); 00233 file = fopen("/fs/numbers.txt", "r+"); 00234 printf("%s\n", (!file ? "Fail :(" : "OK")); 00235 if (!file) { 00236 // Create the numbers file if it doesn't exist 00237 printf("No file found, creating a new file... "); 00238 fflush(stdout); 00239 file = fopen("/fs/numbers.txt", "w+"); 00240 printf("%s\n", (!file ? "Fail :(" : "OK")); 00241 if (!file) { 00242 error("error: %s (%d)\n", strerror(errno), -errno); 00243 } 00244 } 00245 00246 toggle_led.attach(&toggleLed, 0.01); 00247 00248 00249 }
Generated on Fri Jul 15 2022 05:55:10 by
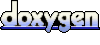