
Test Commit
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "sx12xx.h" 00002 00003 #ifdef TARGET_FF_ARDUINO /* pins of SX126xDVK1xAS board */ 00004 SPI spi(D11, D12, D13); // mosi, miso, sclk 00005 // spi, nss, busy, dio1 00006 SX126x radio(spi, D7, D3, D5 ); 00007 #define CHIP_TYPE_SX1262 0 00008 #define CHIP_TYPE_SX1261 1 00009 DigitalIn chipType(A2); 00010 AnalogIn xtalSel(A3); 00011 DigitalOut antswPower(D8); 00012 #endif /* TARGET_FF_ARDUINO */ 00013 00014 /**********************************************************************/ 00015 volatile bool txDone; 00016 00017 void txDone_callback() 00018 { 00019 txDone = true; 00020 } 00021 00022 int main() 00023 { 00024 uint8_t seq = 0; 00025 00026 printf("\r\nreset-tx "); 00027 00028 radio.setStandby(STBY_XOSC); 00029 radio.setPacketType(PACKET_TYPE_LORA); 00030 radio.setMHz(915.5); 00031 00032 { 00033 ModulationParams_t mp; 00034 00035 mp.lora.spreadingFactor = 11; 00036 mp.lora.bandwidth = LORA_BW_500; 00037 mp.lora.codingRate = LORA_CR_4_5; 00038 mp.lora.LowDatarateOptimize = 0; 00039 00040 radio.xfer(OPCODE_SET_MODULATION_PARAMS, 4,0, mp.buf); 00041 } 00042 00043 if (chipType == CHIP_TYPE_SX1262) 00044 radio.set_tx_dbm(true, 22); 00045 else 00046 radio.set_tx_dbm(false, 14); 00047 00048 00049 { 00050 PacketParams_t p; 00051 00052 p.lora.PreambleLengthHi = 0; 00053 p.lora.PreambleLengthLo = 8; 00054 p.lora.HeaderType = HEADER_TYPE_VARIABLE_LENGTH; 00055 /* constant payload length of one byte */ 00056 p.lora.PayloadLength = 1; 00057 p.lora.CRCType = LORA_CRC_ON; 00058 p.lora.InvertIQ = STANDARD_IQ; 00059 00060 radio.xfer(OPCODE_SET_PACKET_PARAMS, 6,0, p.buf); 00061 } 00062 00063 00064 antswPower = 1; 00065 radio.SetDIO2AsRfSwitchCtrl(1); 00066 00067 radio.txDone = txDone_callback; 00068 00069 for (;;) { 00070 00071 uint8_t buf[2]; 00072 buf[0] = 0; // TX base address 00073 buf[1] = 0; // RX base address 00074 radio.xfer(OPCODE_SET_BUFFER_BASE_ADDR, 2,0, buf); 00075 { 00076 00077 } 00078 00079 radio.tx_buf[0] = seq; /* set payload */ 00080 txDone = false; 00081 radio.start_tx(1); /* begin transmission */ 00082 00083 printf("sent\r\n"); 00084 while (!txDone) { 00085 radio.service(); 00086 } 00087 printf("tx %x\r\n", seq); 00088 printf("got-tx-done\r\n"); 00089 00090 wait(0.5); /* throttle sending rate */ 00091 seq++; /* change payload */ 00092 } 00093 } 00094
Generated on Wed Jul 13 2022 03:32:30 by
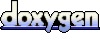