
Blink using Thread
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 DigitalOut led1(LED1); 00004 DigitalOut led2(LED2); 00005 Thread thread; 00006 00007 void led2_thread() { 00008 while (true) { 00009 led2 = !led2; 00010 wait(1); 00011 } 00012 } 00013 00014 int main() { 00015 // Create a thread to execute the function led2_thread 00016 thread.start(led2_thread); 00017 // Now led2_thread is executing concurrently with main at this point 00018 00019 while (true) { 00020 led1 = !led1; 00021 wait(0.5); 00022 } 00023 }
Generated on Sun Jul 17 2022 15:31:33 by
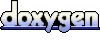