Fork of official USB device library just changed PID (Product ID) in constructor in USBMSD.h to be different for USBMSD_AT45_HelloWorld program
Dependents: USBMSD_AT45_HelloWorld
Fork of USBDevice by
USBAudio.cpp
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "stdint.h" 00020 #include "USBAudio.h" 00021 #include "USBAudio_Types.h" 00022 00023 00024 00025 USBAudio::USBAudio(uint32_t frequency_in, uint8_t channel_nb_in, uint32_t frequency_out, uint8_t channel_nb_out, uint16_t vendor_id, uint16_t product_id, uint16_t product_release): USBDevice(vendor_id, product_id, product_release) { 00026 mute = 0; 00027 volCur = 0x0080; 00028 volMin = 0x0000; 00029 volMax = 0x0100; 00030 volRes = 0x0004; 00031 available = false; 00032 00033 FREQ_IN = frequency_in; 00034 FREQ_OUT = frequency_out; 00035 00036 this->channel_nb_in = channel_nb_in; 00037 this->channel_nb_out = channel_nb_out; 00038 00039 // stereo -> *2, mono -> *1 00040 PACKET_SIZE_ISO_IN = (FREQ_IN / 500) * channel_nb_in; 00041 PACKET_SIZE_ISO_OUT = (FREQ_OUT / 500) * channel_nb_out; 00042 00043 // STEREO -> left and right 00044 channel_config_in = (channel_nb_in == 1) ? CHANNEL_M : CHANNEL_L + CHANNEL_R; 00045 channel_config_out = (channel_nb_out == 1) ? CHANNEL_M : CHANNEL_L + CHANNEL_R; 00046 00047 SOF_handler = false; 00048 00049 buf_stream_out = NULL; 00050 buf_stream_in = NULL; 00051 00052 interruptOUT = false; 00053 writeIN = false; 00054 interruptIN = false; 00055 available = false; 00056 00057 volume = 0; 00058 00059 // connect the device 00060 USBDevice::connect(); 00061 } 00062 00063 bool USBAudio::read(uint8_t * buf) { 00064 buf_stream_in = buf; 00065 SOF_handler = false; 00066 while (!available || !SOF_handler); 00067 available = false; 00068 return true; 00069 } 00070 00071 bool USBAudio::readNB(uint8_t * buf) { 00072 buf_stream_in = buf; 00073 SOF_handler = false; 00074 while (!SOF_handler); 00075 if (available) { 00076 available = false; 00077 buf_stream_in = NULL; 00078 return true; 00079 } 00080 return false; 00081 } 00082 00083 bool USBAudio::readWrite(uint8_t * buf_read, uint8_t * buf_write) { 00084 buf_stream_in = buf_read; 00085 SOF_handler = false; 00086 writeIN = false; 00087 if (interruptIN) { 00088 USBDevice::writeNB(EP3IN, buf_write, PACKET_SIZE_ISO_OUT, PACKET_SIZE_ISO_OUT); 00089 } else { 00090 buf_stream_out = buf_write; 00091 } 00092 while (!available); 00093 if (interruptIN) { 00094 while (!writeIN); 00095 } 00096 while (!SOF_handler); 00097 return true; 00098 } 00099 00100 00101 bool USBAudio::write(uint8_t * buf) { 00102 writeIN = false; 00103 SOF_handler = false; 00104 if (interruptIN) { 00105 USBDevice::writeNB(EP3IN, buf, PACKET_SIZE_ISO_OUT, PACKET_SIZE_ISO_OUT); 00106 } else { 00107 buf_stream_out = buf; 00108 } 00109 while (!SOF_handler); 00110 if (interruptIN) { 00111 while (!writeIN); 00112 } 00113 return true; 00114 } 00115 00116 00117 float USBAudio::getVolume() { 00118 return (mute) ? 0.0 : volume; 00119 } 00120 00121 00122 bool USBAudio::EP3_OUT_callback() { 00123 uint32_t size = 0; 00124 interruptOUT = true; 00125 if (buf_stream_in != NULL) { 00126 readEP(EP3OUT, (uint8_t *)buf_stream_in, &size, PACKET_SIZE_ISO_IN); 00127 available = true; 00128 buf_stream_in = NULL; 00129 } 00130 readStart(EP3OUT, PACKET_SIZE_ISO_IN); 00131 return false; 00132 } 00133 00134 00135 bool USBAudio::EP3_IN_callback() { 00136 interruptIN = true; 00137 writeIN = true; 00138 return true; 00139 } 00140 00141 00142 00143 // Called in ISR context on each start of frame 00144 void USBAudio::SOF(int frameNumber) { 00145 uint32_t size = 0; 00146 00147 if (!interruptOUT) { 00148 // read the isochronous endpoint 00149 if (buf_stream_in != NULL) { 00150 if (USBDevice::readEP_NB(EP3OUT, (uint8_t *)buf_stream_in, &size, PACKET_SIZE_ISO_IN)) { 00151 if (size) { 00152 available = true; 00153 readStart(EP3OUT, PACKET_SIZE_ISO_IN); 00154 buf_stream_in = NULL; 00155 } 00156 } 00157 } 00158 } 00159 00160 if (!interruptIN) { 00161 // write if needed 00162 if (buf_stream_out != NULL) { 00163 USBDevice::writeNB(EP3IN, (uint8_t *)buf_stream_out, PACKET_SIZE_ISO_OUT, PACKET_SIZE_ISO_OUT); 00164 buf_stream_out = NULL; 00165 } 00166 } 00167 00168 SOF_handler = true; 00169 } 00170 00171 00172 // Called in ISR context 00173 // Set configuration. Return false if the configuration is not supported. 00174 bool USBAudio::USBCallback_setConfiguration(uint8_t configuration) { 00175 if (configuration != DEFAULT_CONFIGURATION) { 00176 return false; 00177 } 00178 00179 // Configure isochronous endpoint 00180 realiseEndpoint(EP3OUT, PACKET_SIZE_ISO_IN, ISOCHRONOUS); 00181 realiseEndpoint(EP3IN, PACKET_SIZE_ISO_OUT, ISOCHRONOUS); 00182 00183 // activate readings on this endpoint 00184 readStart(EP3OUT, PACKET_SIZE_ISO_IN); 00185 return true; 00186 } 00187 00188 00189 // Called in ISR context 00190 // Set alternate setting. Return false if the alternate setting is not supported 00191 bool USBAudio::USBCallback_setInterface(uint16_t interface, uint8_t alternate) { 00192 if (interface == 0 && alternate == 0) { 00193 return true; 00194 } 00195 if (interface == 1 && (alternate == 0 || alternate == 1)) { 00196 return true; 00197 } 00198 if (interface == 2 && (alternate == 0 || alternate == 1)) { 00199 return true; 00200 } 00201 return false; 00202 } 00203 00204 00205 00206 // Called in ISR context 00207 // Called by USBDevice on Endpoint0 request 00208 // This is used to handle extensions to standard requests and class specific requests. 00209 // Return true if class handles this request 00210 bool USBAudio::USBCallback_request() { 00211 bool success = false; 00212 CONTROL_TRANSFER * transfer = getTransferPtr(); 00213 00214 // Process class-specific requests 00215 if (transfer->setup.bmRequestType.Type == CLASS_TYPE) { 00216 00217 // Feature Unit: Interface = 0, ID = 2 00218 if (transfer->setup.wIndex == 0x0200) { 00219 00220 // Master Channel 00221 if ((transfer->setup.wValue & 0xff) == 0) { 00222 00223 switch (transfer->setup.wValue >> 8) { 00224 case MUTE_CONTROL: 00225 switch (transfer->setup.bRequest) { 00226 case REQUEST_GET_CUR: 00227 transfer->remaining = 1; 00228 transfer->ptr = &mute; 00229 transfer->direction = DEVICE_TO_HOST; 00230 success = true; 00231 break; 00232 00233 case REQUEST_SET_CUR: 00234 transfer->remaining = 1; 00235 transfer->notify = true; 00236 transfer->direction = HOST_TO_DEVICE; 00237 success = true; 00238 break; 00239 default: 00240 break; 00241 } 00242 break; 00243 case VOLUME_CONTROL: 00244 switch (transfer->setup.bRequest) { 00245 case REQUEST_GET_CUR: 00246 transfer->remaining = 2; 00247 transfer->ptr = (uint8_t *)&volCur; 00248 transfer->direction = DEVICE_TO_HOST; 00249 success = true; 00250 break; 00251 case REQUEST_GET_MIN: 00252 transfer->remaining = 2; 00253 transfer->ptr = (uint8_t *)&volMin; 00254 transfer->direction = DEVICE_TO_HOST; 00255 success = true; 00256 break; 00257 case REQUEST_GET_MAX: 00258 transfer->remaining = 2; 00259 transfer->ptr = (uint8_t *)&volMax; 00260 transfer->direction = DEVICE_TO_HOST; 00261 success = true; 00262 break; 00263 case REQUEST_GET_RES: 00264 transfer->remaining = 2; 00265 transfer->ptr = (uint8_t *)&volRes; 00266 transfer->direction = DEVICE_TO_HOST; 00267 success = true; 00268 break; 00269 00270 case REQUEST_SET_CUR: 00271 transfer->remaining = 2; 00272 transfer->notify = true; 00273 transfer->direction = HOST_TO_DEVICE; 00274 success = true; 00275 break; 00276 case REQUEST_SET_MIN: 00277 transfer->remaining = 2; 00278 transfer->notify = true; 00279 transfer->direction = HOST_TO_DEVICE; 00280 success = true; 00281 break; 00282 case REQUEST_SET_MAX: 00283 transfer->remaining = 2; 00284 transfer->notify = true; 00285 transfer->direction = HOST_TO_DEVICE; 00286 success = true; 00287 break; 00288 case REQUEST_SET_RES: 00289 transfer->remaining = 2; 00290 transfer->notify = true; 00291 transfer->direction = HOST_TO_DEVICE; 00292 success = true; 00293 break; 00294 } 00295 break; 00296 default: 00297 break; 00298 } 00299 } 00300 } 00301 } 00302 return success; 00303 } 00304 00305 00306 // Called in ISR context when a data OUT stage has been performed 00307 void USBAudio::USBCallback_requestCompleted(uint8_t * buf, uint32_t length) { 00308 if ((length == 1) || (length == 2)) { 00309 uint16_t data = (length == 1) ? *buf : *((uint16_t *)buf); 00310 CONTROL_TRANSFER * transfer = getTransferPtr(); 00311 switch (transfer->setup.wValue >> 8) { 00312 case MUTE_CONTROL: 00313 switch (transfer->setup.bRequest) { 00314 case REQUEST_SET_CUR: 00315 mute = data & 0xff; 00316 updateVol.call(); 00317 break; 00318 default: 00319 break; 00320 } 00321 break; 00322 case VOLUME_CONTROL: 00323 switch (transfer->setup.bRequest) { 00324 case REQUEST_SET_CUR: 00325 volCur = data; 00326 volume = (float)volCur/(float)volMax; 00327 updateVol.call(); 00328 break; 00329 default: 00330 break; 00331 } 00332 break; 00333 default: 00334 break; 00335 } 00336 } 00337 } 00338 00339 00340 00341 #define TOTAL_DESCRIPTOR_LENGTH ((1 * CONFIGURATION_DESCRIPTOR_LENGTH) \ 00342 + (5 * INTERFACE_DESCRIPTOR_LENGTH) \ 00343 + (1 * CONTROL_INTERFACE_DESCRIPTOR_LENGTH + 1) \ 00344 + (2 * INPUT_TERMINAL_DESCRIPTOR_LENGTH) \ 00345 + (1 * FEATURE_UNIT_DESCRIPTOR_LENGTH) \ 00346 + (2 * OUTPUT_TERMINAL_DESCRIPTOR_LENGTH) \ 00347 + (2 * STREAMING_INTERFACE_DESCRIPTOR_LENGTH) \ 00348 + (2 * FORMAT_TYPE_I_DESCRIPTOR_LENGTH) \ 00349 + (2 * (ENDPOINT_DESCRIPTOR_LENGTH + 2)) \ 00350 + (2 * STREAMING_ENDPOINT_DESCRIPTOR_LENGTH) ) 00351 00352 #define TOTAL_CONTROL_INTF_LENGTH (CONTROL_INTERFACE_DESCRIPTOR_LENGTH + 1 + \ 00353 2*INPUT_TERMINAL_DESCRIPTOR_LENGTH + \ 00354 FEATURE_UNIT_DESCRIPTOR_LENGTH + \ 00355 2*OUTPUT_TERMINAL_DESCRIPTOR_LENGTH) 00356 00357 uint8_t * USBAudio::configurationDesc() { 00358 static uint8_t configDescriptor[] = { 00359 // Configuration 1 00360 CONFIGURATION_DESCRIPTOR_LENGTH, // bLength 00361 CONFIGURATION_DESCRIPTOR, // bDescriptorType 00362 LSB(TOTAL_DESCRIPTOR_LENGTH), // wTotalLength (LSB) 00363 MSB(TOTAL_DESCRIPTOR_LENGTH), // wTotalLength (MSB) 00364 0x03, // bNumInterfaces 00365 DEFAULT_CONFIGURATION, // bConfigurationValue 00366 0x00, // iConfiguration 00367 0x80, // bmAttributes 00368 50, // bMaxPower 00369 00370 // Interface 0, Alternate Setting 0, Audio Control 00371 INTERFACE_DESCRIPTOR_LENGTH, // bLength 00372 INTERFACE_DESCRIPTOR, // bDescriptorType 00373 0x00, // bInterfaceNumber 00374 0x00, // bAlternateSetting 00375 0x00, // bNumEndpoints 00376 AUDIO_CLASS, // bInterfaceClass 00377 SUBCLASS_AUDIOCONTROL, // bInterfaceSubClass 00378 0x00, // bInterfaceProtocol 00379 0x00, // iInterface 00380 00381 00382 // Audio Control Interface 00383 CONTROL_INTERFACE_DESCRIPTOR_LENGTH + 1,// bLength 00384 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00385 CONTROL_HEADER, // bDescriptorSubtype 00386 LSB(0x0100), // bcdADC (LSB) 00387 MSB(0x0100), // bcdADC (MSB) 00388 LSB(TOTAL_CONTROL_INTF_LENGTH), // wTotalLength 00389 MSB(TOTAL_CONTROL_INTF_LENGTH), // wTotalLength 00390 0x02, // bInCollection 00391 0x01, // baInterfaceNr 00392 0x02, // baInterfaceNr 00393 00394 // Audio Input Terminal (Speaker) 00395 INPUT_TERMINAL_DESCRIPTOR_LENGTH, // bLength 00396 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00397 CONTROL_INPUT_TERMINAL, // bDescriptorSubtype 00398 0x01, // bTerminalID 00399 LSB(TERMINAL_USB_STREAMING), // wTerminalType 00400 MSB(TERMINAL_USB_STREAMING), // wTerminalType 00401 0x00, // bAssocTerminal 00402 channel_nb_in, // bNrChannels 00403 LSB(channel_config_in), // wChannelConfig 00404 MSB(channel_config_in), // wChannelConfig 00405 0x00, // iChannelNames 00406 0x00, // iTerminal 00407 00408 // Audio Feature Unit (Speaker) 00409 FEATURE_UNIT_DESCRIPTOR_LENGTH, // bLength 00410 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00411 CONTROL_FEATURE_UNIT, // bDescriptorSubtype 00412 0x02, // bUnitID 00413 0x01, // bSourceID 00414 0x01, // bControlSize 00415 CONTROL_MUTE | 00416 CONTROL_VOLUME, // bmaControls(0) 00417 0x00, // bmaControls(1) 00418 0x00, // iTerminal 00419 00420 // Audio Output Terminal (Speaker) 00421 OUTPUT_TERMINAL_DESCRIPTOR_LENGTH, // bLength 00422 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00423 CONTROL_OUTPUT_TERMINAL, // bDescriptorSubtype 00424 0x03, // bTerminalID 00425 LSB(TERMINAL_SPEAKER), // wTerminalType 00426 MSB(TERMINAL_SPEAKER), // wTerminalType 00427 0x00, // bAssocTerminal 00428 0x02, // bSourceID 00429 0x00, // iTerminal 00430 00431 00432 // Audio Input Terminal (Microphone) 00433 INPUT_TERMINAL_DESCRIPTOR_LENGTH, // bLength 00434 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00435 CONTROL_INPUT_TERMINAL, // bDescriptorSubtype 00436 0x04, // bTerminalID 00437 LSB(TERMINAL_MICROPHONE), // wTerminalType 00438 MSB(TERMINAL_MICROPHONE), // wTerminalType 00439 0x00, // bAssocTerminal 00440 channel_nb_out, // bNrChannels 00441 LSB(channel_config_out), // wChannelConfig 00442 MSB(channel_config_out), // wChannelConfig 00443 0x00, // iChannelNames 00444 0x00, // iTerminal 00445 00446 // Audio Output Terminal (Microphone) 00447 OUTPUT_TERMINAL_DESCRIPTOR_LENGTH, // bLength 00448 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00449 CONTROL_OUTPUT_TERMINAL, // bDescriptorSubtype 00450 0x05, // bTerminalID 00451 LSB(TERMINAL_USB_STREAMING), // wTerminalType 00452 MSB(TERMINAL_USB_STREAMING), // wTerminalType 00453 0x00, // bAssocTerminal 00454 0x04, // bSourceID 00455 0x00, // iTerminal 00456 00457 00458 00459 00460 00461 00462 // Interface 1, Alternate Setting 0, Audio Streaming - Zero Bandwith 00463 INTERFACE_DESCRIPTOR_LENGTH, // bLength 00464 INTERFACE_DESCRIPTOR, // bDescriptorType 00465 0x01, // bInterfaceNumber 00466 0x00, // bAlternateSetting 00467 0x00, // bNumEndpoints 00468 AUDIO_CLASS, // bInterfaceClass 00469 SUBCLASS_AUDIOSTREAMING, // bInterfaceSubClass 00470 0x00, // bInterfaceProtocol 00471 0x00, // iInterface 00472 00473 // Interface 1, Alternate Setting 1, Audio Streaming - Operational 00474 INTERFACE_DESCRIPTOR_LENGTH, // bLength 00475 INTERFACE_DESCRIPTOR, // bDescriptorType 00476 0x01, // bInterfaceNumber 00477 0x01, // bAlternateSetting 00478 0x01, // bNumEndpoints 00479 AUDIO_CLASS, // bInterfaceClass 00480 SUBCLASS_AUDIOSTREAMING, // bInterfaceSubClass 00481 0x00, // bInterfaceProtocol 00482 0x00, // iInterface 00483 00484 // Audio Streaming Interface 00485 STREAMING_INTERFACE_DESCRIPTOR_LENGTH, // bLength 00486 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00487 STREAMING_GENERAL, // bDescriptorSubtype 00488 0x01, // bTerminalLink 00489 0x00, // bDelay 00490 LSB(FORMAT_PCM), // wFormatTag 00491 MSB(FORMAT_PCM), // wFormatTag 00492 00493 // Audio Type I Format 00494 FORMAT_TYPE_I_DESCRIPTOR_LENGTH, // bLength 00495 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00496 STREAMING_FORMAT_TYPE, // bDescriptorSubtype 00497 FORMAT_TYPE_I, // bFormatType 00498 channel_nb_in, // bNrChannels 00499 0x02, // bSubFrameSize 00500 16, // bBitResolution 00501 0x01, // bSamFreqType 00502 LSB(FREQ_IN), // tSamFreq 00503 (FREQ_IN >> 8) & 0xff, // tSamFreq 00504 (FREQ_IN >> 16) & 0xff, // tSamFreq 00505 00506 // Endpoint - Standard Descriptor 00507 ENDPOINT_DESCRIPTOR_LENGTH + 2, // bLength 00508 ENDPOINT_DESCRIPTOR, // bDescriptorType 00509 PHY_TO_DESC(EPISO_OUT), // bEndpointAddress 00510 E_ISOCHRONOUS, // bmAttributes 00511 LSB(PACKET_SIZE_ISO_IN), // wMaxPacketSize 00512 MSB(PACKET_SIZE_ISO_IN), // wMaxPacketSize 00513 0x01, // bInterval 00514 0x00, // bRefresh 00515 0x00, // bSynchAddress 00516 00517 // Endpoint - Audio Streaming 00518 STREAMING_ENDPOINT_DESCRIPTOR_LENGTH, // bLength 00519 ENDPOINT_DESCRIPTOR_TYPE, // bDescriptorType 00520 ENDPOINT_GENERAL, // bDescriptor 00521 0x00, // bmAttributes 00522 0x00, // bLockDelayUnits 00523 LSB(0x0000), // wLockDelay 00524 MSB(0x0000), // wLockDelay 00525 00526 00527 00528 00529 00530 00531 00532 // Interface 1, Alternate Setting 0, Audio Streaming - Zero Bandwith 00533 INTERFACE_DESCRIPTOR_LENGTH, // bLength 00534 INTERFACE_DESCRIPTOR, // bDescriptorType 00535 0x02, // bInterfaceNumber 00536 0x00, // bAlternateSetting 00537 0x00, // bNumEndpoints 00538 AUDIO_CLASS, // bInterfaceClass 00539 SUBCLASS_AUDIOSTREAMING, // bInterfaceSubClass 00540 0x00, // bInterfaceProtocol 00541 0x00, // iInterface 00542 00543 // Interface 1, Alternate Setting 1, Audio Streaming - Operational 00544 INTERFACE_DESCRIPTOR_LENGTH, // bLength 00545 INTERFACE_DESCRIPTOR, // bDescriptorType 00546 0x02, // bInterfaceNumber 00547 0x01, // bAlternateSetting 00548 0x01, // bNumEndpoints 00549 AUDIO_CLASS, // bInterfaceClass 00550 SUBCLASS_AUDIOSTREAMING, // bInterfaceSubClass 00551 0x00, // bInterfaceProtocol 00552 0x00, // iInterface 00553 00554 // Audio Streaming Interface 00555 STREAMING_INTERFACE_DESCRIPTOR_LENGTH, // bLength 00556 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00557 SUBCLASS_AUDIOCONTROL, // bDescriptorSubtype 00558 0x05, // bTerminalLink (output terminal microphone) 00559 0x01, // bDelay 00560 0x01, // wFormatTag 00561 0x00, // wFormatTag 00562 00563 // Audio Type I Format 00564 FORMAT_TYPE_I_DESCRIPTOR_LENGTH, // bLength 00565 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00566 SUBCLASS_AUDIOSTREAMING, // bDescriptorSubtype 00567 FORMAT_TYPE_I, // bFormatType 00568 channel_nb_out, // bNrChannels 00569 0x02, // bSubFrameSize 00570 0x10, // bBitResolution 00571 0x01, // bSamFreqType 00572 LSB(FREQ_OUT), // tSamFreq 00573 (FREQ_OUT >> 8) & 0xff, // tSamFreq 00574 (FREQ_OUT >> 16) & 0xff, // tSamFreq 00575 00576 // Endpoint - Standard Descriptor 00577 ENDPOINT_DESCRIPTOR_LENGTH + 2, // bLength 00578 ENDPOINT_DESCRIPTOR, // bDescriptorType 00579 PHY_TO_DESC(EPISO_IN), // bEndpointAddress 00580 E_ISOCHRONOUS, // bmAttributes 00581 LSB(PACKET_SIZE_ISO_OUT), // wMaxPacketSize 00582 MSB(PACKET_SIZE_ISO_OUT), // wMaxPacketSize 00583 0x01, // bInterval 00584 0x00, // bRefresh 00585 0x00, // bSynchAddress 00586 00587 // Endpoint - Audio Streaming 00588 STREAMING_ENDPOINT_DESCRIPTOR_LENGTH, // bLength 00589 ENDPOINT_DESCRIPTOR_TYPE, // bDescriptorType 00590 ENDPOINT_GENERAL, // bDescriptor 00591 0x00, // bmAttributes 00592 0x00, // bLockDelayUnits 00593 LSB(0x0000), // wLockDelay 00594 MSB(0x0000), // wLockDelay 00595 00596 // Terminator 00597 0 // bLength 00598 }; 00599 return configDescriptor; 00600 } 00601 00602 uint8_t * USBAudio::stringIinterfaceDesc() { 00603 static uint8_t stringIinterfaceDescriptor[] = { 00604 0x0c, //bLength 00605 STRING_DESCRIPTOR, //bDescriptorType 0x03 00606 'A',0,'u',0,'d',0,'i',0,'o',0 //bString iInterface - Audio 00607 }; 00608 return stringIinterfaceDescriptor; 00609 } 00610 00611 uint8_t * USBAudio::stringIproductDesc() { 00612 static uint8_t stringIproductDescriptor[] = { 00613 0x16, //bLength 00614 STRING_DESCRIPTOR, //bDescriptorType 0x03 00615 'M',0,'b',0,'e',0,'d',0,' ',0,'A',0,'u',0,'d',0,'i',0,'o',0 //bString iProduct - Mbed Audio 00616 }; 00617 return stringIproductDescriptor; 00618 }
Generated on Tue Jul 12 2022 21:32:43 by
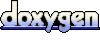