Player Library
Embed:
(wiki syntax)
Show/hide line numbers
Player.h
00001 #ifndef PLAYER_H 00002 #define PLAYER_H 00003 00004 #include "mbed.h" 00005 #include "N5110.h" 00006 #include "Gamepad.h" 00007 00008 00009 class Player 00010 { 00011 public: 00012 00013 00014 Player(); 00015 ~Player(); 00016 00017 /** Initialise Player 00018 * 00019 * This function initalises the player library. 00020 */ 00021 void init(); 00022 00023 /** Draw 00024 * 00025 * This function draws the Player sprite onto the screen at the specified coordinates. 00026 */ 00027 void draw(N5110 &lcd); 00028 00029 /** Update 00030 * 00031 * This function updates the player sprite position on screen. 00032 */ 00033 void update(Direction d,float mag); 00034 00035 /** Get Position 00036 * 00037 * This function obtains the coordinate of the top-left pixel in the player sprite. 00038 */ 00039 Vector2D get_pos(); 00040 00041 00042 private: 00043 int m; 00044 int _x; 00045 int _y; 00046 int _speed; 00047 00048 }; 00049 #endif
Generated on Sat Jul 16 2022 23:20:35 by
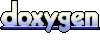