Player Library
Embed:
(wiki syntax)
Show/hide line numbers
Player.cpp
00001 #include "Player.h" 00002 00003 Player::Player() 00004 { 00005 00006 } 00007 00008 Player::~Player() 00009 { 00010 00011 } 00012 00013 //The Player Sprite. 00014 int sprite[5][6] = { 00015 {0,1,0,0,1,0}, 00016 {1,1,0,0,1,1}, 00017 {1,1,1,1,1,1}, 00018 {1,1,1,1,1,1}, 00019 {0,1,1,1,1,0}, 00020 }; 00021 00022 int m = 0; //Variable used to allow a starting location for the player. 00023 00024 00025 void Player::init() 00026 { 00027 00028 _speed = 0.15;// default speed 00029 00030 } 00031 00032 00033 void Player::draw(N5110 &lcd) 00034 { 00035 if(m == 0){ 00036 _x = WIDTH/2 -3; //Spawns player sprite near the middle of the screen. 00037 _y = HEIGHT/2 +5; 00038 m = m+1; 00039 } 00040 //printf("SPRITE %d %d \n", _x, _y); 00041 lcd.drawSprite(_x,_y,5,6,(int *)sprite); //Function used to draw the sprite. 00042 } 00043 00044 00045 00046 Vector2D Player::get_pos() 00047 { 00048 Vector2D playerpos = {_x,_y}; //Obtains the player position. 00049 //printf("playerpos from player = %f %f \n", playerpos.x, playerpos.y); 00050 return playerpos; 00051 } 00052 00053 00054 00055 00056 00057 00058 00059 void Player::update(Direction d,float mag) 00060 { 00061 _speed = int(mag*7.0f); //Speed changes depending on how much you push the joystick. 00062 00063 00064 // North is decrement as origin is at the top-left so decreasing moves up 00065 // Diagonal speeds are /2 to prevent player from going double the speed. 00066 if (d == N) { 00067 _y-=_speed; 00068 } 00069 if (d == S) { 00070 _y+=_speed; 00071 } 00072 if (d == E) { 00073 _x+=_speed; 00074 } 00075 if (d == W) { 00076 _x-=_speed; 00077 } 00078 if (d == NE) { 00079 _y-=_speed/2; 00080 _x+=_speed/2; 00081 } 00082 if (d == NW) { 00083 _y-=_speed/2; 00084 _x-=_speed/2; 00085 } 00086 if (d == SE) { 00087 _y+=_speed/2; 00088 _x+=_speed/2; 00089 } 00090 if (d == SW) { 00091 _y+=_speed/2; 00092 _x-=_speed/2; 00093 } 00094 00095 00096 //Limits set so that the sprite does not travel off the screen. 00097 if (_y <= 0) { 00098 _y = 0; 00099 } 00100 if (_x <= 0) { 00101 _x = 0; 00102 } 00103 if (_x > 78) { 00104 _x = 78; 00105 } 00106 if (_y > 42) { 00107 _y = 42; 00108 } 00109 00110 } 00111
Generated on Sat Jul 16 2022 23:20:35 by
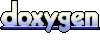