
work fine
Embed:
(wiki syntax)
Show/hide line numbers
WString.h
00001 /* 00002 WString.h - String library for Wiring & Arduino 00003 ...mostly rewritten by Paul Stoffregen... 00004 Copyright (c) 2009-10 Hernando Barragan. All right reserved. 00005 Copyright 2011, Paul Stoffregen, paul@pjrc.com 00006 00007 This library is free software; you can redistribute it and/or 00008 modify it under the terms of the GNU Lesser General Public 00009 License as published by the Free Software Foundation; either 00010 version 2.1 of the License, or (at your option) any later version. 00011 00012 This library is distributed in the hope that it will be useful, 00013 but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00015 Lesser General Public License for more details. 00016 00017 You should have received a copy of the GNU Lesser General Public 00018 License along with this library; if not, write to the Free Software 00019 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00020 */ 00021 00022 #ifndef String_class_h 00023 #define String_class_h 00024 #ifdef __cplusplus 00025 00026 #include <stdlib.h> 00027 #include <string.h> 00028 #include <ctype.h> 00029 //#include <avr/pgmspace.h> 00030 00031 // When compiling programs with this class, the following gcc parameters 00032 // dramatically increase performance and memory (RAM) efficiency, typically 00033 // with little or no increase in code size. 00034 // -felide-constructors 00035 // -std=c++0x 00036 00037 //class __FlashStringHelper; 00038 #define F(string_literal) (reinterpret_cast<const __FlashStringHelper *>(PSTR(string_literal))) 00039 00040 // An inherited class for holding the result of a concatenation. These 00041 // result objects are assumed to be writable by subsequent concatenations. 00042 class StringSumHelper; 00043 00044 // The string class 00045 class String 00046 { 00047 // use a function pointer to allow for "if (s)" without the 00048 // complications of an operator bool(). for more information, see: 00049 // http://www.artima.com/cppsource/safebool.html 00050 typedef void (String::*StringIfHelperType)() const; 00051 void StringIfHelper() const {} 00052 00053 public: 00054 // constructors 00055 // creates a copy of the initial value. 00056 // if the initial value is null or invalid, or if memory allocation 00057 // fails, the string will be marked as invalid (i.e. "if (s)" will 00058 // be false). 00059 String(const char *cstr = ""); 00060 String(const String &str); 00061 #if 0 00062 String(const __FlashStringHelper *str); 00063 #endif 00064 #if __cplusplus >= 201103L || defined(__GXX_EXPERIMENTAL_CXX0X__) 00065 String(String &&rval); 00066 String(StringSumHelper &&rval); 00067 #endif 00068 explicit String(char c); 00069 explicit String(unsigned char, unsigned char base=10); 00070 explicit String(int, unsigned char base=10); 00071 explicit String(unsigned int, unsigned char base=10); 00072 explicit String(long, unsigned char base=10); 00073 explicit String(unsigned long, unsigned char base=10); 00074 explicit String(float, unsigned char decimalPlaces=2); 00075 explicit String(double, unsigned char decimalPlaces=2); 00076 ~String(void); 00077 00078 // memory management 00079 // return true on success, false on failure (in which case, the string 00080 // is left unchanged). reserve(0), if successful, will validate an 00081 // invalid string (i.e., "if (s)" will be true afterwards) 00082 unsigned char reserve(unsigned int size); 00083 inline unsigned int length(void) const { 00084 return len; 00085 } 00086 00087 // creates a copy of the assigned value. if the value is null or 00088 // invalid, or if the memory allocation fails, the string will be 00089 // marked as invalid ("if (s)" will be false). 00090 String & operator = (const String &rhs); 00091 String & operator = (const char *cstr); 00092 #if 0 00093 String & operator = (const __FlashStringHelper *str); 00094 #endif 00095 #if __cplusplus >= 201103L || defined(__GXX_EXPERIMENTAL_CXX0X__) 00096 String & operator = (String &&rval); 00097 String & operator = (StringSumHelper &&rval); 00098 #endif 00099 00100 // concatenate (works w/ built-in types) 00101 00102 // returns true on success, false on failure (in which case, the string 00103 // is left unchanged). if the argument is null or invalid, the 00104 // concatenation is considered unsucessful. 00105 unsigned char concat(const String &str); 00106 unsigned char concat(const char *cstr); 00107 unsigned char concat(char c); 00108 unsigned char concat(unsigned char c); 00109 unsigned char concat(int num); 00110 unsigned char concat(unsigned int num); 00111 unsigned char concat(long num); 00112 unsigned char concat(unsigned long num); 00113 unsigned char concat(float num); 00114 unsigned char concat(double num); 00115 #if 0 00116 unsigned char concat(const __FlashStringHelper * str); 00117 #endif 00118 // if there's not enough memory for the concatenated value, the string 00119 // will be left unchanged (but this isn't signalled in any way) 00120 String & operator += (const String &rhs) { 00121 concat(rhs); 00122 return (*this); 00123 } 00124 String & operator += (const char *cstr) { 00125 concat(cstr); 00126 return (*this); 00127 } 00128 String & operator += (char c) { 00129 concat(c); 00130 return (*this); 00131 } 00132 String & operator += (unsigned char num) { 00133 concat(num); 00134 return (*this); 00135 } 00136 String & operator += (int num) { 00137 concat(num); 00138 return (*this); 00139 } 00140 String & operator += (unsigned int num) { 00141 concat(num); 00142 return (*this); 00143 } 00144 String & operator += (long num) { 00145 concat(num); 00146 return (*this); 00147 } 00148 String & operator += (unsigned long num) { 00149 concat(num); 00150 return (*this); 00151 } 00152 String & operator += (float num) { 00153 concat(num); 00154 return (*this); 00155 } 00156 String & operator += (double num) { 00157 concat(num); 00158 return (*this); 00159 } 00160 #if 0 00161 String & operator += (const __FlashStringHelper *str) { 00162 concat(str); 00163 return (*this); 00164 } 00165 #endif 00166 00167 friend StringSumHelper & operator + (const StringSumHelper &lhs, const String &rhs); 00168 friend StringSumHelper & operator + (const StringSumHelper &lhs, const char *cstr); 00169 friend StringSumHelper & operator + (const StringSumHelper &lhs, char c); 00170 friend StringSumHelper & operator + (const StringSumHelper &lhs, unsigned char num); 00171 friend StringSumHelper & operator + (const StringSumHelper &lhs, int num); 00172 friend StringSumHelper & operator + (const StringSumHelper &lhs, unsigned int num); 00173 friend StringSumHelper & operator + (const StringSumHelper &lhs, long num); 00174 friend StringSumHelper & operator + (const StringSumHelper &lhs, unsigned long num); 00175 friend StringSumHelper & operator + (const StringSumHelper &lhs, float num); 00176 friend StringSumHelper & operator + (const StringSumHelper &lhs, double num); 00177 #if 0 00178 friend StringSumHelper & operator + (const StringSumHelper &lhs, const __FlashStringHelper *rhs); 00179 #endif 00180 // comparison (only works w/ Strings and "strings") 00181 operator StringIfHelperType() const { 00182 return buffer ? &String::StringIfHelper : 0; 00183 } 00184 int compareTo(const String &s) const; 00185 unsigned char equals(const String &s) const; 00186 unsigned char equals(const char *cstr) const; 00187 unsigned char operator == (const String &rhs) const { 00188 return equals(rhs); 00189 } 00190 unsigned char operator == (const char *cstr) const { 00191 return equals(cstr); 00192 } 00193 unsigned char operator != (const String &rhs) const { 00194 return !equals(rhs); 00195 } 00196 unsigned char operator != (const char *cstr) const { 00197 return !equals(cstr); 00198 } 00199 unsigned char operator < (const String &rhs) const; 00200 unsigned char operator > (const String &rhs) const; 00201 unsigned char operator <= (const String &rhs) const; 00202 unsigned char operator >= (const String &rhs) const; 00203 unsigned char equalsIgnoreCase(const String &s) const; 00204 unsigned char startsWith( const String &prefix) const; 00205 unsigned char startsWith(const String &prefix, unsigned int offset) const; 00206 unsigned char endsWith(const String &suffix) const; 00207 00208 // character acccess 00209 char charAt(unsigned int index) const; 00210 void setCharAt(unsigned int index, char c); 00211 char operator [] (unsigned int index) const; 00212 char& operator [] (unsigned int index); 00213 void getBytes(unsigned char *buf, unsigned int bufsize, unsigned int index=0) const; 00214 void toCharArray(char *buf, unsigned int bufsize, unsigned int index=0) const { 00215 getBytes((unsigned char *)buf, bufsize, index); 00216 } 00217 const char * c_str() const { 00218 return buffer; 00219 } 00220 const char* begin() { 00221 return c_str(); 00222 } 00223 const char* end() { 00224 return c_str() + length(); 00225 } 00226 00227 // search 00228 int indexOf( char ch ) const; 00229 int indexOf( char ch, unsigned int fromIndex ) const; 00230 int indexOf( const String &str ) const; 00231 int indexOf( const String &str, unsigned int fromIndex ) const; 00232 int lastIndexOf( char ch ) const; 00233 int lastIndexOf( char ch, unsigned int fromIndex ) const; 00234 int lastIndexOf( const String &str ) const; 00235 int lastIndexOf( const String &str, unsigned int fromIndex ) const; 00236 String substring( unsigned int beginIndex ) const { 00237 return substring(beginIndex, len); 00238 }; 00239 String substring( unsigned int beginIndex, unsigned int endIndex ) const; 00240 00241 // modification 00242 void replace(char find, char replace); 00243 void replace(const String& find, const String& replace); 00244 void remove(unsigned int index); 00245 void remove(unsigned int index, unsigned int count); 00246 void toLowerCase(void); 00247 void toUpperCase(void); 00248 void trim(void); 00249 00250 // parsing/conversion 00251 long toInt(void) const; 00252 float toFloat(void) const; 00253 00254 protected: 00255 char *buffer; // the actual char array 00256 unsigned int capacity; // the array length minus one (for the '\0') 00257 unsigned int len; // the String length (not counting the '\0') 00258 protected: 00259 void init(void); 00260 void invalidate(void); 00261 unsigned char changeBuffer(unsigned int maxStrLen); 00262 unsigned char concat(const char *cstr, unsigned int length); 00263 00264 // copy and move 00265 String & copy(const char *cstr, unsigned int length); 00266 #if 0 00267 String & copy(const __FlashStringHelper *pstr, unsigned int length); 00268 #endif 00269 #if __cplusplus >= 201103L || defined(__GXX_EXPERIMENTAL_CXX0X__) 00270 void move(String &rhs); 00271 #endif 00272 }; 00273 00274 class StringSumHelper : public String 00275 { 00276 public: 00277 StringSumHelper(const String &s) : String(s) {} 00278 StringSumHelper(const char *p) : String(p) {} 00279 StringSumHelper(char c) : String(c) {} 00280 StringSumHelper(unsigned char num) : String(num) {} 00281 StringSumHelper(int num) : String(num) {} 00282 StringSumHelper(unsigned int num) : String(num) {} 00283 StringSumHelper(long num) : String(num) {} 00284 StringSumHelper(unsigned long num) : String(num) {} 00285 StringSumHelper(float num) : String(num) {} 00286 StringSumHelper(double num) : String(num) {} 00287 }; 00288 00289 #endif // __cplusplus 00290 #endif // String_class_h 00291
Generated on Thu Jul 14 2022 22:36:21 by
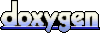