
work fine
Embed:
(wiki syntax)
Show/hide line numbers
Microduino_Matrix.h
00001 // 本作品采用知识共享 署名-非商业性使用-相同方式共享 3.0 未本地化版本 许可协议进行许可 00002 // 访问 http://creativecommons.org/licenses/by-nc-sa/3.0/ 查看该许可协议 00003 // ============== 00004 00005 // 版权所有: 00006 // @老潘orz wasdpkj@hotmail.com 00007 // ============== 00008 00009 // Microduino-IDE 00010 // ============== 00011 // Microduino Getting start: 00012 // http://www.microduino.cc/download/ 00013 00014 // Microduino IDE Support: 00015 // https://github.com/wasdpkj/Microduino-IDE-Support/ 00016 00017 // ============== 00018 // Microduino wiki: 00019 // http://wiki.microduino.cc 00020 00021 // ============== 00022 // E-mail: 00023 // Kejia Pan 00024 // pankejia@microduino.cc 00025 00026 // ============== 00027 // Weibo: 00028 // @老潘orz 00029 00030 #ifndef Microduino_Matrix_h 00031 #define Microduino_Matrix_h 00032 #include "Printit.h" 00033 #include "mbed.h" 00034 00035 #if 0 00036 #if (ARDUINO >= 100) 00037 #include "Arduino.h" 00038 #include "Print.h" 00039 #else 00040 #include "WProgram.h" 00041 #endif 00042 #endif 00043 #include "Microduino_MatrixBase.h" 00044 00045 #define WDT 00046 #ifdef WDT 00047 //#include <avr/wdt.h> 00048 #endif 00049 00050 #define MODE_H 1 00051 #define MODE_V 0 00052 00053 #define U8G_DRAW_UPPER_RIGHT 0x01 00054 #define U8G_DRAW_UPPER_LEFT 0x02 00055 #define U8G_DRAW_LOWER_LEFT 0x04 00056 #define U8G_DRAW_LOWER_RIGHT 0x08 00057 #define U8G_DRAW_ALL (U8G_DRAW_UPPER_RIGHT|U8G_DRAW_UPPER_LEFT|U8G_DRAW_LOWER_RIGHT|U8G_DRAW_LOWER_LEFT) 00058 00059 #define min(x, y) ({ \ 00060 typeof(x) _min1 = (x); \ 00061 typeof(y) _min2 = (y); \ 00062 (void) (&_min1 == &_min2); \ 00063 _min1 < _min2 ? _min1 : _min2; }) 00064 00065 #define max(x, y) ({ \ 00066 typeof(x) _max1 = (x); \ 00067 typeof(y) _max2 = (y); \ 00068 (void) (&_max1 == &_max2); \ 00069 _max1 > _max2 ? _max1 : _max2; }) 00070 00071 class Matrix : public Print 00072 { 00073 public: 00074 LedControl* led; 00075 00076 Matrix(uint8_t (*_addr)[8]); 00077 00078 int16_t getWidth(); 00079 int16_t getHeight(); 00080 int16_t getMatrixNum(); 00081 00082 uint8_t getDeviceAddr(uint8_t _a); 00083 00084 void setDeviceAddr(uint8_t* _addr); 00085 00086 void clearDisplay(); 00087 00088 void setColor(uint8_t value_r, uint8_t value_g, uint8_t value_b); 00089 void clearColor(); 00090 00091 void setFontMode(bool _Mode); 00092 00093 void setLed(uint8_t row, uint8_t column, bool state); 00094 void setLedColor(uint8_t row, uint8_t column, uint8_t value_r, uint8_t value_g, uint8_t value_b); 00095 void setLedColorFast(uint8_t row, uint8_t column, uint8_t value_r, uint8_t value_g, uint8_t value_b); 00096 00097 void drawLine(int8_t x1, int8_t y1, int8_t x2, int8_t y2); 00098 00099 void drawCircle(int8_t x0, int8_t y0, int8_t rad, int8_t option = U8G_DRAW_ALL); 00100 void drawDisc(int8_t x0, int8_t y0, int8_t rad, int8_t option = U8G_DRAW_ALL); 00101 00102 void drawFrame(int8_t x, int8_t y, int8_t w, int8_t h); 00103 void drawRFrame(int8_t x, int8_t y, int8_t w, int8_t h, uint8_t r); 00104 void drawBox(int8_t x, int8_t y, int8_t w, int8_t h); 00105 void drawRBox(int8_t x, int8_t y, int8_t w, int8_t h, uint8_t r); 00106 00107 void drawBMP(int16_t x, int16_t y, int16_t w, int16_t h,const uint8_t *bitmap); 00108 bool drawBMP(int16_t x, int16_t y, const uint8_t *bitmap); 00109 00110 void setFastMode(); 00111 void clearFastMode(); 00112 00113 virtual size_t write(uint8_t); 00114 00115 void setCursor(int16_t x, int16_t y); 00116 00117 void runFun(const void* Fun = NULL); 00118 void (*Fun)(); 00119 00120 int16_t getStringWidth( char* _String); 00121 int16_t getStringHeight( char* _String); 00122 00123 void writeString(char* _c,bool _m,uint16_t _t,int16_t _xy); 00124 00125 //private: 00126 // bool Fast_mode; 00127 void drawCircle_section(int8_t x, int8_t y, int8_t x0, int8_t y0, uint8_t option); 00128 void drawDisc_section(int8_t x, int8_t y, int8_t x0, int8_t y0, uint8_t option); 00129 void drawVLine(int8_t x, int8_t y, int8_t w); 00130 void drawHLine(int8_t x, int8_t y, int8_t h); 00131 00132 int16_t _numX, _numY; // Display w/h as modified by current rotation 00133 int16_t cursor_x, cursor_y; 00134 int16_t _matrixNum; 00135 }; 00136 00137 00138 #endif
Generated on Thu Jul 14 2022 22:36:21 by
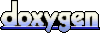