
* AM2321的取温度间隔得大于2s,否则,i2c会不工作了 * SimpleTimer有个bug,会导致两次快速的读温度,现在读温度函数里加了保护 * Blynk有个bug,会导致无法把数据传到服务器 * 现在可以正常工作了
SimpleTimer.cpp
00001 /* 00002 * SimpleTimer.cpp 00003 * 00004 * SimpleTimer - A timer library for Arduino. 00005 * Author: mromani@ottotecnica.com 00006 * Copyright (c) 2010 OTTOTECNICA Italy 00007 * 00008 * This library is free software; you can redistribute it 00009 * and/or modify it under the terms of the GNU Lesser 00010 * General Public License as published by the Free Software 00011 * Foundation; either version 2.1 of the License, or (at 00012 * your option) any later version. 00013 * 00014 * This library is distributed in the hope that it will 00015 * be useful, but WITHOUT ANY WARRANTY; without even the 00016 * implied warranty of MERCHANTABILITY or FITNESS FOR A 00017 * PARTICULAR PURPOSE. See the GNU Lesser General Public 00018 * License for more details. 00019 * 00020 * You should have received a copy of the GNU Lesser 00021 * General Public License along with this library; if not, 00022 * write to the Free Software Foundation, Inc., 00023 * 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00024 */ 00025 00026 00027 #include "SimpleTimer.h" 00028 00029 Timer g_SimpleTimer; 00030 // Select time function: 00031 //static inline unsigned long elapsed() { return micros(); } 00032 //static inline uint32_t elapsed() { return g_SimpleTimer.read_ms(); } 00033 00034 00035 SimpleTimer::SimpleTimer() { 00036 g_SimpleTimer.start(); 00037 uint32_t current_millis = g_SimpleTimer.read_ms(); 00038 00039 for (int i = 0; i < MAX_TIMERS; i++) { 00040 enabled[i] = false; 00041 callbacks[i] = 0; // if the callback pointer is zero, the slot is free, i.e. doesn't "contain" any timer 00042 prev_millis[i] = current_millis; 00043 numRuns[i] = 0; 00044 } 00045 00046 numTimers = 0; 00047 } 00048 00049 00050 void SimpleTimer::run() { 00051 int i; 00052 uint32_t current_millis; 00053 00054 // get current time 00055 current_millis = g_SimpleTimer.read_ms(); 00056 00057 for (i = 0; i < MAX_TIMERS; i++) { 00058 00059 toBeCalled[i] = DEFCALL_DONTRUN; 00060 00061 // no callback == no timer, i.e. jump over empty slots 00062 if (callbacks[i]) { 00063 current_millis = g_SimpleTimer.read_ms(); //LiXianyu added. 00064 // is it time to process this timer ? 00065 // see http://arduino.cc/forum/index.php/topic,124048.msg932592.html#msg932592 00066 00067 if (current_millis - prev_millis[i] >= delays[i]) { 00068 00069 // update time 00070 //prev_millis[i] = current_millis; 00071 //prev_millis[i] += delays[i]; 00072 prev_millis[i] = g_SimpleTimer.read_ms(); // LiXianyu added. 00073 00074 // check if the timer callback has to be executed 00075 if (enabled[i]) { 00076 00077 // "run forever" timers must always be executed 00078 if (maxNumRuns[i] == RUN_FOREVER) { 00079 toBeCalled[i] = DEFCALL_RUNONLY; 00080 //(*callbacks[i])(); 00081 } 00082 // other timers get executed the specified number of times 00083 else if (numRuns[i] < maxNumRuns[i]) { 00084 toBeCalled[i] = DEFCALL_RUNONLY; 00085 numRuns[i]++; 00086 00087 // after the last run, delete the timer 00088 if (numRuns[i] >= maxNumRuns[i]) { 00089 toBeCalled[i] = DEFCALL_RUNANDDEL; 00090 //(*callbacks[i])(); 00091 } 00092 } 00093 } 00094 } 00095 } 00096 } 00097 00098 for (i = 0; i < MAX_TIMERS; i++) { 00099 switch(toBeCalled[i]) { 00100 case DEFCALL_DONTRUN: 00101 break; 00102 00103 case DEFCALL_RUNONLY: 00104 (*callbacks[i])(); 00105 break; 00106 00107 case DEFCALL_RUNANDDEL: 00108 (*callbacks[i])(); 00109 deleteTimer(i); 00110 break; 00111 } 00112 //prev_millis[i] = g_SimpleTimer.read_ms(); // LiXianyu added. 00113 } 00114 } 00115 00116 00117 // find the first available slot 00118 // return -1 if none found 00119 int SimpleTimer::findFirstFreeSlot() { 00120 int i; 00121 00122 // all slots are used 00123 if (numTimers >= MAX_TIMERS) { 00124 return -1; 00125 } 00126 00127 // return the first slot with no callback (i.e. free) 00128 for (i = 0; i < MAX_TIMERS; i++) { 00129 if (callbacks[i] == 0) { 00130 return i; 00131 } 00132 } 00133 00134 // no free slots found 00135 return -1; 00136 } 00137 00138 00139 int SimpleTimer::setTimer(uint32_t d, timer_callback f, int n) { 00140 int freeTimer; 00141 00142 freeTimer = findFirstFreeSlot(); 00143 if (freeTimer < 0) { 00144 return -1; 00145 } 00146 00147 if (f == NULL) { 00148 return -1; 00149 } 00150 00151 delays[freeTimer] = d; 00152 callbacks[freeTimer] = f; 00153 maxNumRuns[freeTimer] = n; 00154 enabled[freeTimer] = true; 00155 prev_millis[freeTimer] = g_SimpleTimer.read_ms(); 00156 00157 numTimers++; 00158 00159 return freeTimer; 00160 } 00161 00162 00163 int SimpleTimer::setInterval(uint32_t d, timer_callback f) { 00164 return setTimer(d, f, RUN_FOREVER); 00165 } 00166 00167 00168 int SimpleTimer::setTimeout(uint32_t d, timer_callback f) { 00169 return setTimer(d, f, RUN_ONCE); 00170 } 00171 00172 00173 void SimpleTimer::deleteTimer(int timerId) { 00174 if (timerId >= MAX_TIMERS) { 00175 return; 00176 } 00177 00178 // nothing to delete if no timers are in use 00179 if (numTimers == 0) { 00180 return; 00181 } 00182 00183 // don't decrease the number of timers if the 00184 // specified slot is already empty 00185 if (callbacks[timerId] != NULL) { 00186 callbacks[timerId] = 0; 00187 enabled[timerId] = false; 00188 toBeCalled[timerId] = DEFCALL_DONTRUN; 00189 delays[timerId] = 0; 00190 numRuns[timerId] = 0; 00191 00192 // update number of timers 00193 numTimers--; 00194 } 00195 } 00196 00197 // function contributed by code@rowansimms.com 00198 void SimpleTimer::restartTimer(int numTimer) { 00199 if (numTimer >= MAX_TIMERS) { 00200 return; 00201 } 00202 00203 prev_millis[numTimer] = g_SimpleTimer.read_ms(); 00204 } 00205 00206 00207 bool SimpleTimer::isEnabled(int numTimer) { 00208 if (numTimer >= MAX_TIMERS) { 00209 return false; 00210 } 00211 00212 return enabled[numTimer]; 00213 } 00214 00215 00216 void SimpleTimer::enable(int numTimer) { 00217 if (numTimer >= MAX_TIMERS) { 00218 return; 00219 } 00220 00221 enabled[numTimer] = true; 00222 } 00223 00224 00225 void SimpleTimer::disable(int numTimer) { 00226 if (numTimer >= MAX_TIMERS) { 00227 return; 00228 } 00229 00230 enabled[numTimer] = false; 00231 } 00232 00233 00234 void SimpleTimer::toggle(int numTimer) { 00235 if (numTimer >= MAX_TIMERS) { 00236 return; 00237 } 00238 00239 enabled[numTimer] = !enabled[numTimer]; 00240 } 00241 00242 00243 int SimpleTimer::getNumTimers() { 00244 return numTimers; 00245 }
Generated on Tue Jul 12 2022 13:01:30 by
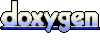