
* AM2321的取温度间隔得大于2s,否则,i2c会不工作了 * SimpleTimer有个bug,会导致两次快速的读温度,现在读温度函数里加了保护 * Blynk有个bug,会导致无法把数据传到服务器 * 现在可以正常工作了
BlynkApiMbed2.h
00001 /** 00002 * @file BlynkApiArduino.h 00003 * @author Volodymyr Shymanskyy 00004 * @license This project is released under the MIT License (MIT) 00005 * @copyright Copyright (c) 2015 Volodymyr Shymanskyy 00006 * @date Mar 2015 00007 * @brief 00008 * 00009 */ 00010 00011 #ifndef BlynkApiArduino_h 00012 #define BlynkApiArduino_h 00013 00014 #include "mbed.h" 00015 #include <Blynk/BlynkApi.h> 00016 00017 00018 static Timer blynk_millis_timer; 00019 static Ticker blynk_waker; 00020 00021 static 00022 void blynk_wake() { 00023 //pc.puts("(...)"); 00024 } 00025 00026 static 00027 void delay(unsigned long ms) 00028 { 00029 wait_ms(ms); 00030 } 00031 00032 static 00033 unsigned long millis(void) 00034 { 00035 return blynk_millis_timer.read_ms(); 00036 } 00037 00038 template<class Proto> 00039 void BlynkApi<Proto>::Init() 00040 { 00041 blynk_waker.attach(&blynk_wake, 2.0); 00042 blynk_millis_timer.start(); 00043 } 00044 00045 template<class Proto> 00046 BLYNK_FORCE_INLINE 00047 millis_time_t BlynkApi<Proto>::getMillis() 00048 { 00049 return blynk_millis_timer.read_ms(); 00050 } 00051 00052 #ifdef BLYNK_NO_INFO 00053 00054 template<class Proto> 00055 BLYNK_FORCE_INLINE 00056 void BlynkApi<Proto>::sendInfo() {} 00057 00058 #else 00059 00060 template<class Proto> 00061 BLYNK_FORCE_INLINE 00062 void BlynkApi<Proto>::sendInfo() 00063 { 00064 static const char profile[] BLYNK_PROGMEM = 00065 BLYNK_PARAM_KV("ver" , BLYNK_VERSION) 00066 BLYNK_PARAM_KV("h-beat" , TOSTRING(BLYNK_HEARTBEAT)) 00067 BLYNK_PARAM_KV("buff-in", TOSTRING(BLYNK_MAX_READBYTES)) 00068 #ifdef BLYNK_INFO_DEVICE 00069 BLYNK_PARAM_KV("dev" , BLYNK_INFO_DEVICE) 00070 #endif 00071 #ifdef BLYNK_INFO_CPU 00072 BLYNK_PARAM_KV("cpu" , BLYNK_INFO_CPU) 00073 #endif 00074 #ifdef BLYNK_INFO_CONNECTION 00075 BLYNK_PARAM_KV("con" , BLYNK_INFO_CONNECTION) 00076 #endif 00077 BLYNK_PARAM_KV("build" , __DATE__ " " __TIME__) 00078 ; 00079 const size_t profile_len = sizeof(profile)-1; 00080 00081 #ifdef BLYNK_HAS_PROGMEM 00082 char mem[profile_len]; 00083 memcpy_P(mem, profile, profile_len); 00084 static_cast<Proto*>(this)->sendCmd(BLYNK_CMD_HARDWARE_INFO, 0, mem, profile_len); 00085 #else 00086 static_cast<Proto*>(this)->sendCmd(BLYNK_CMD_HARDWARE_INFO, 0, profile, profile_len); 00087 #endif 00088 return; 00089 } 00090 00091 #endif 00092 00093 template<class Proto> 00094 BLYNK_FORCE_INLINE 00095 void BlynkApi<Proto>::processCmd(const void* buff, size_t len) 00096 { 00097 BlynkParam param((void*)buff, len); 00098 BlynkParam::iterator it = param.begin(); 00099 if (it >= param.end()) 00100 return; 00101 const char* cmd = it.asStr(); 00102 const uint16_t cmd16 = *(uint16_t*)cmd; 00103 00104 if (++it >= param.end()) 00105 return; 00106 00107 #if defined(analogInputToDigitalPin) 00108 // Good! Analog pins can be referenced on this device by name. 00109 const uint8_t pin = (it.asStr()[0] == 'A') ? 00110 analogInputToDigitalPin(atoi(it.asStr()+1)) : 00111 it.asInt(); 00112 #else 00113 const uint8_t pin = it.asInt(); 00114 #endif 00115 00116 switch(cmd16) { 00117 00118 #ifndef BLYNK_NO_BUILTIN 00119 00120 case BLYNK_HW_PM: { 00121 while (it < param.end()) { 00122 ++it; 00123 if (!strcmp(it.asStr(), "in")) { 00124 //pinMode(pin, INPUT); 00125 } else if (!strcmp(it.asStr(), "out") || !strcmp(it.asStr(), "pwm")) { 00126 //pinMode(pin, OUTPUT); 00127 } else { 00128 #ifdef BLYNK_DEBUG 00129 BLYNK_LOG4(BLYNK_F("Invalid pin "), pin, BLYNK_F(" mode "), it.asStr()); 00130 #endif 00131 } 00132 ++it; 00133 } 00134 } break; 00135 case BLYNK_HW_DR: { 00136 DigitalIn p((PinName)pin); 00137 char mem[16]; 00138 BlynkParam rsp(mem, 0, sizeof(mem)); 00139 rsp.add("dw"); 00140 rsp.add(pin); 00141 rsp.add(int(p)); 00142 static_cast<Proto*>(this)->sendCmd(BLYNK_CMD_HARDWARE, 0, rsp.getBuffer(), rsp.getLength()-1); 00143 } break; 00144 case BLYNK_HW_DW: { 00145 // Should be 1 parameter (value) 00146 if (++it >= param.end()) 00147 return; 00148 00149 //BLYNK_LOG("digitalWrite %d -> %d", pin, it.asInt()); 00150 DigitalOut p((PinName)pin); 00151 p = it.asInt() ? 1 : 0; 00152 } break; 00153 case BLYNK_HW_AR: { 00154 AnalogIn p((PinName)pin); 00155 char mem[16]; 00156 BlynkParam rsp(mem, 0, sizeof(mem)); 00157 rsp.add("aw"); 00158 rsp.add(pin); 00159 rsp.add(int(p.read() * 1024)); 00160 static_cast<Proto*>(this)->sendCmd(BLYNK_CMD_HARDWARE, 0, rsp.getBuffer(), rsp.getLength()-1); 00161 } break; 00162 case BLYNK_HW_AW: { 00163 // TODO: Not supported yet 00164 } break; 00165 00166 #endif 00167 00168 case BLYNK_HW_VR: { 00169 BlynkReq req = { pin }; 00170 WidgetReadHandler handler = GetReadHandler(pin); 00171 if (handler && (handler != BlynkWidgetRead)) { 00172 handler(req); 00173 } else { 00174 BlynkWidgetReadDefault(req); 00175 } 00176 } break; 00177 case BLYNK_HW_VW: { 00178 ++it; 00179 char* start = (char*)it.asStr(); 00180 BlynkParam param2(start, len - (start - (char*)buff)); 00181 BlynkReq req = { pin }; 00182 WidgetWriteHandler handler = GetWriteHandler(pin); 00183 if (handler && (handler != BlynkWidgetWrite)) { 00184 handler(req, param2); 00185 } else { 00186 BlynkWidgetWriteDefault(req, param2); 00187 } 00188 } break; 00189 default: 00190 BLYNK_LOG2(BLYNK_F("Invalid HW cmd: "), cmd); 00191 static_cast<Proto*>(this)->sendCmd(BLYNK_CMD_RESPONSE, static_cast<Proto*>(this)->currentMsgId, NULL, BLYNK_ILLEGAL_COMMAND); 00192 } 00193 } 00194 00195 #endif
Generated on Tue Jul 12 2022 13:01:26 by
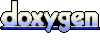