
work fine.
Embed:
(wiki syntax)
Show/hide line numbers
Microduino_Motor.h
00001 #ifndef Motor_h 00002 #define Motor_h 00003 00004 //#include "Arduino.h" 00005 #include "mbed.h" 00006 00007 #define CHAN_LEFT 0 00008 #define CHAN_RIGHT 1 00009 00010 #if 0 00011 typedef struct { 00012 uint8_t nbr_A :6 ; 00013 uint8_t nbr_B :6 ; 00014 } MotorPin_t; 00015 #else 00016 typedef struct { 00017 PinName nbr_A; 00018 PinName nbr_B; 00019 } MotorPin_t; 00020 00021 #endif 00022 00023 typedef struct { 00024 MotorPin_t Pin; 00025 } motor_t; 00026 00027 class Motor 00028 { 00029 public: 00030 Motor(PinName _motor_pinA, PinName _motor_pinB); 00031 void Fix(float _fix); 00032 void Driver(int16_t _motor_driver); 00033 void Free(); 00034 void Brake(); 00035 int16_t GetData(int16_t _throttle, int16_t _steering, uint8_t _dir); 00036 private: 00037 uint8_t motorIndex; // index into the channel data for this key 00038 float fix; 00039 int16_t _motor_vol; 00040 protected: 00041 pwmout_t _pwmA; 00042 pwmout_t _pwmB; 00043 int _period_us; 00044 }; 00045 00046 #endif
Generated on Sat Jul 23 2022 18:32:59 by
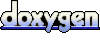