
May 2021 Commit
Dependencies: sx128x sx12xx_hal
main.cpp
00001 #include "mbed.h" 00002 #include "radio.h" 00003 00004 #include "mbed-client-cli/ns_cmdline.h" 00005 #include "mbed-trace/mbed_trace.h" 00006 #define TRACE_GROUP "mast" 00007 00008 #if defined(SX127x_H) || defined(SX126x_H) 00009 #define BW_KHZ 500 00010 #define SPREADING_FACTOR 11 00011 #define CF_HZ 910800000 00012 #if defined(SX126x_H) 00013 #define TX_DBM (Radio::chipType == CHIP_TYPE_SX1262 ? 20 : 14) 00014 #else 00015 #define TX_DBM 17 00016 #endif 00017 #elif defined(SX128x_H) 00018 #define BW_KHZ 200 00019 #define SPREADING_FACTOR 11 00020 #define CF_HZ 2487000000 00021 00022 #define TX_DBM 5 00023 #endif 00024 00025 Timer t; 00026 InterruptIn UserButtonPB2(PB_2); 00027 DigitalIn b[8] = {PB_5,PB_6,PB_8,PB_9,PB_12,PB_13,PB_14,PB_15}; 00028 Thread thread; 00029 // Create a queue that can hold a maximum of 32 events 00030 EventQueue queue(32 * EVENTS_EVENT_SIZE); 00031 00032 00033 volatile bool tx_done; 00034 uint8_t sendCommandFlag = 0; 00035 00036 static uint16_t crc_ccitt( uint8_t *buffer, uint16_t length ) 00037 { 00038 tr_debug(__func__); 00039 const uint16_t polynom = 0x1021; // The CRC calculation follows CCITT 00040 uint16_t crc = 0x0000; // CRC initial value 00041 00042 if( buffer == NULL ) return 0; 00043 00044 for( uint16_t i = 0; i < length; ++i ) 00045 { 00046 crc ^= ( uint16_t ) buffer[i] << 8; 00047 for( uint16_t j = 0; j < 8; ++j ) 00048 { 00049 crc = ( crc & 0x8000 ) ? ( crc << 1 ) ^ polynom : ( crc << 1 ); 00050 } 00051 } 00052 return crc; 00053 } 00054 00055 void transmit(unsigned target, uint8_t cmd) 00056 { 00057 tr_debug(__func__); 00058 unsigned t_diff; 00059 uint16_t crc; 00060 00061 Radio::radio.tx_buf[0] = cmd; 00062 t_diff = target - t.read_us(); 00063 Radio::radio.tx_buf[1] = t_diff >> 24; 00064 Radio::radio.tx_buf[2] = t_diff >> 16; 00065 Radio::radio.tx_buf[3] = t_diff >> 8; 00066 Radio::radio.tx_buf[4] = t_diff & 0xff; 00067 crc = crc_ccitt(Radio::radio.tx_buf, 5); 00068 Radio::radio.tx_buf[5] = crc >> 8; 00069 Radio::radio.tx_buf[6] = crc & 0xff; 00070 Radio::Send(7, 0, 0, 0); 00071 00072 for (tx_done=false;!tx_done;) 00073 { 00074 Radio::service(); 00075 } 00076 tr_debug("t_diff:%u crc:%04x\r\n", t_diff, crc); 00077 } 00078 00079 #define TARGET_LATENCY 2000000 00080 static void send_alarm(uint8_t cmd) 00081 { 00082 tr_debug(__func__); 00083 int i; 00084 unsigned target = t.read_us() + TARGET_LATENCY; 00085 tr_debug("send_alarm() %u\n", target); 00086 00087 for (i = 0; i < 5; i++) 00088 { 00089 transmit(target, cmd); 00090 wait(0.1); 00091 } 00092 } 00093 00094 void txDoneCB() 00095 { 00096 tx_done = true; 00097 } 00098 00099 void rxDoneCB(uint8_t size, float Rssi, float Snr) 00100 { 00101 } 00102 00103 const RadioEvents_t rev = 00104 { 00105 /* Dio0_top_half */ NULL, 00106 /* TxDone_topHalf */ NULL, 00107 /* TxDone_botHalf */ txDoneCB, 00108 /* TxTimeout */ NULL, 00109 /* RxDone */ rxDoneCB, 00110 /* RxTimeout */ NULL, 00111 /* RxError */ NULL, 00112 /* FhssChangeChannel */NULL, 00113 /* CadDone */ NULL 00114 }; 00115 00116 00117 static Mutex serialOutMutex; 00118 static void serial_out_mutex_wait() 00119 { 00120 serialOutMutex.lock(); 00121 } 00122 00123 static void serial_out_mutex_release() 00124 { 00125 osStatus s = serialOutMutex.unlock(); 00126 MBED_ASSERT(s == osOK); 00127 } 00128 00129 static int cmd_transmitByte(int argc, char *argv[]) 00130 { 00131 tr_debug("%s args: %d",__FUNCTION__,argc); 00132 if(argc != 2) 00133 { 00134 tr_debug("Invalid argument count"); 00135 return CMDLINE_RETCODE_INVALID_PARAMETERS; 00136 } 00137 uint8_t byte = atoi(argv[1]); 00138 tr_debug("sending byte: %d",byte); 00139 send_alarm(byte); 00140 return CMDLINE_RETCODE_SUCCESS; 00141 } 00142 00143 static void manual_transmission_queue_callback(void) 00144 { 00145 uint8_t byte = 0; 00146 uint8_t value = 0; 00147 tr_debug("Parallel Port Data Input"); 00148 for (int i = 0; i< 8; i++) 00149 { 00150 value = 0; 00151 value = b[i].read(); 00152 //tr_debug("bit %d, value: %d",i,value); 00153 value = value << i; 00154 //tr_debug("value shifted: %d",value); 00155 byte |= value; 00156 //tr_debug("byte: %d",byte); 00157 } 00158 tr_debug("sending byte: %d",byte); 00159 send_alarm(byte); 00160 } 00161 00162 static void manual_transmission(void) 00163 { 00164 00165 queue.call(manual_transmission_queue_callback); 00166 } 00167 00168 00169 00170 int main() 00171 { 00172 00173 00174 mbed_trace_mutex_wait_function_set( serial_out_mutex_wait ); 00175 mbed_trace_mutex_release_function_set( serial_out_mutex_release ); 00176 mbed_trace_init(); 00177 tr_debug(__FUNCTION__); 00178 tr_debug("Program Entry"); 00179 00180 thread.start(callback(&queue, &EventQueue::dispatch_forever)); 00181 UserButtonPB2.rise(&manual_transmission); 00182 00183 wait(0.05); 00184 t.start(); 00185 00186 Radio::Init(&rev); 00187 Radio::Standby(); 00188 Radio::LoRaModemConfig(BW_KHZ, SPREADING_FACTOR, 1); 00189 Radio::LoRaPacketConfig(8, false, true, false); // preambleLen, fixLen, crcOn, invIQ 00190 Radio::SetChannel(CF_HZ); 00191 Radio::set_tx_dbm(TX_DBM); 00192 00193 tr_debug("Initializing Command Line"); 00194 cmd_init(0); 00195 cmd_mutex_wait_func( serial_out_mutex_wait ); 00196 cmd_mutex_release_func( serial_out_mutex_release ); 00197 cmd_add("transmit-byte", cmd_transmitByte,"Transmit Byte","Transmit a decimal byte over LoRa radio"); 00198 00199 00200 while(true) 00201 { 00202 00203 int c = getchar(); 00204 if (c != EOF) 00205 { 00206 cmd_char_input(c); 00207 } 00208 } 00209 }
Generated on Sat Aug 6 2022 00:28:43 by
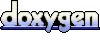