
SW encryption
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include <stdio.h> 00002 #include <iostream> 00003 00004 #include "lorawan/LoRaWANInterface.h" 00005 #include "lorawan/system/lorawan_data_structures.h" 00006 #include "events/EventQueue.h" 00007 00008 // Application helpers 00009 #include "DummySensor.h" 00010 #include "trace_helper.h" 00011 #include "lora_radio_helper.h" 00012 00013 #include "sha256.h" 00014 00015 using namespace events; 00016 00017 // Max payload size can be LORAMAC_PHY_MAXPAYLOAD. 00018 // This example only communicates with much shorter messages (<30 bytes). 00019 // If longer messages are used, these buffers must be changed accordingly. 00020 uint8_t tx_buffer[128]; 00021 uint8_t rx_buffer[128]; 00022 00023 /* 00024 * Sets up an application dependent transmission timer in ms. Used only when Duty Cycling is off for testing 00025 */ 00026 #define TX_TIMER 10000 00027 00028 /** 00029 * Maximum number of events for the event queue. 00030 * 10 is the safe number for the stack events, however, if application 00031 * also uses the queue for whatever purposes, this number should be increased. 00032 */ 00033 #define MAX_NUMBER_OF_EVENTS 10 00034 00035 /** 00036 * Maximum number of retries for CONFIRMED messages before giving up 00037 */ 00038 #define CONFIRMED_MSG_RETRY_COUNTER 3 00039 00040 /** 00041 * Dummy pin for dummy sensor 00042 */ 00043 #define PC_9 0 00044 00045 /** 00046 * Dummy sensor class object 00047 */ 00048 DS1820 ds1820(PC_9); 00049 00050 /** 00051 * This event queue is the global event queue for both the 00052 * application and stack. To conserve memory, the stack is designed to run 00053 * in the same thread as the application and the application is responsible for 00054 * providing an event queue to the stack that will be used for ISR deferment as 00055 * well as application information event queuing. 00056 */ 00057 static EventQueue ev_queue(MAX_NUMBER_OF_EVENTS *EVENTS_EVENT_SIZE); 00058 00059 /** 00060 * Event handler. 00061 * 00062 * This will be passed to the LoRaWAN stack to queue events for the 00063 * application which in turn drive the application. 00064 */ 00065 static void lora_event_handler(lorawan_event_t event); 00066 00067 /** 00068 * Constructing Mbed LoRaWANInterface and passing it the radio object from lora_radio_helper. 00069 */ 00070 static LoRaWANInterface lorawan(radio); 00071 00072 /** 00073 * Application specific callbacks 00074 */ 00075 static lorawan_app_callbacks_t callbacks; 00076 00077 /** 00078 * Entry point for application 00079 */ 00080 int main(void) 00081 { 00082 // setup tracing 00083 setup_trace(); 00084 00085 // stores the status of a call to LoRaWAN protocol 00086 lorawan_status_t loraStatus; 00087 00088 // Initialize LoRaWAN stack 00089 if (lorawan.initialize(&ev_queue) != LORAWAN_STATUS_OK) { 00090 printf("\r\n LoRa initialization failed! \r\n"); 00091 return -1; 00092 } 00093 00094 printf("\r\n Mbed LoRaWANStack initialized \r\n"); 00095 00096 // prepare application callbacks 00097 callbacks.events = mbed::callback(lora_event_handler); 00098 lorawan.add_app_callbacks(&callbacks); 00099 00100 // Set number of retries in case of CONFIRMED messages 00101 if (lorawan.set_confirmed_msg_retries(CONFIRMED_MSG_RETRY_COUNTER) 00102 != LORAWAN_STATUS_OK) { 00103 printf("\r\n set_confirmed_msg_retries failed! \r\n\r\n"); 00104 return -1; 00105 } 00106 00107 printf("\r\n CONFIRMED message retries : %d \r\n", 00108 CONFIRMED_MSG_RETRY_COUNTER); 00109 00110 // Enable adaptive data rate 00111 if (lorawan.enable_adaptive_datarate() != LORAWAN_STATUS_OK) { 00112 printf("\r\n enable_adaptive_datarate failed! \r\n"); 00113 return -1; 00114 } 00115 00116 printf("\r\n Adaptive data rate (ADR) - Enabled \r\n"); 00117 00118 loraStatus = lorawan.connect(); 00119 00120 if (loraStatus == LORAWAN_STATUS_OK || 00121 loraStatus == LORAWAN_STATUS_CONNECT_IN_PROGRESS) { 00122 } else { 00123 printf("\r\n Connection error, code = %d \r\n", loraStatus); 00124 return -1; 00125 } 00126 printf("\r\n Connection - In Progress ...\r\n"); 00127 00128 // make your event queue dispatching events forever 00129 ev_queue.dispatch_forever(); 00130 00131 return 0; 00132 } 00133 00134 /** 00135 * Sends a message to the Network Server 00136 */ 00137 static void send_message() { 00138 uint16_t packet_cont; 00139 int16_t loraStatus; 00140 uint8_t hash_content; 00141 uint8_t sensor_val; 00142 unsigned char output[32]; 00143 00144 //Begin sensor 00145 if (ds1820.begin()) { 00146 ds1820.startConversion(); 00147 sensor_val = ds1820.read(); 00148 ds1820.startConversion(); 00149 } else { 00150 printf("\r\n No sensor found \r\n"); 00151 return; 00152 } 00153 00154 hash_content = sensor_val; //Assign sensor value to hash_content 00155 printf("\r\n %d sensor value \r\n", hash_content); //print to check value 00156 char hash_str[] = ""; //create new char array 00157 sprintf(hash_str, "%d", hash_content); //put value into new char array 00158 00159 static const unsigned char * hash_buffer = (const unsigned char * ) hash_str; //cast char array to const unsigned char 00160 static const size_t hash_len = strlen(hash_str); //get size of message 00161 00162 00163 mbedtls_sha256(hash_buffer, hash_len, output, 0); //hash message 00164 00165 const char * c = (const char * ) output; //put hashed message into char 00166 00167 packet_cont = sprintf((char * ) tx_buffer, "%d%s",hash_content , c); //build message with original value + hashed message 00168 00169 loraStatus = lorawan.send(MBED_CONF_LORA_APP_PORT, tx_buffer, packet_cont, //send message 00170 MSG_UNCONFIRMED_FLAG); 00171 00172 if (loraStatus < 0) { //perform checks to confirm message is sent 00173 loraStatus == LORAWAN_STATUS_WOULD_BLOCK ? printf("send - WOULD BLOCK\r\n") : 00174 printf("\r\n send() - Error code %d \r\n", loraStatus); 00175 return; 00176 } 00177 00178 printf("\r\n %d bytes scheduled for transmission \r\n", loraStatus); //print if successful and clear tx buffer 00179 memset(tx_buffer, 0, sizeof(tx_buffer)); 00180 } 00181 /** 00182 * Receive a message from the Network Server 00183 */ 00184 static void receive_message() 00185 { 00186 uint8_t port; 00187 int flags; 00188 int16_t retcode = lorawan.receive(rx_buffer, sizeof(rx_buffer), port, flags); 00189 00190 if (retcode < 0) { 00191 printf("\r\n receive() - Error code %d \r\n", retcode); 00192 return; 00193 } 00194 00195 printf(" RX Data on port %u (%d bytes): ", port, retcode); 00196 for (uint8_t i = 0; i < retcode; i++) { 00197 printf("%02x ", rx_buffer[i]); 00198 } 00199 printf("\r\n"); 00200 00201 memset(rx_buffer, 0, sizeof(rx_buffer)); 00202 } 00203 00204 /** 00205 * Event handler 00206 */ 00207 static void lora_event_handler(lorawan_event_t event) 00208 { 00209 switch (event) { 00210 case CONNECTED: 00211 printf("\r\n Connection - Successful \r\n"); 00212 if (MBED_CONF_LORA_DUTY_CYCLE_ON) { 00213 send_message(); 00214 } else { 00215 ev_queue.call_every(TX_TIMER, send_message); 00216 } 00217 00218 break; 00219 case DISCONNECTED: 00220 ev_queue.break_dispatch(); 00221 printf("\r\n Disconnected Successfully \r\n"); 00222 break; 00223 case TX_DONE: 00224 printf("\r\n Message Sent to Network Server \r\n"); 00225 if (MBED_CONF_LORA_DUTY_CYCLE_ON) { 00226 send_message(); 00227 } 00228 break; 00229 case TX_TIMEOUT: 00230 case TX_ERROR: 00231 case TX_CRYPTO_ERROR: 00232 case TX_SCHEDULING_ERROR: 00233 printf("\r\n Transmission Error - EventCode = %d \r\n", event); 00234 // try again 00235 if (MBED_CONF_LORA_DUTY_CYCLE_ON) { 00236 send_message(); 00237 } 00238 break; 00239 case RX_DONE: 00240 printf("\r\n Received message from Network Server \r\n"); 00241 receive_message(); 00242 break; 00243 case RX_TIMEOUT: 00244 case RX_ERROR: 00245 printf("\r\n Error in reception - Code = %d \r\n", event); 00246 break; 00247 case JOIN_FAILURE: 00248 printf("\r\n OTAA Failed - Check Keys \r\n"); 00249 break; 00250 case UPLINK_REQUIRED: 00251 printf("\r\n Uplink required by NS \r\n"); 00252 if (MBED_CONF_LORA_DUTY_CYCLE_ON) { 00253 send_message(); 00254 } 00255 break; 00256 default: 00257 MBED_ASSERT("Unknown Event"); 00258 } 00259 } 00260 00261 // EOF
Generated on Sat Jul 16 2022 16:11:52 by
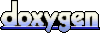