
Working sample which demonstrates the Http Server implementation using WiFlyHTTPServer library.
Dependencies: WiFlyHTTPServer WiflyInterface mbed-rpc mbed
main.cpp
00001 #include "mbed.h" 00002 #include "HTTPServer.h" 00003 #include "FsHandler.h" 00004 #include "RpcHandler.h" 00005 #include "LocalFileSystem.h" 00006 #include "mbed_rpc.h" 00007 00008 DigitalOut myled(LED1); 00009 00010 LocalFileSystem local("local"); 00011 00012 HTTPServer svr(p9, p10, p30, p29, "<Your AP Name>", "<Your Passphrase>", WPA); 00013 00014 00015 RpcDigitalOut Led1(LED1, "Led1"); 00016 RpcDigitalOut Led2(LED2, "Led2"); 00017 RpcDigitalOut Led3(LED3, "Led3"); 00018 RpcDigitalOut Led4(LED4, "Led4"); 00019 00020 int main() { 00021 std::string tim; 00022 00023 /* Mount the local file system. */ 00024 HTTPFsRequestHandler::mount("/local/", "/"); 00025 00026 /* Add handler for file system access */ 00027 svr.addHandler<HTTPFsRequestHandler>("/"); 00028 /* Add handler to acces RPC objects */ 00029 svr.addHandler<HTTPRpcRequestHandler>("/rpc/"); 00030 00031 /* Start the server which will listen for incoming connections on port 80 */ 00032 svr.start(80); 00033 00034 00035 while(1) { 00036 /* get the time and the uptime from WiFly */ 00037 tim = svr.getTime(); 00038 printf("Current time is : %s\n", tim.c_str()); 00039 00040 if (svr.poll(false) >= 0) { 00041 myled = !myled; 00042 } 00043 else { 00044 error("WiFly Polling failed."); 00045 } 00046 00047 } 00048 }
Generated on Sat Jul 16 2022 18:58:29 by
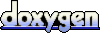