This library allows to parse and work with data sent by the Paradigma pelletti oven.
Embed:
(wiki syntax)
Show/hide line numbers
ParadigmaDateTime.h
00001 #ifndef __PARADIGMADATETIME_H__ 00002 #define __PARADIGMADATETIME_H__ 00003 00004 #include <string> 00005 00006 namespace Paradigma { 00007 00008 __packed class ParadigmaDateTime 00009 { 00010 protected: 00011 int bcdToDec(char n) const { return (((unsigned)n)>>4)*10 + (n&0x0f); } 00012 00013 private: 00014 unsigned char m_Date; 00015 unsigned char m_Month; 00016 unsigned char m_Minute; 00017 unsigned char m_Hour; 00018 00019 00020 public: 00021 ParadigmaDateTime() : m_Date(0), m_Month(0), m_Minute(0), m_Hour(0) {} 00022 00023 operator string() const { char Buffer[15]; sprintf(Buffer, "%02d.%02d, %02d:%02d", getDate(), getMonth(), getHour(), getMinute()); return Buffer; } 00024 00025 std::string getDateString() { char Buffer[15]; sprintf(Buffer, "%02d.%02d", getDate(), getMonth()); return Buffer; } 00026 std::string getTimeString() { char Buffer[15]; sprintf(Buffer, "%02d:%02d", getHour(), getMinute()); return Buffer; } 00027 00028 unsigned char getDate() const { return bcdToDec(m_Date); } 00029 unsigned char getMonth() const { return bcdToDec(m_Month); } 00030 unsigned char getMinute() const { return bcdToDec(m_Minute); } 00031 unsigned char getHour() const { return bcdToDec(m_Hour); } 00032 }; 00033 } 00034 #endif // __PARADIGMADATETIME_H__
Generated on Wed Jul 13 2022 16:47:28 by
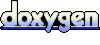