This library allows to parse and work with data sent by the Paradigma pelletti oven.
Embed:
(wiki syntax)
Show/hide line numbers
ParadigmaData.h
00001 #ifndef __PARADIGMA_DATA_H__ 00002 #define __PARADIGMA_DATA_H__ 00003 00004 #include "ParadigmaBase.h" 00005 #include "ParadigmaTemperature.h" 00006 #include "ParadigmaDateTime.h" 00007 00008 #include <string> 00009 using namespace std; 00010 00011 namespace Paradigma 00012 { 00013 00014 /** ParadigmaBlockType_t enumerates valid values for the block type. The block type is always the first 00015 * data byte in a response from paradigma. There are currently two known values. 00016 */ 00017 typedef __packed enum { 00018 ParadigmaVariables = 0xFC, 00019 ParadigmaParameters = 0xFD, 00020 } ParadigmaBlockType_t; 00021 00022 /** ParadigmaMessageID_t specifies the content of the transmitted object. Currently only one valid value 00023 * is known which indicates a valuid variable or parameter block is being transmitted */ 00024 typedef __packed enum { 00025 ParadigmaMessage = 0x0C, 00026 } ParadigmaMessageID_t; 00027 00028 /** ParadigmaDatasetType_t specifies which type of information is contained in the datablock. Currently 00029 * known values are : Dataset1 and Dataset2 for Variables, and Parameters for different settings which 00030 * can be changed and queried by the user. 00031 */ 00032 typedef __packed enum { 00033 ParadigmaMonitorDataset1 = 0x01, 00034 ParadigmaMonitorDataset2 = 0x02, 00035 ParadigmaParameterset = 0x03, 00036 ParadigmaUnknown1 = 0x14, 00037 Invalid = 0xFF 00038 } ParadigmaDatasetType_t; 00039 00040 typedef char ParadigmaParameterAddress_t[3]; 00041 00042 const ParadigmaParameterAddress_t ParadigmaAdresses[] = { 00043 {0x00, 0x02, 0x2A}, // Heizkreis 1 00044 {0x01, 0x88, 0x2A}, // Heizkreis 2 00045 {0x03, 0x0E, 0x09}, // Warmwasser 00046 {0x03, 0xF7, 0x0F}, // Anlagendaten Kessel/Puffer und Zirkulation 00047 {0x03, 0xE6, 0x12}, // Wartung Telefonnummer 00048 {0x03, 0x17, 0x70}, // Warmwasserprogramm 1 00049 {0x03, 0x87, 0x70}, // Warmwasserprogramm 2 00050 {0x04, 0x06, 0x70}, // Zirkulationszeitprogramm 1 00051 {0x04, 0x76, 0x70}, // Zirkulationszeitprogramm 2 00052 {0x00, 0x2C, 0x70}, // Heizzeitprogramm 1 HK1 00053 {0x00, 0x9C, 0x70}, // Heizzeitprogramm 2 HK1 00054 {0x01, 0x0C, 0x70}, // Heizzeitprogramm 3 HK1 00055 {0x01, 0xB2, 0x70}, // Heizzeitprogramm 1 HK2 00056 {0x02, 0x22, 0x70}, // Heizzeitprogramm 2 HK2 00057 {0x02, 0x92, 0x70}, // Heizzeitprogramm 3 HK2 00058 {0x05, 0x08, 0x03} // Anlagendaten Kessel/Puffer 2 00059 }; 00060 00061 /** ParadigmaBlockHeader_t represents the header of any sent block by paradigma. */ 00062 typedef __packed struct { 00063 ParadigmaBlockType_t block_type; // 0xFC for normal observable variables, 0xFD for parameter blocks 00064 unsigned char block_length; 00065 ParadigmaMessageID_t message_id; // always 0x0C 00066 ParadigmaDatasetType_t dataset_type; 00067 } ParadigmaBlockHeader_t; 00068 00069 typedef __packed struct { 00070 ParadigmaDateTime DateTime; 00071 ParadigmaTemperature Aussentemp; // (in 0,1 Grad Schritten) 00072 ParadigmaTemperature Warmwassertemp; // (in 0,1 Grad Schritten) 00073 ParadigmaTemperature Kesselvorlauf; // (in 0,1 Grad Schritten) 00074 ParadigmaTemperature Kesselruecklauf; // (in 0,1 Grad Schritten) 00075 00076 ParadigmaTemperature RaumtemperaturHK1; // (in 0,1 Grad Schritten) 00077 ParadigmaTemperature RaumtemperaturHK2; // (in 0,1 Grad Schritten) 00078 ParadigmaTemperature VorlauftemperaturHK1; // (in 0,1 Grad Schritten) 00079 ParadigmaTemperature VorlauftemperaturHK2; // (in 0,1 Grad Schritten) 00080 ParadigmaTemperature RuecklauftemperaturHK1; // (in 0,1 Grad Schritten) 00081 ParadigmaTemperature RuecklauftemperaturHK2; // (in 0,1 Grad Schritten) 00082 ParadigmaTemperature PuffertemperaturOben; // (in 0,1 Grad Schritten) 00083 ParadigmaTemperature PuffertemperaturUnten; // (in 0,1 Grad Schritten) 00084 ParadigmaTemperature Zirkulationstemperatur; // (in 0,1 Grad Schritten) 00085 00086 byte Checksumme; 00087 } MonDta1_t; 00088 00089 typedef __packed struct { 00090 ParadigmaTemperature RaumsollHK1; 00091 ParadigmaTemperature RaumsollHK2; 00092 ParadigmaTemperature VorlaufsollHK1; 00093 ParadigmaTemperature VorlaufsollHK2; 00094 ParadigmaTemperature Warmwassersolltemp; 00095 ParadigmaTemperature Puffersolltemp; 00096 __packed struct { 00097 word PHK1:1; 00098 word PHK2:1; 00099 word PK:1; 00100 word Mischer1Auf:1; 00101 word Mischer1Zu:1; 00102 word Mischer2Auf:1; 00103 word Mischer2Zu:1; 00104 word ULV:1; 00105 word PZ:1; 00106 word B1:1; 00107 word Taster:1; 00108 word LONModul:1; 00109 word OTModul:1; 00110 word reserver:3; 00111 } ZustandAusgaenge; 00112 ParadigmaDword BetriebsstundenKessel; 00113 ParadigmaDword AnzahlKesselstarts; 00114 ParadigmaWord StoercodeKessel; 00115 byte StoercodeFuehler; 00116 byte BetriebsartHK1; 00117 byte NiveauHK1; 00118 byte BetriebsartHK2; 00119 byte NiveauHK2; 00120 byte LeistungPHK1; 00121 byte LeistungPHK2; 00122 byte LeistungPK; 00123 00124 byte Checksumme; 00125 } MonDta2_t; 00126 00127 00128 /** Class ParadigmaMonitorData encapsulates the data representation and parsing of the 00129 * data blocks that are being sent by the paradigma heater. The class also provides 00130 * a stream type functionality to detect and parse the data from a stream of chars. 00131 */ 00132 class ParadigmaMonitorData 00133 { 00134 FunctionPointer m_CallbackData1; 00135 FunctionPointer m_CallbackData2; 00136 00137 MonDta1_t m_Data1; 00138 MonDta2_t m_Data2; 00139 00140 ParadigmaBlockHeader_t m_Header; 00141 ParadigmaDatasetType_t m_activeDataBlock; 00142 int m_actualPos; 00143 char m_checksum; 00144 00145 void invalidateHeader(); 00146 void callBack1() { 00147 m_CallbackData1.call(); 00148 } 00149 void callBack2() { 00150 m_CallbackData2.call(); 00151 } 00152 word swapWord(word d) { 00153 return ((d&0xFF)<<8) | ((d>>8)&0xFF); 00154 } 00155 ulong swapDWord(ulong d) { 00156 return ((d>>24)&0xFF) | ((d>>8)&0x0000FF00) | ((d<<8)&0x00FF0000) | ((d<<24)&0xFF000000); 00157 } 00158 public: 00159 /** Public constructor. Will initialize all internal data and variables. */ 00160 ParadigmaMonitorData(): m_checksum(0) { 00161 memset(&m_Data1, 0, sizeof(m_Data1)); 00162 memset(&m_Data2, 0, sizeof(m_Data2)); 00163 invalidateHeader(); 00164 } 00165 /** Access function to get the transmitted date time object. 00166 * @returns : the date and time objects of the transmitted data. 00167 */ 00168 ParadigmaDateTime getDateTime() { 00169 return m_Data1.DateTime; 00170 } 00171 /** Access function to the Outside air temperature (AUSSENTEMP). 00172 * @returns : the outside air temperature. 00173 */ 00174 ParadigmaTemperature getAussentemp() { 00175 return m_Data1.Aussentemp; 00176 } 00177 /** Access function to the warm water temperature (WARMWASSERTEMP). 00178 * @returns : the warm water temperature. 00179 */ 00180 ParadigmaTemperature getWarmwassertemp() { 00181 return m_Data1.Warmwassertemp; 00182 } 00183 /** Access function to the 'kessel vorlauf' temperature. 00184 * @returns : the temperature of the 'kessel vorlauf'. 00185 */ 00186 ParadigmaTemperature getKesselvorlauf() { 00187 return m_Data1.Kesselvorlauf; 00188 } 00189 /** Access function to the 'kessel ruecklauf' temperature. 00190 * @returns : the temperature of the 'kessel ruecklauf'. 00191 */ 00192 ParadigmaTemperature getKesselruecklauf() { 00193 return m_Data1.Kesselruecklauf; 00194 } 00195 /** Access function to the room temperature of heating circuit 1. 00196 * @returns : the room temperature of heating circuit 1. 00197 */ 00198 ParadigmaTemperature getRaumtemperaturHK1() { 00199 return m_Data1.RaumtemperaturHK1; 00200 } 00201 /** Access function to the room temperature of heating circuit 2. 00202 * @returns : the room temperature of heating circuit 2. 00203 */ 00204 ParadigmaTemperature getRaumtemperaturHK2() { 00205 return m_Data1.RaumtemperaturHK2; 00206 } 00207 ParadigmaTemperature getVorlauftemperaturHK1() { 00208 return m_Data1.VorlauftemperaturHK1; 00209 } 00210 ParadigmaTemperature getVorlauftemperaturHK2() { 00211 return m_Data1.VorlauftemperaturHK2; 00212 } 00213 ParadigmaTemperature getRuecklauftemperaturHK1() { 00214 return m_Data1.RuecklauftemperaturHK1; 00215 } 00216 ParadigmaTemperature getRuecklauftemperaturHK2() { 00217 return m_Data1.RuecklauftemperaturHK2; 00218 } 00219 ParadigmaTemperature getPuffertemperaturOben() { 00220 return m_Data1.PuffertemperaturOben; 00221 } 00222 ParadigmaTemperature getPuffertemperaturUnten() { 00223 return m_Data1.PuffertemperaturUnten; 00224 } 00225 ParadigmaTemperature getZirkulationstemperatur() { 00226 return m_Data1.Zirkulationstemperatur; 00227 } 00228 00229 ParadigmaTemperature getRaumsollHK1() { 00230 return m_Data2.RaumsollHK1; 00231 } 00232 ParadigmaTemperature getRaumsollHK2() { 00233 return m_Data2.RaumsollHK2; 00234 } 00235 ParadigmaTemperature getVorlaufsollHK1() { 00236 return m_Data2.VorlaufsollHK1; 00237 } 00238 ParadigmaTemperature getVorlaufsollHK2() { 00239 return m_Data2.VorlaufsollHK2; 00240 } 00241 ParadigmaTemperature getWarmwassersolltemp() { 00242 return m_Data2.Warmwassersolltemp; 00243 } 00244 ParadigmaTemperature getPuffersolltemp() { 00245 return m_Data2.Puffersolltemp; 00246 } 00247 00248 /** Function will let the user select the temperature to be returned. 00249 * @param sel : Will specify which temperature value to return. 00250 * @returns : the temperature object as selected by param sel. 00251 */ 00252 ParadigmaTemperature getTemperature(ParadigmaTemperatureSelector_t sel); 00253 00254 ulong getBetriebsstundenKessel() { 00255 return (ulong)m_Data2.BetriebsstundenKessel; 00256 } 00257 ulong getAnzahlKesselstarts() { 00258 return (ulong)m_Data2.AnzahlKesselstarts; 00259 } 00260 word getStoercodeKessel() { 00261 return (word)m_Data2.StoercodeKessel; 00262 } 00263 byte getStoercodeFuehler() { 00264 return (byte)m_Data2.StoercodeFuehler; 00265 } 00266 00267 00268 ParadigmaMonitorData& operator<<( char c ); 00269 ParadigmaMonitorData& operator<<( char Buffer[] ); 00270 00271 void attach1( void (*fct)(void) ) { 00272 m_CallbackData1.attach(fct); 00273 } 00274 void attach2( void (*fct)(void) ) { 00275 m_CallbackData2.attach(fct); 00276 } 00277 00278 template<typename T> 00279 void attach1(T* p, void (T::*mptr)(void)) { 00280 m_CallbackData1.attach(p, mptr); 00281 } 00282 template<typename T> 00283 void attach2(T* p, void (T::*mptr)(void)) { 00284 m_CallbackData2.attach(p, mptr); 00285 } 00286 00287 MonDta1_t * getData1() { return &m_Data1; } 00288 MonDta2_t * getData2() { return &m_Data2; } 00289 }; 00290 } 00291 #endif
Generated on Wed Jul 13 2022 16:47:28 by
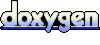