This library allows to parse and work with data sent by the Paradigma pelletti oven.
Embed:
(wiki syntax)
Show/hide line numbers
ParadigmaData.cpp
00001 #include "mbed.h" 00002 #include "ParadigmaData.h" 00003 #ifndef DEBUG 00004 #define DEBUG 00005 #endif 00006 #include "debug.h" 00007 00008 using namespace Paradigma; 00009 static char m_Buffer[50]; 00010 00011 ParadigmaMonitorData& ParadigmaMonitorData::operator<<(char c) 00012 { 00013 int nLen = 0; 00014 if (m_activeDataBlock != Invalid) { 00015 // The actual data block had been detected already, so write into the data block 00016 m_Buffer[m_actualPos++] = c; 00017 // Build checksum on the fly 00018 m_checksum += c; 00019 // Now check if all bytes have been received already 00020 if (m_activeDataBlock == ParadigmaMonitorDataset1) { 00021 // for datablock 1 00022 nLen = sizeof(m_Data1); 00023 if (nLen == m_actualPos) { 00024 // all bytes received, so reset counter and check if checksum is matching 00025 if (m_checksum != 0) { 00026 ERR("Data corruption error. Received invalid data ! (checksum is %02x)", m_checksum); 00027 } else { 00028 memcpy(&m_Data1, m_Buffer, nLen); 00029 m_Data1.Aussentemp.adjustEndiness(); 00030 m_Data1.Warmwassertemp.adjustEndiness(); 00031 m_Data1.Kesselvorlauf.adjustEndiness(); 00032 m_Data1.Kesselruecklauf.adjustEndiness(); 00033 m_Data1.RaumtemperaturHK1.adjustEndiness(); 00034 m_Data1.RaumtemperaturHK2.adjustEndiness(); 00035 m_Data1.VorlauftemperaturHK1.adjustEndiness(); 00036 m_Data1.VorlauftemperaturHK2.adjustEndiness(); 00037 m_Data1.RuecklauftemperaturHK1.adjustEndiness(); 00038 m_Data1.RuecklauftemperaturHK2.adjustEndiness(); 00039 m_Data1.PuffertemperaturOben.adjustEndiness(); 00040 m_Data1.PuffertemperaturUnten.adjustEndiness(); 00041 m_Data1.Zirkulationstemperatur.adjustEndiness(); 00042 INFO("*********************** received new data1 !"); 00043 callBack1(); 00044 } 00045 invalidateHeader(); 00046 } 00047 } else { 00048 // for datablock 2 00049 nLen = sizeof(m_Data2); 00050 if (nLen == m_actualPos) { 00051 // all bytes received, so reset counter and check if checksum is matching 00052 if (m_checksum != 0) { 00053 ERR("Data corruption error. Received invalid data ! (checksum is %02x)", m_checksum); 00054 } else { 00055 memcpy(&m_Data2, m_Buffer, nLen); 00056 m_Data2.RaumsollHK1.adjustEndiness(); 00057 m_Data2.RaumsollHK2.adjustEndiness(); 00058 m_Data2.VorlaufsollHK1.adjustEndiness(); 00059 m_Data2.VorlaufsollHK2.adjustEndiness(); 00060 m_Data2.Warmwassersolltemp.adjustEndiness(); 00061 m_Data2.Puffersolltemp.adjustEndiness(); 00062 m_Data2.BetriebsstundenKessel.adjustEndiness(); 00063 m_Data2.AnzahlKesselstarts.adjustEndiness(); 00064 m_Data2.StoercodeKessel.adjustEndiness(); 00065 INFO("*********************** received new data2 !"); 00066 callBack2(); 00067 } 00068 invalidateHeader(); 00069 } 00070 } 00071 } else { 00072 // Still trying to detect a valid data block, check to see if a valid address-part is here 00073 switch (m_actualPos) { 00074 case 0 : // block type 00075 if ( (c== ParadigmaParameters) || (c== ParadigmaVariables)) { 00076 m_Header.block_type = (ParadigmaBlockType_t)c; 00077 m_actualPos++; 00078 m_checksum = c; 00079 INFO("Block start !\n"); 00080 } 00081 break; 00082 00083 case 1 : // length 00084 m_Header.block_length = c; 00085 m_actualPos++; 00086 m_checksum += c; 00087 INFO("Block len !\n"); 00088 break; 00089 00090 case 2 : // Message ID 00091 if ( c == ParadigmaMessage ) { 00092 m_Header.message_id = (ParadigmaMessageID_t)c; 00093 m_actualPos++; 00094 m_checksum += c; 00095 } else { 00096 invalidateHeader(); 00097 INFO("Rejected due to incorrect Message ID %d\n", c); 00098 } 00099 break; 00100 00101 case 3 : // 00102 if ( (c == ParadigmaMonitorDataset1) || (c == ParadigmaMonitorDataset2)) { 00103 m_Header.dataset_type = (ParadigmaDatasetType_t)c; 00104 m_actualPos = 0; 00105 m_checksum += c; 00106 m_activeDataBlock = (ParadigmaDatasetType_t)c; 00107 INFO("Dataset Number %d!\n", c); 00108 } else { 00109 invalidateHeader(); 00110 INFO("Rejected due to incorrect Dataset number %d\n", c); 00111 } 00112 break; 00113 00114 default: 00115 invalidateHeader(); 00116 break; 00117 } 00118 } 00119 return *this; 00120 } 00121 00122 /** Function will reset all header and actual position information so that search for valid header 00123 * will be reset and starting from beginning with receival of next character. 00124 * 00125 * Will have to clearup the header itsself and the checksum. Also the actual data block information 00126 * needs to be invalidated. Actual position will also be reset 00127 */ 00128 void ParadigmaMonitorData::invalidateHeader() 00129 { 00130 // Invalidate Header information 00131 memset(&m_Header, 0, sizeof(m_Header)); 00132 // Set active data block information to invalid 00133 m_activeDataBlock = (ParadigmaDatasetType_t)Invalid; 00134 // Reset actual position with in Buffer so that next char starts from beginning 00135 m_actualPos = 0; 00136 // Reset the checksum, because it will be calculated on the fly. 00137 m_checksum = 0; 00138 } 00139 00140 /** Function will let user select which temperature to retrieve */ 00141 ParadigmaTemperature ParadigmaMonitorData::getTemperature(ParadigmaTemperatureSelector_t sel) 00142 { 00143 ParadigmaTemperature temp; 00144 switch(sel) { 00145 // Temperatures from Monitor Data 1 00146 case T_aussen : temp = m_Data1.Aussentemp; break; 00147 case T_warm_wasser : temp = m_Data1.Warmwassertemp; break; 00148 case T_kessel_vorlauf : temp = m_Data1.Kesselvorlauf; break; 00149 case T_kessel_ruecklauf : temp = m_Data1.Kesselruecklauf; break; 00150 case T_HK1_raum : temp = m_Data1.RaumtemperaturHK1; break; 00151 case T_HK2_raum : temp = m_Data1.RaumtemperaturHK2; break; 00152 case T_HK1_vorlauf : temp = m_Data1.VorlauftemperaturHK1; break; 00153 case T_HK2_vorlauf : temp = m_Data1.VorlauftemperaturHK2; break; 00154 case T_HK1_ruecklauf : temp = m_Data1.RuecklauftemperaturHK1; break; 00155 case T_HK2_ruecklauf : temp = m_Data1.RuecklauftemperaturHK2; break; 00156 case T_puffer_oben : temp = m_Data1.PuffertemperaturOben; break; 00157 case T_puffer_unten : temp = m_Data1.PuffertemperaturUnten; break; 00158 case T_zirkulation : temp = m_Data1.Zirkulationstemperatur; break; 00159 00160 // Temperatures form Monitor Data 2 00161 case T_HK1_raum_soll : temp = m_Data2.RaumsollHK1; break; 00162 case T_HK2_raum_soll : temp = m_Data2.RaumsollHK2; break; 00163 case T_HK1_vorlauf_soll : temp = m_Data2.VorlaufsollHK1; break; 00164 case T_HK2_vorlauf_soll : temp = m_Data2.VorlaufsollHK2; break; 00165 case T_warm_wasser_soll : temp = m_Data2.Warmwassersolltemp; break; 00166 case T_puffer_soll : temp = m_Data2.Puffersolltemp; break; 00167 00168 default: // Invalid selecion ! 00169 ERR("**** An unknown selection for the temperature was made !"); 00170 break; 00171 } 00172 00173 return temp; 00174 }
Generated on Wed Jul 13 2022 16:47:28 by
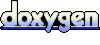