
Project aiming to do a Bluetooth Low Energy IoT devices, which measure temperature and humidity. Bluetooth achieved with the IDB05A1 shield. Temperature/humidity achieved with a DHT11 sensor. Project working, tested on an STM32 L476 board.
Dependencies: BLE_API X_NUCLEO_IDB0XA1 mbed
DHT11.h
00001 #ifndef DHT11_H 00002 #define DHT11_H 00003 00004 //Class to manage DHT11 sensor 00005 class DHT11{ 00006 00007 public: 00008 /* Takes the data pin of the sensor in parameter */ 00009 DHT11(PinName pin):dataPin(pin) 00010 { 00011 iBit = 0; 00012 data = 0; 00013 } 00014 00015 /* Request and receive new data from sensor 00016 Returns : 00017 1 : OK 00018 -1 : Checksum Error 00019 */ 00020 int readData(void){ 00021 this->data = 0; 00022 this->dataPin.output(); 00023 // Request a measurement (low during t > 18ms) 00024 this->dataPin = 0; 00025 wait_ms(20); 00026 this->dataPin = 1; 00027 00028 //Wait for the sensor to take control and set low level /!\ Important 00029 wait_us(20); 00030 00031 this->dataPin.input(); 00032 00033 //TODO Check if timing is correct (low : 80µs ; high 80µs) 00034 // Wait until end of 80µs low 00035 while(!this->dataPin.read()); 00036 // Wait until end of 80 µs high 00037 while(this->dataPin.read()); 00038 00039 // Sensor reply 40bits 00040 for(iBit=0; iBit<40; iBit++) { 00041 this->data = this->data << 1; // Shift for new number 00042 this->timer.stop(); 00043 this->timer.reset(); 00044 00045 // Wait for low level to end 00046 while(!this->dataPin.read()); 00047 this->timer.start(); 00048 // Count time while high level 00049 while(this->dataPin.read()); 00050 00051 if(this->timer.read_us() > 50) 00052 { 00053 //This bit is '1' 00054 this->data++; 00055 } 00056 } 00057 00058 wait_ms(250); 00059 00060 //Checking checksum 00061 if((this->data & 0x00000000000000ff) != ((this->data & 0x000000ff00000000) >> 32) + 00062 ((this->data & 0x00000000ff000000) >> 24) + 00063 ((this->data & 0x0000000000ff0000) >> 16) + 00064 ((this->data & 0x000000000000ff00) >> 8)) 00065 { 00066 return -1; 00067 } 00068 00069 return 1; 00070 } 00071 00072 int getTemperature(void){ 00073 return (int)((this->data & 0x0000000000ff0000) >> 16); 00074 } 00075 00076 int getHumidity(void){ 00077 return (int)((this->data & 0x000000ff00000000) >> 32); 00078 } 00079 00080 00081 private: 00082 DigitalInOut dataPin;//Communication with the sensor 00083 Timer timer; //initialize timer 00084 uint64_t data; // 64 bit variable for temporary data 00085 int iBit; 00086 }; 00087 00088 00089 #endif
Generated on Wed Jul 13 2022 01:51:01 by
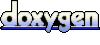