The official mbed C/C SDK provides the software platform and libraries to build your applications.
Fork of mbed by
objects.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_OBJECTS_H 00017 #define MBED_OBJECTS_H 00018 00019 #include "cmsis.h" 00020 #include "PortNames.h" 00021 #include "PeripheralNames.h" 00022 #include "PinNames.h" 00023 00024 #ifdef __cplusplus 00025 extern "C" { 00026 #endif 00027 00028 struct gpio_irq_s { 00029 uint32_t port; 00030 uint32_t pin; 00031 uint32_t ch; 00032 }; 00033 00034 struct port_s { 00035 __IO uint32_t *reg_dir; 00036 __IO uint32_t *reg_out; 00037 __I uint32_t *reg_in; 00038 PortName port; 00039 uint32_t mask; 00040 }; 00041 00042 struct pwmout_s { 00043 __IO uint32_t *MR; 00044 PWMName pwm; 00045 }; 00046 00047 struct serial_s { 00048 LPC_UART_TypeDef *uart; 00049 int index; 00050 }; 00051 00052 struct analogin_s { 00053 ADCName adc; 00054 }; 00055 00056 struct dac_s { 00057 DACName dac; 00058 }; 00059 00060 struct can_s { 00061 LPC_CAN_TypeDef *dev; 00062 }; 00063 00064 struct i2c_s { 00065 LPC_I2C_TypeDef *i2c; 00066 }; 00067 00068 struct spi_s { 00069 LPC_SSP_TypeDef *spi; 00070 }; 00071 00072 #include "gpio_object.h" 00073 00074 #ifdef __cplusplus 00075 } 00076 #endif 00077 00078 #endif
Generated on Tue Jul 12 2022 21:08:34 by
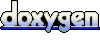