The official mbed C/C SDK provides the software platform and libraries to build your applications.
Fork of mbed by
core_cmInstr.h
00001 /**************************************************************************//** 00002 * @file core_cmInstr.h 00003 * @brief CMSIS Cortex-M Core Instruction Access Header File 00004 * @version V3.03 00005 * @date 29. August 2012 00006 * 00007 * @note 00008 * Copyright (C) 2009-2012 ARM Limited. All rights reserved. 00009 * 00010 * @par 00011 * ARM Limited (ARM) is supplying this software for use with Cortex-M 00012 * processor based microcontrollers. This file can be freely distributed 00013 * within development tools that are supporting such ARM based processors. 00014 * 00015 * @par 00016 * THIS SOFTWARE IS PROVIDED "AS IS". NO WARRANTIES, WHETHER EXPRESS, IMPLIED 00017 * OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. 00019 * ARM SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL, OR 00020 * CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER. 00021 * 00022 ******************************************************************************/ 00023 00024 #ifndef __CORE_CMINSTR_H 00025 #define __CORE_CMINSTR_H 00026 00027 00028 /* ########################## Core Instruction Access ######################### */ 00029 /** \defgroup CMSIS_Core_InstructionInterface CMSIS Core Instruction Interface 00030 Access to dedicated instructions 00031 @{ 00032 */ 00033 00034 #if defined ( __CC_ARM ) /*------------------RealView Compiler -----------------*/ 00035 /* ARM armcc specific functions */ 00036 00037 #if (__ARMCC_VERSION < 400677) 00038 #error "Please use ARM Compiler Toolchain V4.0.677 or later!" 00039 #endif 00040 00041 00042 /** \brief No Operation 00043 00044 No Operation does nothing. This instruction can be used for code alignment purposes. 00045 */ 00046 #define __NOP __nop 00047 00048 00049 /** \brief Wait For Interrupt 00050 00051 Wait For Interrupt is a hint instruction that suspends execution 00052 until one of a number of events occurs. 00053 */ 00054 #define __WFI __wfi 00055 00056 00057 /** \brief Wait For Event 00058 00059 Wait For Event is a hint instruction that permits the processor to enter 00060 a low-power state until one of a number of events occurs. 00061 */ 00062 #define __WFE __wfe 00063 00064 00065 /** \brief Send Event 00066 00067 Send Event is a hint instruction. It causes an event to be signaled to the CPU. 00068 */ 00069 #define __SEV __sev 00070 00071 00072 /** \brief Instruction Synchronization Barrier 00073 00074 Instruction Synchronization Barrier flushes the pipeline in the processor, 00075 so that all instructions following the ISB are fetched from cache or 00076 memory, after the instruction has been completed. 00077 */ 00078 #define __ISB() __isb(0xF) 00079 00080 00081 /** \brief Data Synchronization Barrier 00082 00083 This function acts as a special kind of Data Memory Barrier. 00084 It completes when all explicit memory accesses before this instruction complete. 00085 */ 00086 #define __DSB() __dsb(0xF) 00087 00088 00089 /** \brief Data Memory Barrier 00090 00091 This function ensures the apparent order of the explicit memory operations before 00092 and after the instruction, without ensuring their completion. 00093 */ 00094 #define __DMB() __dmb(0xF) 00095 00096 00097 /** \brief Reverse byte order (32 bit) 00098 00099 This function reverses the byte order in integer value. 00100 00101 \param [in] value Value to reverse 00102 \return Reversed value 00103 */ 00104 #define __REV __rev 00105 00106 00107 /** \brief Reverse byte order (16 bit) 00108 00109 This function reverses the byte order in two unsigned short values. 00110 00111 \param [in] value Value to reverse 00112 \return Reversed value 00113 */ 00114 #ifndef __NO_EMBEDDED_ASM 00115 __attribute__((section(".rev16_text"))) __STATIC_INLINE __ASM uint32_t __REV16(uint32_t value) 00116 { 00117 rev16 r0, r0 00118 bx lr 00119 } 00120 #endif 00121 00122 /** \brief Reverse byte order in signed short value 00123 00124 This function reverses the byte order in a signed short value with sign extension to integer. 00125 00126 \param [in] value Value to reverse 00127 \return Reversed value 00128 */ 00129 #ifndef __NO_EMBEDDED_ASM 00130 __attribute__((section(".revsh_text"))) __STATIC_INLINE __ASM int32_t __REVSH(int32_t value) 00131 { 00132 revsh r0, r0 00133 bx lr 00134 } 00135 #endif 00136 00137 00138 /** \brief Rotate Right in unsigned value (32 bit) 00139 00140 This function Rotate Right (immediate) provides the value of the contents of a register rotated by a variable number of bits. 00141 00142 \param [in] value Value to rotate 00143 \param [in] value Number of Bits to rotate 00144 \return Rotated value 00145 */ 00146 #define __ROR __ror 00147 00148 00149 /** \brief Breakpoint 00150 00151 This function causes the processor to enter Debug state. 00152 Debug tools can use this to investigate system state when the instruction at a particular address is reached. 00153 00154 \param [in] value is ignored by the processor. 00155 If required, a debugger can use it to store additional information about the breakpoint. 00156 */ 00157 #define __BKPT(value) __breakpoint(value) 00158 00159 00160 #if (__CORTEX_M >= 0x03) 00161 00162 /** \brief Reverse bit order of value 00163 00164 This function reverses the bit order of the given value. 00165 00166 \param [in] value Value to reverse 00167 \return Reversed value 00168 */ 00169 #define __RBIT __rbit 00170 00171 00172 /** \brief LDR Exclusive (8 bit) 00173 00174 This function performs a exclusive LDR command for 8 bit value. 00175 00176 \param [in] ptr Pointer to data 00177 \return value of type uint8_t at (*ptr) 00178 */ 00179 #define __LDREXB(ptr) ((uint8_t ) __ldrex(ptr)) 00180 00181 00182 /** \brief LDR Exclusive (16 bit) 00183 00184 This function performs a exclusive LDR command for 16 bit values. 00185 00186 \param [in] ptr Pointer to data 00187 \return value of type uint16_t at (*ptr) 00188 */ 00189 #define __LDREXH(ptr) ((uint16_t) __ldrex(ptr)) 00190 00191 00192 /** \brief LDR Exclusive (32 bit) 00193 00194 This function performs a exclusive LDR command for 32 bit values. 00195 00196 \param [in] ptr Pointer to data 00197 \return value of type uint32_t at (*ptr) 00198 */ 00199 #define __LDREXW(ptr) ((uint32_t ) __ldrex(ptr)) 00200 00201 00202 /** \brief STR Exclusive (8 bit) 00203 00204 This function performs a exclusive STR command for 8 bit values. 00205 00206 \param [in] value Value to store 00207 \param [in] ptr Pointer to location 00208 \return 0 Function succeeded 00209 \return 1 Function failed 00210 */ 00211 #define __STREXB(value, ptr) __strex(value, ptr) 00212 00213 00214 /** \brief STR Exclusive (16 bit) 00215 00216 This function performs a exclusive STR command for 16 bit values. 00217 00218 \param [in] value Value to store 00219 \param [in] ptr Pointer to location 00220 \return 0 Function succeeded 00221 \return 1 Function failed 00222 */ 00223 #define __STREXH(value, ptr) __strex(value, ptr) 00224 00225 00226 /** \brief STR Exclusive (32 bit) 00227 00228 This function performs a exclusive STR command for 32 bit values. 00229 00230 \param [in] value Value to store 00231 \param [in] ptr Pointer to location 00232 \return 0 Function succeeded 00233 \return 1 Function failed 00234 */ 00235 #define __STREXW(value, ptr) __strex(value, ptr) 00236 00237 00238 /** \brief Remove the exclusive lock 00239 00240 This function removes the exclusive lock which is created by LDREX. 00241 00242 */ 00243 #define __CLREX __clrex 00244 00245 00246 /** \brief Signed Saturate 00247 00248 This function saturates a signed value. 00249 00250 \param [in] value Value to be saturated 00251 \param [in] sat Bit position to saturate to (1..32) 00252 \return Saturated value 00253 */ 00254 #define __SSAT __ssat 00255 00256 00257 /** \brief Unsigned Saturate 00258 00259 This function saturates an unsigned value. 00260 00261 \param [in] value Value to be saturated 00262 \param [in] sat Bit position to saturate to (0..31) 00263 \return Saturated value 00264 */ 00265 #define __USAT __usat 00266 00267 00268 /** \brief Count leading zeros 00269 00270 This function counts the number of leading zeros of a data value. 00271 00272 \param [in] value Value to count the leading zeros 00273 \return number of leading zeros in value 00274 */ 00275 #define __CLZ __clz 00276 00277 #endif /* (__CORTEX_M >= 0x03) */ 00278 00279 00280 00281 #elif defined ( __ICCARM__ ) /*------------------ ICC Compiler -------------------*/ 00282 /* IAR iccarm specific functions */ 00283 00284 #include <cmsis_iar.h> 00285 00286 00287 #elif defined ( __TMS470__ ) /*---------------- TI CCS Compiler ------------------*/ 00288 /* TI CCS specific functions */ 00289 00290 #include <cmsis_ccs.h> 00291 00292 00293 #elif defined ( __GNUC__ ) /*------------------ GNU Compiler ---------------------*/ 00294 /* GNU gcc specific functions */ 00295 00296 /** \brief No Operation 00297 00298 No Operation does nothing. This instruction can be used for code alignment purposes. 00299 */ 00300 __attribute__( ( always_inline ) ) __STATIC_INLINE void __NOP(void) 00301 { 00302 __ASM volatile ("nop"); 00303 } 00304 00305 00306 /** \brief Wait For Interrupt 00307 00308 Wait For Interrupt is a hint instruction that suspends execution 00309 until one of a number of events occurs. 00310 */ 00311 __attribute__( ( always_inline ) ) __STATIC_INLINE void __WFI(void) 00312 { 00313 __ASM volatile ("wfi"); 00314 } 00315 00316 00317 /** \brief Wait For Event 00318 00319 Wait For Event is a hint instruction that permits the processor to enter 00320 a low-power state until one of a number of events occurs. 00321 */ 00322 __attribute__( ( always_inline ) ) __STATIC_INLINE void __WFE(void) 00323 { 00324 __ASM volatile ("wfe"); 00325 } 00326 00327 00328 /** \brief Send Event 00329 00330 Send Event is a hint instruction. It causes an event to be signaled to the CPU. 00331 */ 00332 __attribute__( ( always_inline ) ) __STATIC_INLINE void __SEV(void) 00333 { 00334 __ASM volatile ("sev"); 00335 } 00336 00337 00338 /** \brief Instruction Synchronization Barrier 00339 00340 Instruction Synchronization Barrier flushes the pipeline in the processor, 00341 so that all instructions following the ISB are fetched from cache or 00342 memory, after the instruction has been completed. 00343 */ 00344 __attribute__( ( always_inline ) ) __STATIC_INLINE void __ISB(void) 00345 { 00346 __ASM volatile ("isb"); 00347 } 00348 00349 00350 /** \brief Data Synchronization Barrier 00351 00352 This function acts as a special kind of Data Memory Barrier. 00353 It completes when all explicit memory accesses before this instruction complete. 00354 */ 00355 __attribute__( ( always_inline ) ) __STATIC_INLINE void __DSB(void) 00356 { 00357 __ASM volatile ("dsb"); 00358 } 00359 00360 00361 /** \brief Data Memory Barrier 00362 00363 This function ensures the apparent order of the explicit memory operations before 00364 and after the instruction, without ensuring their completion. 00365 */ 00366 __attribute__( ( always_inline ) ) __STATIC_INLINE void __DMB(void) 00367 { 00368 __ASM volatile ("dmb"); 00369 } 00370 00371 00372 /** \brief Reverse byte order (32 bit) 00373 00374 This function reverses the byte order in integer value. 00375 00376 \param [in] value Value to reverse 00377 \return Reversed value 00378 */ 00379 __attribute__( ( always_inline ) ) __STATIC_INLINE uint32_t __REV(uint32_t value) 00380 { 00381 uint32_t result; 00382 00383 __ASM volatile ("rev %0, %1" : "=r" (result) : "r" (value) ); 00384 return(result); 00385 } 00386 00387 00388 /** \brief Reverse byte order (16 bit) 00389 00390 This function reverses the byte order in two unsigned short values. 00391 00392 \param [in] value Value to reverse 00393 \return Reversed value 00394 */ 00395 __attribute__( ( always_inline ) ) __STATIC_INLINE uint32_t __REV16(uint32_t value) 00396 { 00397 uint32_t result; 00398 00399 __ASM volatile ("rev16 %0, %1" : "=r" (result) : "r" (value) ); 00400 return(result); 00401 } 00402 00403 00404 /** \brief Reverse byte order in signed short value 00405 00406 This function reverses the byte order in a signed short value with sign extension to integer. 00407 00408 \param [in] value Value to reverse 00409 \return Reversed value 00410 */ 00411 __attribute__( ( always_inline ) ) __STATIC_INLINE int32_t __REVSH(int32_t value) 00412 { 00413 uint32_t result; 00414 00415 __ASM volatile ("revsh %0, %1" : "=r" (result) : "r" (value) ); 00416 return(result); 00417 } 00418 00419 00420 /** \brief Rotate Right in unsigned value (32 bit) 00421 00422 This function Rotate Right (immediate) provides the value of the contents of a register rotated by a variable number of bits. 00423 00424 \param [in] value Value to rotate 00425 \param [in] value Number of Bits to rotate 00426 \return Rotated value 00427 */ 00428 __attribute__( ( always_inline ) ) __STATIC_INLINE uint32_t __ROR(uint32_t op1, uint32_t op2) 00429 { 00430 00431 __ASM volatile ("ror %0, %0, %1" : "+r" (op1) : "r" (op2) ); 00432 return(op1); 00433 } 00434 00435 00436 /** \brief Breakpoint 00437 00438 This function causes the processor to enter Debug state. 00439 Debug tools can use this to investigate system state when the instruction at a particular address is reached. 00440 00441 \param [in] value is ignored by the processor. 00442 If required, a debugger can use it to store additional information about the breakpoint. 00443 */ 00444 #define __BKPT(value) __ASM volatile ("bkpt "#value) 00445 00446 00447 #if (__CORTEX_M >= 0x03) 00448 00449 /** \brief Reverse bit order of value 00450 00451 This function reverses the bit order of the given value. 00452 00453 \param [in] value Value to reverse 00454 \return Reversed value 00455 */ 00456 __attribute__( ( always_inline ) ) __STATIC_INLINE uint32_t __RBIT(uint32_t value) 00457 { 00458 uint32_t result; 00459 00460 __ASM volatile ("rbit %0, %1" : "=r" (result) : "r" (value) ); 00461 return(result); 00462 } 00463 00464 00465 /** \brief LDR Exclusive (8 bit) 00466 00467 This function performs a exclusive LDR command for 8 bit value. 00468 00469 \param [in] ptr Pointer to data 00470 \return value of type uint8_t at (*ptr) 00471 */ 00472 __attribute__( ( always_inline ) ) __STATIC_INLINE uint8_t __LDREXB(volatile uint8_t *addr) 00473 { 00474 uint8_t result; 00475 00476 __ASM volatile ("ldrexb %0, [%1]" : "=r" (result) : "r" (addr) ); 00477 return(result); 00478 } 00479 00480 00481 /** \brief LDR Exclusive (16 bit) 00482 00483 This function performs a exclusive LDR command for 16 bit values. 00484 00485 \param [in] ptr Pointer to data 00486 \return value of type uint16_t at (*ptr) 00487 */ 00488 __attribute__( ( always_inline ) ) __STATIC_INLINE uint16_t __LDREXH(volatile uint16_t *addr) 00489 { 00490 uint16_t result; 00491 00492 __ASM volatile ("ldrexh %0, [%1]" : "=r" (result) : "r" (addr) ); 00493 return(result); 00494 } 00495 00496 00497 /** \brief LDR Exclusive (32 bit) 00498 00499 This function performs a exclusive LDR command for 32 bit values. 00500 00501 \param [in] ptr Pointer to data 00502 \return value of type uint32_t at (*ptr) 00503 */ 00504 __attribute__( ( always_inline ) ) __STATIC_INLINE uint32_t __LDREXW(volatile uint32_t *addr) 00505 { 00506 uint32_t result; 00507 00508 __ASM volatile ("ldrex %0, [%1]" : "=r" (result) : "r" (addr) ); 00509 return(result); 00510 } 00511 00512 00513 /** \brief STR Exclusive (8 bit) 00514 00515 This function performs a exclusive STR command for 8 bit values. 00516 00517 \param [in] value Value to store 00518 \param [in] ptr Pointer to location 00519 \return 0 Function succeeded 00520 \return 1 Function failed 00521 */ 00522 __attribute__( ( always_inline ) ) __STATIC_INLINE uint32_t __STREXB(uint8_t value, volatile uint8_t *addr) 00523 { 00524 uint32_t result; 00525 00526 __ASM volatile ("strexb %0, %2, [%1]" : "=&r" (result) : "r" (addr), "r" (value) ); 00527 return(result); 00528 } 00529 00530 00531 /** \brief STR Exclusive (16 bit) 00532 00533 This function performs a exclusive STR command for 16 bit values. 00534 00535 \param [in] value Value to store 00536 \param [in] ptr Pointer to location 00537 \return 0 Function succeeded 00538 \return 1 Function failed 00539 */ 00540 __attribute__( ( always_inline ) ) __STATIC_INLINE uint32_t __STREXH(uint16_t value, volatile uint16_t *addr) 00541 { 00542 uint32_t result; 00543 00544 __ASM volatile ("strexh %0, %2, [%1]" : "=&r" (result) : "r" (addr), "r" (value) ); 00545 return(result); 00546 } 00547 00548 00549 /** \brief STR Exclusive (32 bit) 00550 00551 This function performs a exclusive STR command for 32 bit values. 00552 00553 \param [in] value Value to store 00554 \param [in] ptr Pointer to location 00555 \return 0 Function succeeded 00556 \return 1 Function failed 00557 */ 00558 __attribute__( ( always_inline ) ) __STATIC_INLINE uint32_t __STREXW(uint32_t value, volatile uint32_t *addr) 00559 { 00560 uint32_t result; 00561 00562 __ASM volatile ("strex %0, %2, [%1]" : "=&r" (result) : "r" (addr), "r" (value) ); 00563 return(result); 00564 } 00565 00566 00567 /** \brief Remove the exclusive lock 00568 00569 This function removes the exclusive lock which is created by LDREX. 00570 00571 */ 00572 __attribute__( ( always_inline ) ) __STATIC_INLINE void __CLREX(void) 00573 { 00574 __ASM volatile ("clrex"); 00575 } 00576 00577 00578 /** \brief Signed Saturate 00579 00580 This function saturates a signed value. 00581 00582 \param [in] value Value to be saturated 00583 \param [in] sat Bit position to saturate to (1..32) 00584 \return Saturated value 00585 */ 00586 #define __SSAT(ARG1,ARG2) \ 00587 ({ \ 00588 uint32_t __RES, __ARG1 = (ARG1); \ 00589 __ASM ("ssat %0, %1, %2" : "=r" (__RES) : "I" (ARG2), "r" (__ARG1) ); \ 00590 __RES; \ 00591 }) 00592 00593 00594 /** \brief Unsigned Saturate 00595 00596 This function saturates an unsigned value. 00597 00598 \param [in] value Value to be saturated 00599 \param [in] sat Bit position to saturate to (0..31) 00600 \return Saturated value 00601 */ 00602 #define __USAT(ARG1,ARG2) \ 00603 ({ \ 00604 uint32_t __RES, __ARG1 = (ARG1); \ 00605 __ASM ("usat %0, %1, %2" : "=r" (__RES) : "I" (ARG2), "r" (__ARG1) ); \ 00606 __RES; \ 00607 }) 00608 00609 00610 /** \brief Count leading zeros 00611 00612 This function counts the number of leading zeros of a data value. 00613 00614 \param [in] value Value to count the leading zeros 00615 \return number of leading zeros in value 00616 */ 00617 __attribute__( ( always_inline ) ) __STATIC_INLINE uint8_t __CLZ(uint32_t value) 00618 { 00619 uint8_t result; 00620 00621 __ASM volatile ("clz %0, %1" : "=r" (result) : "r" (value) ); 00622 return(result); 00623 } 00624 00625 #endif /* (__CORTEX_M >= 0x03) */ 00626 00627 00628 00629 00630 #elif defined ( __TASKING__ ) /*------------------ TASKING Compiler --------------*/ 00631 /* TASKING carm specific functions */ 00632 00633 /* 00634 * The CMSIS functions have been implemented as intrinsics in the compiler. 00635 * Please use "carm -?i" to get an up to date list of all intrinsics, 00636 * Including the CMSIS ones. 00637 */ 00638 00639 #endif 00640 00641 /*@}*/ /* end of group CMSIS_Core_InstructionInterface */ 00642 00643 #endif /* __CORE_CMINSTR_H */
Generated on Tue Jul 12 2022 21:08:34 by
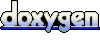