The official mbed C/C SDK provides the software platform and libraries to build your applications.
Fork of mbed by
PeripheralNames.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_PERIPHERALNAMES_H 00017 #define MBED_PERIPHERALNAMES_H 00018 00019 #include "cmsis.h" 00020 00021 #ifdef __cplusplus 00022 extern "C" { 00023 #endif 00024 00025 typedef enum { 00026 UART_0 = (int)LPC_UART0_BASE, 00027 UART_1 = (int)LPC_UART1_BASE, 00028 UART_2 = (int)LPC_UART2_BASE, 00029 UART_3 = (int)LPC_UART3_BASE 00030 } UARTName; 00031 00032 typedef enum { 00033 ADC0_0 = 0, 00034 ADC0_1, 00035 ADC0_2, 00036 ADC0_3, 00037 ADC0_4, 00038 ADC0_5, 00039 ADC0_6, 00040 ADC0_7 00041 } ADCName; 00042 00043 typedef enum { 00044 DAC_0 = 0 00045 } DACName; 00046 00047 typedef enum { 00048 SPI_0 = (int)LPC_SSP0_BASE, 00049 SPI_1 = (int)LPC_SSP1_BASE 00050 } SPIName; 00051 00052 typedef enum { 00053 I2C_0 = (int)LPC_I2C0_BASE, 00054 I2C_1 = (int)LPC_I2C1_BASE, 00055 I2C_2 = (int)LPC_I2C2_BASE 00056 } I2CName; 00057 00058 typedef enum { 00059 PWM_1 = 1, 00060 PWM_2, 00061 PWM_3, 00062 PWM_4, 00063 PWM_5, 00064 PWM_6 00065 } PWMName; 00066 00067 typedef enum { 00068 CAN_1 = (int)LPC_CAN1_BASE, 00069 CAN_2 = (int)LPC_CAN2_BASE 00070 } CANName; 00071 00072 #define STDIO_UART_TX USBTX 00073 #define STDIO_UART_RX USBRX 00074 #define STDIO_UART UART_0 00075 00076 #ifdef __cplusplus 00077 } 00078 #endif 00079 00080 #endif
Generated on Tue Jul 12 2022 21:08:34 by
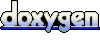