
this is a test code for Mbed_Shield_GPRS_Call_Up_Test
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 Serial gprs(p28,p27); //Hardware Serial 00004 //Serial gprs(p9,p10); //Software Serial 00005 Serial pc(USBTX,USBRX); 00006 00007 Timer timeCnt; 00008 00009 int waitForResp(char *resp, int timeout) 00010 { 00011 int len = strlen(resp); 00012 int sum=0; 00013 timeCnt.start(); 00014 00015 while(1) { 00016 if(gprs.readable()) { 00017 char c = gprs.getc(); 00018 sum = (c==resp[sum]) ? sum+1 : 0; 00019 if(sum == len)break; 00020 } 00021 if(timeCnt.read() > timeout) { // time out 00022 timeCnt.stop(); 00023 timeCnt.reset(); 00024 pc.printf("Error:time out"); 00025 return -1; 00026 } 00027 } 00028 timeCnt.stop(); // stop timer 00029 timeCnt.reset(); // clear timer 00030 while(gprs.readable()) { // display the other thing.. 00031 char c = gprs.getc(); 00032 } 00033 00034 return 0; 00035 } 00036 00037 int sendCmdAndWaitForResp(char *cmd, char *resp, int timeout) 00038 { 00039 gprs.puts(cmd); 00040 return waitForResp(resp,timeout); 00041 } 00042 00043 int callUp(char *number) 00044 { 00045 if(0 != sendCmdAndWaitForResp("AT+COLP=1\r\n","OK",5)) { 00046 pc.printf("Error:COLP"); 00047 return -1; 00048 } 00049 wait(1); 00050 gprs.printf("\r\nATD%s;\r\n",number); 00051 return 0; 00052 } 00053 00054 int main() 00055 { 00056 pc.baud(19200); 00057 gprs.baud(19200); 00058 00059 for(int i = 0; i < 5; i++) { 00060 wait(1); 00061 printf("wait\n"); 00062 } 00063 callUp("139****7382"); 00064 while(1); 00065 }
Generated on Tue Jul 19 2022 16:44:52 by
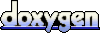