
seeedstudio ARCH GPRS Test for GPRS function
Fork of ARCH_GPRS_Demo by
main.cpp
00001 /* 00002 main.cpp 00003 2013 Copyright (c) Seeed Technology Inc. All right reserved. 00004 00005 Author:lawliet zou(lawliet.zou@gmail.com) 00006 2013-7-21 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General Public 00019 License along with this library; if not, write to the Free Software 00020 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00021 */ 00022 #include "mbed.h" 00023 #include "string.h" 00024 #include "gprs.h" 00025 00026 #define SEND_SMS_TEST 0 00027 #define CALL_UP_TEST 0 00028 #define ANSWER_TEST 0 00029 #define READ_SMS_TEST 0 00030 00031 #define PHONE_NUMBER "150****9566" 00032 00033 #define PINPWR P1_2 // power on EG 10, low enable 00034 #define PINONOFF P1_7 // switch of EG10, low enable, low for 2s to turn on EG10 00035 00036 DigitalOut eg10_pwr(PINPWR); 00037 DigitalOut eg10_on(PINONOFF); 00038 GPRS gprsTest(P0_19, P0_18, 115200, PHONE_NUMBER); 00039 00040 void EG10_PowerUp(void) 00041 { 00042 eg10_pwr = 1; 00043 eg10_on = 1; 00044 wait(2); 00045 eg10_pwr = 0; 00046 eg10_on = 0; 00047 wait(2); 00048 eg10_on = 1; 00049 wait(2); 00050 } 00051 00052 int main(void) 00053 { 00054 EG10_PowerUp(); 00055 while(0 != gprsTest.init()) { 00056 wait(2); 00057 } 00058 00059 #if CALL_UP_TEST 00060 gprsTest.callUp(PHONE_NUMBER); 00061 #endif 00062 00063 #if SEND_SMS_TEST 00064 gprsTest.sendSMS(PHONE_NUMBER,"hello,lawliet"); 00065 #endif 00066 00067 #if ANSWER_TEST || READ_SMS_TEST 00068 while(1) { 00069 int messageType = gprsTest.loopHandle(); 00070 if(MESSAGE_RING == messageType) { 00071 gprsTest.answer(); 00072 } else if(MESSAGE_SMS == messageType) { 00073 char smsMessage[SMS_MAX_LENGTH]; 00074 gprsTest.getSMS(smsMessage); 00075 } 00076 } 00077 #endif 00078 00079 return 0; 00080 }
Generated on Sat Jul 23 2022 19:09:22 by
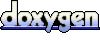