This fork re-enables FRDM boards and adds WebUSB CDC functionality
Fork of USBDevice_STM32F103 by
USBDFU.h
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef USB_DFU_H 00020 #define USB_DFU_H 00021 00022 /* These headers are included for child class. */ 00023 #include "USBEndpoints.h" 00024 #include "USBDescriptor.h" 00025 #include "USBDevice_Types.h" 00026 00027 #include "USBDevice.h" 00028 00029 #include "Callback.h" 00030 00031 00032 class USBDFU: public USBDevice { 00033 public: 00034 00035 /** 00036 * Constructor 00037 * 00038 * @param vendor_id Your vendor_id 00039 * @param product_id Your product_id 00040 * @param product_release Your product_release 00041 * @param connect Connect the device 00042 */ 00043 USBDFU(uint16_t vendor_id = 0x1234, uint16_t product_id = 0x0006, uint16_t product_release = 0x0001, bool connect = true); 00044 00045 /** 00046 * Attach a callback called when a DFU detach request is received 00047 * 00048 * @param tptr pointer to the object to call the member function on 00049 * @param mptr pointer to the member function to be called 00050 */ 00051 template<typename T> 00052 void attach(T* tptr, void (T::*mptr)(void)) { 00053 if((mptr != NULL) && (tptr != NULL)) { 00054 detach.attach(tptr, mptr); 00055 } 00056 } 00057 00058 /** 00059 * Attach a callback called when a DFU detach request is received 00060 * 00061 * @param func function pointer 00062 */ 00063 void attach(Callback<void()> func); 00064 00065 protected: 00066 uint16_t reportLength; 00067 00068 /* 00069 * Get string product descriptor 00070 * 00071 * @returns pointer to the string product descriptor 00072 */ 00073 virtual uint8_t * stringIproductDesc(); 00074 00075 /* 00076 * Get string interface descriptor 00077 * 00078 * @returns pointer to the string interface descriptor 00079 */ 00080 virtual uint8_t * stringIinterfaceDesc(); 00081 00082 /* 00083 * Get configuration descriptor 00084 * 00085 * @returns pointer to the configuration descriptor 00086 */ 00087 virtual uint8_t * configurationDesc(); 00088 00089 /* 00090 * Called by USBDevice on Endpoint0 request. Warning: Called in ISR context 00091 * This is used to handle extensions to standard requests 00092 * and class specific requests 00093 * 00094 * @returns true if class handles this request 00095 */ 00096 virtual bool USBCallback_request(); 00097 00098 00099 /* 00100 * Called by USBDevice layer. Set configuration of the device. 00101 * For instance, you can add all endpoints that you need on this function. 00102 * 00103 * @param configuration Number of the configuration 00104 * @returns true if class handles this request 00105 */ 00106 virtual bool USBCallback_setConfiguration(uint8_t configuration); 00107 00108 private: 00109 Callback<void()> detach; 00110 00111 static void no_op() {}; 00112 }; 00113 00114 #endif
Generated on Tue Jul 12 2022 21:49:58 by
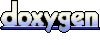