
Prototype program for balancing robot based on various examples from other users.
Dependencies: HCSR04 MPU6050_1 Motor Servo ledControl2 mbed
HALLFX_ENCODER.h
00001 #ifndef HALLFX_ENCODER_H 00002 #define HALLFX_ENCODER_H 00003 00004 /* 00005 Basic Encoder Library for Sparkfun's Wheel Encoder Kit 00006 Part# ROB-12629. 00007 */ 00008 00009 #include "mbed.h" 00010 00011 class HALLFX_ENCODER{ 00012 public: 00013 /* 00014 Constructor for Encoder objects 00015 @param enc_in The mBed pin connected to encoder output 00016 */ 00017 HALLFX_ENCODER(PinName enc_in); 00018 /* 00019 read() returns total number of counts of the encoder. 00020 Count can be +/- and indicates the overall direction, 00021 (+): CW (-): CCW 00022 @return The toltal number of counts of the encoder. 00023 */ 00024 long read(); 00025 /* 00026 reset() clears the counter to 0. 00027 */ 00028 void reset(); 00029 private: 00030 long count; // Total number of counts since start. 00031 InterruptIn _enc_in;// Encoder Input/Interrupt Pin 00032 /* 00033 Increments/Decrements count on interrrupt. 00034 */ 00035 void callback(); // Interrupt callback function 00036 }; 00037 00038 #endif
Generated on Sun Aug 21 2022 21:37:40 by
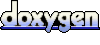