A library for AQM0802A I2C connecting LCD module.
Embed:
(wiki syntax)
Show/hide line numbers
KuAQM0802A.cpp
00001 #include "KuAQM0802A.h" 00002 00003 const int KU_AQM0802A_I2C_ADDR = 0x3E << 1; // 0x7C 00004 const int KU_AQM0802A_INITIAL_CONTRAST = 35; 00005 00006 KuAQM0802A::KuAQM0802A(I2C &i2c_) : i2c(i2c_) 00007 { 00008 reset(); 00009 } 00010 00011 KuAQM0802A::~KuAQM0802A() 00012 { 00013 } 00014 00015 /** 00016 * Reset AQM0802A LCD 00017 */ 00018 void KuAQM0802A::reset() { 00019 // wait 40 msec 00020 wait(0.04); 00021 // Function set (to Normal Mode) 00022 send_cmd(0x38); 00023 // Function set (to Extention Mode) 00024 send_cmd(0x39); 00025 // EntryMode (Left to Right, No Shift) 00026 send_cmd(0x04); 00027 // Interval OSC frequency 00028 send_cmd(0x14); 00029 // Contrast (Low bits) 00030 send_cmd(0x70 | (KU_AQM0802A_INITIAL_CONTRAST & 0xF)); 00031 // Icon on (0x08) / Booster circuit on (0x04) / Contrast (High bits) 00032 send_cmd(0x50 | 0x08 | 0x04 | ((KU_AQM0802A_INITIAL_CONTRAST >> 4) & 0x3)); 00033 // Follower control 00034 send_cmd(0x6C); 00035 wait(0.2); 00036 // Function set (to Normal Mode) 00037 send_cmd(0x38); 00038 // Display On 00039 send_cmd(0x0C); 00040 // Clear Display 00041 send_cmd(0x01); 00042 wait(0.2); 00043 } 00044 00045 /** 00046 * Locate cursor 00047 */ 00048 void KuAQM0802A::locate(unsigned int x, unsigned int y) { 00049 send_cmd(0x80 | (y * 0x40 + x)); 00050 } 00051 00052 /** 00053 * Print a character 00054 */ 00055 void KuAQM0802A::print_char(const int character) { 00056 send(false, true, character); 00057 } 00058 00059 /** 00060 * Change LCD contrast (0-63) 00061 */ 00062 void KuAQM0802A::set_contrast(unsigned int c) { 00063 send_cmd(0x39); 00064 send_cmd(0x70 | (c & 0xF)); // Contrast Set (Low bits) 00065 send_cmd(0x50 | 0x08 | 0x40 | ((c >> 4) & 0x3)); // Icon on / Booster circuit on / Contrast Set (High bits) 00066 send_cmd(0x38); 00067 } 00068 00069 /** 00070 * Send an AQM0802A command 00071 */ 00072 void KuAQM0802A::send_cmd(char cmd) { 00073 send(false, false, cmd); 00074 } 00075 00076 /** 00077 * Send raw code. 00078 */ 00079 void KuAQM0802A::send(bool CO, bool RS, char code) { 00080 char data[2]; 00081 char CObit = CO ? 0x80 : 0x00; 00082 char RSbit = RS ? 0x40 : 0x00; 00083 data[0] = CObit | RSbit; 00084 data[1] = code; 00085 i2c.write(KU_AQM0802A_I2C_ADDR, data, 2); 00086 }
Generated on Wed Jul 13 2022 00:16:57 by
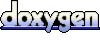