Simple test app to run a NeoStrip connected to a nRF51 Dongle
Dependencies: RedBearNano_NeoPixels
main.cpp
00001 #include "mbed.h" 00002 00003 #include "neopixel.h" 00004 00005 #define NRF51_DONGLE 00006 00007 #define LED_COUNT 15 00008 00009 #ifdef NRF51_DONGLE 00010 #define LED_0 p21 00011 #define LED_1 p23 00012 #define LED_2 p22 00013 00014 #define STRIP_0 p15 00015 #define STRIP_1 p16 00016 00017 #define PULSE_0 p17 00018 00019 #elif NRF51_MICROBIT 00020 #define LED_0 p18 00021 #define LED_1 p19 00022 #endif 00023 00024 DigitalOut myled_0(LED_0); 00025 DigitalOut myled_1(LED_1); 00026 00027 DigitalOut myledError(LED_2); 00028 00029 neopixel_strip_t m_strip; 00030 uint8_t dig_pin_num = 15; 00031 uint8_t leds_per_strip = 24; 00032 uint8_t result; 00033 00034 uint8_t current = 0; 00035 00036 //clear and remove strip 00037 // neopixel_clear(&m_strip); 00038 // neopixel_destroy(&m_strip); 00039 00040 void renderLine() 00041 { 00042 result = neopixel_set_color_and_show(&m_strip, current, 0x22, 0xAA, 0x88); 00043 00044 current = (current + 1) % LED_COUNT; 00045 00046 if (result) { 00047 myledError = 1; 00048 } 00049 else { 00050 myledError = 0; 00051 } 00052 } 00053 00054 int main () 00055 { 00056 neopixel_init(&m_strip, STRIP_0, LED_COUNT); 00057 neopixel_clear(&m_strip); 00058 00059 while (1) { 00060 myled_0 = 1; 00061 myled_1 = 0; 00062 wait (0.2); 00063 00064 myled_0 = 0; 00065 myled_1 = 1; 00066 wait (0.2); 00067 00068 renderLine(); 00069 } 00070 }
Generated on Mon Aug 8 2022 16:32:35 by
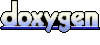