To call Azure Marketplace Translation (and Speech) service
Dependencies: EthernetInterface-FRDM HTTPClient-SSL R_BSP SDFileSystem TLV320_RBSP USBHost mbed-rtos mbed-src
wavecnv.cpp
00001 //////////////////////////////////////////////////////////////////////////// 00002 // Licensed under the Apache License, Version 2.0 (the "License"); 00003 // you may not use this file except in compliance with the License. 00004 // You may obtain a copy of the License at 00005 // 00006 // http://www.apache.org/licenses/LICENSE-2.0 00007 // 00008 // Unless required by applicable law or agreed to in writing, software 00009 // distributed under the License is distributed on an "AS IS" BASIS, 00010 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00011 // See the License for the specific language governing permissions and 00012 // limitations under the License. 00013 // 00014 // Copyright (c) Microsoft Corporation. All rights reserved. 00015 // Portions Copyright (c) Kentaro Sekimoto All rights reserved. 00016 // 00017 // Convert wave file from 8bit mono to 16 bit stereo 00018 // 00019 //////////////////////////////////////////////////////////////////////////// 00020 00021 #include "mbed.h" 00022 00023 #define HEADER_SIZE 0x2e 00024 #define BUF_SIZE 1024 00025 #define TO_BUF_SIZE (FROM_BUF_SIZE * 2) 00026 #define MUL 20 00027 00028 unsigned char fromHeader[HEADER_SIZE]; 00029 unsigned char toHeader[HEADER_SIZE]; 00030 00031 unsigned char fromBuf[BUF_SIZE]; 00032 short toBuf[BUF_SIZE*2]; 00033 00034 // 0x25c8 + 0x2e = 0x25f6 00035 void wavecnv(char *fromfn, char *tofn) 00036 { 00037 FILE *fromfp; 00038 FILE *tofp; 00039 int fromSize; 00040 int toSize; 00041 unsigned int size; 00042 int i; 00043 unsigned int fromChunkSize; 00044 unsigned int toChunkSize; 00045 unsigned int sampleRate; 00046 unsigned short uval; 00047 short sval; 00048 if ((fromfp = fopen(fromfn, "rb")) == NULL) 00049 return; 00050 if ((tofp = fopen(tofn, "wb")) == NULL) 00051 return; 00052 fromSize = fread(fromHeader, 1, HEADER_SIZE, fromfp); 00053 memcpy(toHeader, fromHeader, HEADER_SIZE); 00054 sampleRate = (unsigned int)fromHeader[0x18] + 00055 ((unsigned int)fromHeader[0x19] << 8) + 00056 ((unsigned int)fromHeader[0x1a] << 16) + 00057 ((unsigned int)fromHeader[0x1b] << 24) ; 00058 sampleRate *= 4; 00059 toHeader[0x1c] = (unsigned char)(sampleRate & 0xff); 00060 toHeader[0x1d] = (unsigned char)((sampleRate >> 8) & 0xff); 00061 toHeader[0x1e] = (unsigned char)((sampleRate >> 16) & 0xff); 00062 toHeader[0x1f] = (unsigned char)((sampleRate >> 24) & 0xff); 00063 toHeader[0x10] = 0x10; 00064 toHeader[0x16] = 0x02; 00065 toHeader[0x20] = 0x04; 00066 toHeader[0x22] = 0x10; 00067 fromChunkSize = (unsigned int)fromHeader[0x2a] + 00068 ((unsigned int)fromHeader[0x2b] << 8) + 00069 ((unsigned int)fromHeader[0x2c] << 16) + 00070 ((unsigned int)fromHeader[0x2d] << 24) ; 00071 toChunkSize = fromChunkSize * 4; 00072 toHeader[0x24] = 'd'; 00073 toHeader[0x25] = 'a'; 00074 toHeader[0x26] = 't'; 00075 toHeader[0x27] = 'a'; 00076 toHeader[0x28] = (unsigned char)(toChunkSize & 0xff); 00077 toHeader[0x29] = (unsigned char)((toChunkSize >> 8) & 0xff); 00078 toHeader[0x2a] = (unsigned char)((toChunkSize >> 16) & 0xff); 00079 toHeader[0x2b] = (unsigned char)((toChunkSize >> 24) & 0xff); 00080 size = toChunkSize + 0x2c - 8; 00081 toHeader[0x04] = (unsigned char)(size & 0xff); 00082 toHeader[0x05] = (unsigned char)((size >> 8) & 0xff); 00083 toHeader[0x06] = (unsigned char)((size >> 16) & 0xff); 00084 toHeader[0x07] = (unsigned char)((size >> 24) & 0xff); 00085 toSize = fwrite(toHeader, 1, HEADER_SIZE, tofp); 00086 while (fromChunkSize > 0) { 00087 if (fromChunkSize > BUF_SIZE) 00088 size = BUF_SIZE; 00089 else 00090 size = fromChunkSize; 00091 fromSize = fread(fromBuf, 1, size, fromfp); 00092 for (i = 0; i < size; i++) { 00093 if (fromBuf[i] == 0x80) { 00094 toBuf[i*2] = 0; 00095 toBuf[i*2+1] = 0; 00096 } else { 00097 uval = (unsigned short)fromBuf[i]; 00098 if (uval > 0x80) { 00099 uval = (uval - 0x80) * 256; 00100 sval = (short)uval; 00101 } else { 00102 uval = (0x80 - uval) * 256; 00103 sval = ((short)uval) * (-1); 00104 } 00105 toBuf[i*2] = sval; 00106 toBuf[i*2+1] = sval; 00107 } 00108 } 00109 toSize = fwrite(toBuf, 4, size, tofp); 00110 fromChunkSize -= size; 00111 } 00112 fclose(tofp); 00113 fclose(fromfp); 00114 }
Generated on Fri Jul 15 2022 04:07:23 by
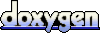