Simple 6-LED bar library. Provides some useful functions.
Embed:
(wiki syntax)
Show/hide line numbers
6LedBar.h
00001 #ifndef LED_BAR_H 00002 #define LED_BAR_H 00003 00004 /* * * * * * * * * * * * * * * * * * * * * * * * 00005 00006 Simple 6-LEDs bar library for using with MBed 2.0 library 00007 00008 Copyright 2017 Dmitry Makarenko 00009 00010 Licensed under the Apache License, Version 2.0 (the "License"); 00011 you may not use this file except in compliance with the License. 00012 You may obtain a copy of the License at 00013 00014 http://www.apache.org/licenses/LICENSE-2.0 00015 00016 Unless required by applicable law or agreed to in writing, software 00017 distributed under the License is distributed on an "AS IS" BASIS, 00018 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 See the License for the specific language governing permissions and 00020 limitations under the License. 00021 00022 * * * * * * * * * * * * * * * * * * * * * * * * */ 00023 00024 #include "mbed.h" 00025 00026 00027 00028 /** 00029 * LedBar class is used for controlling 6 led bar. 00030 * 00031 * Example using on, off, toggle methods: 00032 * 00033 * @code 00034 * 00035 * #include "mbed.h" 00036 * #include "6LedBar.h" 00037 * 00038 * 00039 * LedBar leds (A0, A1, A2, A3, A4, A5); 00040 * 00041 * int main() { 00042 * leds.on(0); 00043 * leds.on(2); 00044 * leds.on(4); 00045 * 00046 * while(true) { 00047 * for(int i = 0; i < 6; i++) { 00048 * leds.toggle(i); 00049 * } 00050 * wait(0.5); 00051 * } 00052 * } 00053 * 00054 * 00055 * @endcode 00056 * 00057 * * * * * * * * * * * * * * * * * * * * * * * * * 00058 * 00059 * Example using mask methods: 00060 * 00061 * @code 00062 * 00063 * #include "mbed.h" 00064 * #include "6LedBar.h" 00065 * 00066 * 00067 * LedBar leds (A0, A1, A2, A3, A4, A5); 00068 * 00069 * int main() { 00070 * 00071 * leds.set_mask(0b010101); 00072 * 00073 * while(true) { 00074 * leds.toggle_mask(0b111111); 00075 * wait(0.5); 00076 * } 00077 * } 00078 * 00079 * @endcode 00080 * 00081 **/ 00082 00083 //! 00084 class LedBar { 00085 00086 public: 00087 00088 //Constructor, you need to specify pins to which 00089 //leds are connected 00090 LedBar(PinName p0, 00091 PinName p1, 00092 PinName p2, 00093 PinName p3, 00094 PinName p4, 00095 PinName p5); 00096 00097 //! Turn n-s led on 00098 void on(int n); 00099 00100 //! Turn n-s led off 00101 void off(int n); 00102 00103 //! Toggle n-s led 00104 void toggle(int n); 00105 00106 //! Set bit mask 00107 //i.e. mask 0b000101 will turn on leds 0 and 1, and off all others 00108 void set_mask(int m); 00109 00110 //! Turn on leds specified by bit-mask 00111 void on_mask(int m); 00112 00113 //! Turn off leds specified by bit-mask 00114 void off_mask(int m); 00115 00116 //! Toggle leds specified by bit-mask 00117 void toggle_mask(int m); 00118 private: 00119 static const int LEDS_COUNT = 6; 00120 DigitalOut l0, l1, l2, l3, l4, l5; 00121 DigitalOut *m_leds[LEDS_COUNT]; 00122 00123 }; 00124 00125 00126 #endif //LED_BAR_H
Generated on Tue Jul 12 2022 15:23:02 by
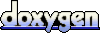