An API for interacting with the Adafruit flow meter.
Embed:
(wiki syntax)
Show/hide line numbers
FlowMeter.cpp
00001 #include "FlowMeter.h" 00002 00003 void FlowMeter::handle_pulse_irq(void) 00004 { 00005 _pulses++; 00006 } 00007 00008 FlowMeter::FlowMeter(PinName out) : _pin(out) 00009 { 00010 _pulses = 0; 00011 _pin.rise(this, &FlowMeter::handle_pulse_irq); 00012 00013 } 00014 00015 long FlowMeter::get_pulse_count(void) 00016 { 00017 return _pulses; 00018 } 00019 00020 float FlowMeter::get_fl_oz(void) 00021 { 00022 return pulses_to_fl_oz(_pulses); 00023 } 00024 00025 float FlowMeter::get_ml(void) 00026 { 00027 return pulses_to_ml(_pulses); 00028 } 00029 00030 float FlowMeter::pulses_to_ml(int pulses) 00031 { 00032 return ((float)pulses) / 2.2222222f; // pulses per mL. 00033 } 00034 00035 float FlowMeter::pulses_to_fl_oz(int pulses) 00036 { 00037 return ((float)pulses) / 13.308294f; // pulses per fl oz. 00038 } 00039 00040 00041 void FlowMeter::reset(void) 00042 { 00043 _pulses = 0; 00044 }
Generated on Wed Jul 13 2022 12:28:59 by
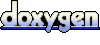