
Initial commit
Dependencies: ConfigFile FXOS8700CQ M2XStreamClient-JMF MODSERIAL SDFileSystem WNCInterface jsonlite mbed-rtos mbed
Fork of StarterKit_M2X_DevLab by
config_me.h
00001 const char* hStreamName = "humidity"; // Humidity stream ID 00002 const char* tStreamName = "temp"; // Temperature stream ID 00003 const char* accelStreamNames[] = { "accelX", "accelY", "accelZ" }; // Accelerometer stream IDs 00004 00005 class Configuration { 00006 public: 00007 char deviceId[33], // Device you want to post to 00008 m2xKey[33], // Your M2X API Key or Master API Key 00009 udpHost[30], 00010 devIMEI[16]; 00011 00012 bool enableM2X, 00013 enableCommands, 00014 enableUDP, 00015 m2xConfigured; 00016 00017 int commandDelay, // how many seconds we should wait between polling for a command 00018 commandPolls, // how many times we should poll for a command 00019 udpPort; 00020 00021 double locLat, 00022 locLong; 00023 00024 Configuration(): deviceId(""), 00025 m2xKey(""), 00026 enableM2X(true), 00027 enableCommands(false), 00028 enableUDP(false), 00029 commandDelay(10), 00030 commandPolls(6) 00031 { 00032 if (cfg.read("/sd/config.ini")) { 00033 cfg.getValue("Device_ID", deviceId, sizeof(deviceId)); 00034 cfg.getValue("M2X_Key", m2xKey, sizeof(m2xKey)); 00035 cfg.getValue("UDP_Host", udpHost, sizeof(udpHost)); 00036 cfg.getValue("IMEI", devIMEI, sizeof(devIMEI)); 00037 00038 char buff[15]; 00039 int aux; 00040 00041 if (cfg.getValue("Enable_M2X", buff, sizeof(buff))) 00042 enableM2X = strcmp(buff, "1") == 0; 00043 00044 if (cfg.getValue("M2X_Commmands", buff, sizeof(buff))) 00045 enableCommands = strcmp(buff, "1") == 0; 00046 00047 if (cfg.getValue("Enable_UDP", buff, sizeof(buff))) 00048 enableUDP = strcmp(buff, "1") == 0; 00049 00050 if (cfg.getValue("Command_Wait", buff, sizeof(buff))) { 00051 aux = atoi(buff); 00052 if ((aux > 0) && (aux < 3600)) 00053 commandDelay = aux; 00054 }; 00055 00056 if (cfg.getValue("Command_Polls", buff, sizeof(buff))) { 00057 aux = atoi(buff); 00058 if ((aux > 0) && (aux < 10)) 00059 commandPolls = aux; 00060 }; 00061 00062 if (cfg.getValue("UDP_Port", buff, sizeof(buff))) { 00063 aux = atoi(buff); 00064 if ((aux > 0) && (aux < 65535)) 00065 udpPort = aux; 00066 }; 00067 00068 double lat, lon; 00069 00070 if (cfg.getValue("Latitude", buff, sizeof(buff))) 00071 lat = atof(buff); 00072 00073 if (cfg.getValue("Longitude", buff, sizeof(buff))) 00074 lon = atof(buff); 00075 00076 if ((lat >= -90) && (lat <= 90) && (lon >= -180) && (lon <= 180)) { 00077 locLat = lat; 00078 locLong = lon; 00079 } 00080 } 00081 00082 m2xConfigured = *deviceId && *m2xKey; 00083 00084 enableCommands = enableM2X && enableCommands; 00085 }; 00086 00087 void dumpConfig(Serial& pc) { 00088 pc.printf("# config.ini" CRLF); 00089 pc.printf("Enable_M2X=%d" CRLF, enableM2X ? 1 : 0); 00090 pc.printf("Device_ID=%s" CRLF, deviceId); 00091 pc.printf("M2X_Key=%s" CRLF, m2xKey); 00092 pc.printf("M2X_Commands=%d" CRLF, enableCommands ? 1 : 0); 00093 pc.printf("#" CRLF); 00094 pc.printf("Enable_UDP=%d" CRLF, enableUDP ? 1 : 0); 00095 pc.printf("UDP_Host=%s" CRLF, udpHost); 00096 pc.printf("UDP_Port=%d" CRLF, udpPort); 00097 pc.printf("IMEI=%s" CRLF, devIMEI); 00098 pc.printf("Latitude=%f" CRLF, locLat); 00099 pc.printf("Longitude=%f" CRLF, locLong); 00100 pc.printf("#" CRLF); 00101 pc.printf("Command_Wait=%d" CRLF, commandDelay); 00102 pc.printf("Command_Polls=%d" CRLF, commandPolls); 00103 pc.printf("# Data will be sent every %d seconds" CRLF, commandPolls * commandDelay); 00104 } 00105 00106 private: 00107 ConfigFile cfg; 00108 };
Generated on Fri Jul 15 2022 01:40:15 by
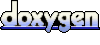