
LED Game Candle Panic details here https://kohacraft.com/candlepanic
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 candle panic 00003 キャンドル パニック 00004 00005 詳しくはこちら 00006 https://kohacraft.com/candlepanic 00007 00008 public domain 00009 */ 00010 00011 #include "mbed.h" 00012 00013 //LED&光センサー 00014 #define ArrayNum 8 00015 DigitalOut ledK[ArrayNum] = { dp1, dp2, dp4, dp6, dp9, dp10, dp13, dp14}; 00016 DigitalOut ledA(dp11); 00017 DigitalIn ledSensor(dp15); 00018 DigitalOut flash(dp5); 00019 bool sensorArray[ArrayNum] = { 0, 0, 0, 0, 0, 0, 0, 0 }; 00020 bool ledArray[ArrayNum] = { 0, 0, 0, 0, 0, 0, 0, 0 }; 00021 #define LedA_Led 0 //LEDをLEDモードにする 00022 #define LedA_Sensor 1 //LEDをセンサーモードにする 00023 00024 //サウンド 00025 #define C1 1/261.626 00026 #define D1 1/293.665 00027 #define E1 1/329.628 00028 #define F1 1/349.228 00029 #define G1 1/391.995 00030 #define A1 1/440.000 00031 #define B1 1/493.883 00032 #define C2 C1/2 00033 #define D2 D1/2 00034 #define E2 E1/2 00035 #define F2 F1/2 00036 #define G2 G1/2 00037 #define A2 A1/2 00038 #define B2 B1/2 00039 const float tone[] = { C1, D1, E1, F1, G1, A1, B1, C2, D2, E2, F2, G2, A2, B2 }; 00040 PwmOut sp(dp18); 00041 bool offBeepOn = 0; //LEDがOFFするときの音を出す 00042 bool onBeepOn = 0; //LEDがONするときの音を出す 00043 int beepDevide = 30; 00044 00045 int loopCount = 0; 00046 int offCount = 500; //loopCountがこの値になったらLEDを一つ消す 00047 int speedUpCount = 30; //loopCountがこの値になったらOffCountを減らす 00048 int goleOffCount = 50; //offCountがこの値になったらゲームクリア 00049 00050 DigitalOut led(dp17); 00051 00052 void lightDetect(); 00053 void beep(float); 00054 void showLed(); 00055 void offBeep(); 00056 void onBeep(); 00057 00058 int main() { 00059 00060 00061 //初期化 00062 for( int i=0 ; i< ArrayNum ; i++ ) 00063 { 00064 ledK[i] = 1; 00065 ledArray[i] = 0; 00066 } 00067 flash = 1; 00068 00069 //LEDを順番に点灯しながら音を鳴らす 00070 00071 for( int i=0 ; i< ArrayNum ; i++ ) 00072 { 00073 ledArray[i] = 1; 00074 showLed(); 00075 beep( tone[i] ); 00076 wait( 0.1 ); 00077 } 00078 beep( 0 ); 00079 00080 int offCounter = 0; 00081 while(1) { 00082 00083 //ランダムにどれか1つ消灯する 00084 if( offCounter > offCount ) 00085 { 00086 while(1) 00087 { 00088 float numFloat = (float)rand()/RAND_MAX * ( ArrayNum - 1 ); 00089 int num = int( numFloat + 0.5 ); 00090 if( ledArray[ num ] == 1 ) 00091 { 00092 ledArray[ num ] = 0; 00093 break; 00094 } 00095 } 00096 00097 offBeepOn = 1; 00098 offCounter = 0; 00099 } 00100 offCounter++; 00101 00102 showLed(); 00103 00104 lightDetect(); //センサ状態を取得 00105 for( int i=0 ; i< ArrayNum ; i++ ) 00106 { 00107 if( ledArray[i] == 0 && sensorArray[i] == 1 ) 00108 { 00109 ledArray[i] = 1; 00110 onBeepOn = 1; 00111 } 00112 } 00113 showLed(); 00114 00115 //全部消えたら終わり 00116 int result = 0; 00117 for( int i=0 ; i< ArrayNum ; i++ ) 00118 { 00119 result += ledArray[i]; 00120 } 00121 if( result == 0 ) 00122 { 00123 break; 00124 } 00125 00126 onBeep(); 00127 offBeep(); 00128 00129 wait(0.002); 00130 loopCount++; 00131 if( loopCount%speedUpCount == 0 ) 00132 { 00133 offCount--; 00134 if( offCount < goleOffCount ) 00135 break; 00136 } 00137 00138 } 00139 00140 if( offCount >= goleOffCount ) 00141 { 00142 //失敗 00143 beep( C1 ); 00144 wait( 0.2 ); 00145 beep( 0 ); 00146 wait( 0.2 ); 00147 beep( C1 ); 00148 wait( 1 ); 00149 beep( 0 ); 00150 00151 } 00152 else 00153 { 00154 //成功 00155 for( int j=0 ; j<3 ; j++ ) 00156 { 00157 for( int i=7 ; i<15 ; i++ ) 00158 { 00159 beep(tone[i]); 00160 wait( 0.05 ); 00161 } 00162 } 00163 beep(0); 00164 00165 while(1) 00166 { 00167 for( int i=0 ; i< ArrayNum ; i++ ) 00168 ledArray[i] = 1; 00169 showLed(); 00170 wait(0.2); 00171 00172 for( int i=0 ; i< ArrayNum ; i++ ) 00173 ledArray[i] = 0; 00174 showLed(); 00175 wait(0.2); 00176 } 00177 } 00178 while(1) 00179 { 00180 ; 00181 } 00182 } 00183 00184 //光センサとして光を検知する 00185 void lightDetect() 00186 { 00187 ledA = LedA_Sensor; //光センサのモードにする 00188 for( int i=0 ; i < ArrayNum ; i++ ) //全てのセンサをOFFにする 00189 { 00190 ledK[i] = 0; 00191 sensorArray[i] = 0; 00192 } 00193 00194 for( int i=0 ; i < ArrayNum ; i++ ) 00195 { 00196 flash = 0; 00197 wait(0.0001); 00198 flash = 1; 00199 00200 ledK[i] = 1; //特定のセンサのみONにする 00201 wait(0.0001); 00202 bool res = ledSensor; //センサの状態を読み込む 00203 if( res == 0 ) 00204 sensorArray[i] = 1; 00205 else 00206 sensorArray[i] = 0; 00207 ledK[i] = 0; //特定のセンサをOFFにする 00208 } 00209 return; 00210 } 00211 00212 //指定した周期でピープを鳴らす 0ならOFF 00213 void beep( float scale ) 00214 { 00215 if( scale != 0 ) 00216 { 00217 sp.period(scale); 00218 sp.write(0.5f); 00219 } 00220 else 00221 { 00222 sp.write(0.0f); 00223 } 00224 } 00225 00226 //ledArrayにしたがってLEDを点灯する 00227 void showLed() 00228 { 00229 for( int i=0 ; i<ArrayNum ; i++ ) 00230 { 00231 if( ledArray[i] == 1) 00232 { 00233 ledK[i] = 0; 00234 } 00235 else 00236 { 00237 ledK[i] = 1; 00238 } 00239 } 00240 ledA = LedA_Led; //LEDのモードにする 00241 } 00242 00243 //LEDがOFFするときのサウンド 00244 bool offBeepRun = 0; 00245 int offBeepCount = 0; 00246 int offMelodyCounter = 0; 00247 void offBeep() 00248 { 00249 int melodyLength = 2; 00250 float melody[2] = { D1, C1 }; 00251 00252 if( offBeepOn == 1 ) 00253 { 00254 offBeepOn = 0; 00255 offBeepRun = 1; 00256 offBeepCount = 0; 00257 offMelodyCounter = 0; 00258 } 00259 00260 if( offBeepRun == 1 ) 00261 { 00262 if( offBeepCount%beepDevide == 0 ) 00263 { 00264 if( offMelodyCounter < melodyLength ) 00265 { 00266 //メロディの長さ以下だったら音を鳴らす 00267 beep( melody[ offMelodyCounter++ ] ); 00268 } 00269 else 00270 { 00271 //音を止める 00272 beep(0); 00273 offBeepRun = 0; 00274 } 00275 } 00276 00277 offBeepCount++; 00278 return; 00279 } 00280 00281 return; 00282 } 00283 00284 //LEDがONするときのサウンド 00285 bool onBeepRun = 0; 00286 int onBeepCount = 0; 00287 int onMelodyCounter = 0; 00288 void onBeep() 00289 { 00290 int melodyLength = 2; 00291 float melody[2] = { C2, D2 }; 00292 00293 if( onBeepOn == 1 ) 00294 { 00295 onBeepOn = 0; 00296 onBeepRun = 1; 00297 onBeepCount = 0; 00298 onMelodyCounter = 0; 00299 } 00300 00301 if( onBeepRun == 1 ) 00302 { 00303 if( onBeepCount%beepDevide == 0 ) 00304 { 00305 if( onMelodyCounter < melodyLength ) 00306 { 00307 //メロディの長さ以下だったら音を鳴らす 00308 beep( melody[ onMelodyCounter++ ] ); 00309 } 00310 else 00311 { 00312 //音を止める 00313 beep(0); 00314 onBeepRun = 0; 00315 } 00316 } 00317 00318 onBeepCount++; 00319 return; 00320 } 00321 00322 return; 00323 } 00324
Generated on Mon Jul 25 2022 04:00:58 by
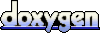