32bits encoder counter using 16bits TIM and a 32bits software counter
Dependents: v1 v2 v2bis GestionPixy ... more
Nucleo_Encoder_16_bits.cpp
00001 #include "Nucleo_Encoder_16_bits.h" 00002 00003 int32_t Soft_32_Counter_TIM2, Soft_32_Counter_TIM3, Soft_32_Counter_TIM4, Soft_32_Counter_TIM5; 00004 00005 void Overflow_Routine_TIM2() 00006 { 00007 if(TIM2->SR & 0x0001) 00008 { 00009 printf("Overflow Routine"); 00010 TIM2->SR &= 0xfffe; 00011 if(!(TIM2->CR1&TIM_CR1_DIR)) 00012 Soft_32_Counter_TIM2 += 0xffff; 00013 else 00014 Soft_32_Counter_TIM2 -= 0xffff; 00015 } 00016 } 00017 00018 void Overflow_Routine_TIM3() 00019 { 00020 if(TIM3->SR & 0x0001) 00021 { 00022 printf("Overflow Routine"); 00023 TIM3->SR &= 0xfffe; 00024 if(!(TIM3->CR1&TIM_CR1_DIR)) 00025 Soft_32_Counter_TIM3 += 0xffff; 00026 else 00027 Soft_32_Counter_TIM3 -= 0xffff; 00028 } 00029 } 00030 void Overflow_Routine_TIM4() 00031 { 00032 if(TIM4->SR & 0x0001) 00033 { 00034 printf("Overflow Routine"); 00035 TIM4->SR &= 0xfffe; 00036 if(!(TIM4->CR1&TIM_CR1_DIR)) 00037 Soft_32_Counter_TIM4 += 0xffff; 00038 else 00039 Soft_32_Counter_TIM4 -= 0xffff; 00040 } 00041 } 00042 void Overflow_Routine_TIM5() 00043 { 00044 if(TIM5->SR & 0x0001) 00045 { 00046 printf("Overflow Routine"); 00047 TIM5->SR &= 0xfffe; 00048 if(!(TIM5->CR1&TIM_CR1_DIR)) 00049 Soft_32_Counter_TIM5 += 0xffff; 00050 else 00051 Soft_32_Counter_TIM5 -= 0xffff; 00052 } 00053 } 00054 00055 namespace mbed 00056 { 00057 00058 Nucleo_Encoder_16_bits::Nucleo_Encoder_16_bits(TIM_TypeDef * _TIM) 00059 { 00060 TIM = _TIM; 00061 // Initialisation of the TIM module as an encoder counter 00062 EncoderInit(&encoder, &timer, _TIM, 0xffff, TIM_ENCODERMODE_TI12); 00063 00064 // Update (aka over- and underflow) interrupt enabled 00065 TIM->DIER |= 0x0001; 00066 // The initialisation process generates an update interrupt, so we'll have to clear the update flag before anything else 00067 TIM->SR &= 0xfffe; 00068 00069 // Setting the ISR for the corresponding interrupt vector 00070 switch((uint32_t)TIM) 00071 { 00072 case TIM2_BASE : 00073 NVIC_SetVector(TIM2_IRQn, (uint32_t)&Overflow_Routine_TIM2); 00074 NVIC_EnableIRQ(TIM2_IRQn); 00075 Soft_32_Counter_TIM2 = 0; 00076 break; 00077 00078 case TIM3_BASE : 00079 NVIC_SetVector(TIM3_IRQn, (uint32_t)&Overflow_Routine_TIM3); 00080 NVIC_EnableIRQ(TIM3_IRQn); 00081 Soft_32_Counter_TIM3 = 0; 00082 break; 00083 00084 case TIM4_BASE : 00085 NVIC_SetVector(TIM4_IRQn, (uint32_t)&Overflow_Routine_TIM4); 00086 NVIC_EnableIRQ(TIM4_IRQn); 00087 Soft_32_Counter_TIM4 = 0; 00088 break; 00089 00090 case TIM5_BASE : 00091 NVIC_SetVector(TIM5_IRQn, (uint32_t)&Overflow_Routine_TIM5); 00092 NVIC_EnableIRQ(TIM5_IRQn); 00093 Soft_32_Counter_TIM5 = 0; 00094 break; 00095 00096 default : 00097 00098 break; 00099 } 00100 00101 } 00102 Nucleo_Encoder_16_bits::Nucleo_Encoder_16_bits(TIM_TypeDef * _TIM, uint32_t _maxcount, uint32_t _encmode) 00103 { 00104 TIM = _TIM; 00105 // Initialisation of the TIM module as an encoder counter 00106 EncoderInit(&encoder, &timer, _TIM, _maxcount, _encmode); 00107 00108 // Update (aka over- and underflow) interrupt enabled 00109 TIM->DIER |= 0x0001; 00110 // The initialisation process generates an update interrupt, so we'll have to clear the update flag before anything else 00111 TIM->SR &= 0xfffe; 00112 00113 // Setting the ISR for the corresponding interrupt vector 00114 switch((uint32_t)TIM) 00115 { 00116 case TIM2_BASE : 00117 NVIC_SetVector(TIM2_IRQn, (uint32_t)&Overflow_Routine_TIM2); 00118 NVIC_EnableIRQ(TIM2_IRQn); 00119 Soft_32_Counter_TIM2 = 0; 00120 break; 00121 00122 case TIM3_BASE : 00123 NVIC_SetVector(TIM3_IRQn, (uint32_t)&Overflow_Routine_TIM3); 00124 NVIC_EnableIRQ(TIM3_IRQn); 00125 Soft_32_Counter_TIM3 = 0; 00126 break; 00127 00128 case TIM4_BASE : 00129 NVIC_SetVector(TIM4_IRQn, (uint32_t)&Overflow_Routine_TIM4); 00130 NVIC_EnableIRQ(TIM4_IRQn); 00131 Soft_32_Counter_TIM4 = 0; 00132 break; 00133 00134 case TIM5_BASE : 00135 NVIC_SetVector(TIM5_IRQn, (uint32_t)&Overflow_Routine_TIM5); 00136 NVIC_EnableIRQ(TIM5_IRQn); 00137 Soft_32_Counter_TIM5 = 0; 00138 break; 00139 00140 default : 00141 00142 break; 00143 } 00144 00145 } 00146 00147 Nucleo_Encoder_16_bits::Nucleo_Encoder_16_bits(TIM_Encoder_InitTypeDef * _encoder, TIM_HandleTypeDef * _timer, TIM_TypeDef * _TIM, uint32_t _maxcount, uint32_t _encmode) 00148 { 00149 timer = *_timer; 00150 encoder = *_encoder; 00151 TIM = _TIM; 00152 // Initialisation of the TIM module as an encoder counter 00153 EncoderInit(&encoder, &timer, _TIM, _maxcount, _encmode); 00154 00155 // Update (aka over- and underflow) interrupt enabled 00156 TIM->DIER |= 0x0001; 00157 // The initialisation process generates an update interrupt, so we'll have to clear the update flag before anything else 00158 TIM->SR &= 0xfffe; 00159 00160 // Setting the ISR for the corresponding interrupt vector 00161 switch((uint32_t)TIM) 00162 { 00163 case TIM2_BASE : 00164 NVIC_SetVector(TIM2_IRQn, (uint32_t)&Overflow_Routine_TIM2); 00165 NVIC_EnableIRQ(TIM2_IRQn); 00166 Soft_32_Counter_TIM2 = 0; 00167 break; 00168 00169 case TIM3_BASE : 00170 NVIC_SetVector(TIM3_IRQn, (uint32_t)&Overflow_Routine_TIM3); 00171 NVIC_EnableIRQ(TIM3_IRQn); 00172 Soft_32_Counter_TIM3 = 0; 00173 break; 00174 00175 case TIM4_BASE : 00176 NVIC_SetVector(TIM4_IRQn, (uint32_t)&Overflow_Routine_TIM4); 00177 NVIC_EnableIRQ(TIM4_IRQn); 00178 Soft_32_Counter_TIM4 = 0; 00179 break; 00180 00181 case TIM5_BASE : 00182 NVIC_SetVector(TIM5_IRQn, (uint32_t)&Overflow_Routine_TIM5); 00183 NVIC_EnableIRQ(TIM5_IRQn); 00184 Soft_32_Counter_TIM5 = 0; 00185 break; 00186 00187 default : 00188 00189 break; 00190 } 00191 00192 } 00193 00194 00195 int32_t Nucleo_Encoder_16_bits::GetCounter() 00196 { 00197 uint16_t count = TIM->CNT; 00198 switch((uint32_t)TIM) 00199 { 00200 case TIM2_BASE : 00201 return (int32_t)count + Soft_32_Counter_TIM2; 00202 break; 00203 00204 case TIM3_BASE : 00205 return (int32_t)count + Soft_32_Counter_TIM3; 00206 break; 00207 00208 case TIM4_BASE : 00209 return (int32_t)count + Soft_32_Counter_TIM4; 00210 break; 00211 00212 case TIM5_BASE : 00213 return (int32_t)count + Soft_32_Counter_TIM5; 00214 break; 00215 } 00216 00217 return (int32_t)count; 00218 } 00219 00220 TIM_HandleTypeDef* Nucleo_Encoder_16_bits::GetTimer() 00221 { 00222 return &timer; 00223 } 00224 00225 00226 00227 00228 00229 00230 }
Generated on Wed Jul 20 2022 14:22:54 by
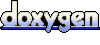