A - floating heart B - FOX
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "MicroBit.h" 00002 00003 MicroBit uBit; 00004 00005 void onButtonA(MicroBitEvent e) { 00006 MicroBitImage heart("0, 1, 0, 1, 0\n1, 1, 1, 1, 1\n1, 1, 1, 1, 1\n0, 1, 1, 1, 0\n0, 0, 1, 0, 0\n"); 00007 uBit.display.setDisplayMode(DISPLAY_MODE_BLACK_AND_WHITE_LIGHT_SENSE); 00008 for (int y = 4; y >= 0; --y) { 00009 uBit.display.image.paste(heart, 0, y); 00010 uBit.sleep(500); 00011 } 00012 } 00013 00014 void onButtonB(MicroBitEvent e) { 00015 uBit.display.print("FOX"); 00016 } 00017 00018 int main() 00019 { 00020 // Initialise the micro:bit runtime. 00021 uBit.init(); 00022 00023 // Insert your code here! 00024 00025 uBit.messageBus.listen(MICROBIT_ID_BUTTON_A, MICROBIT_BUTTON_EVT_CLICK, onButtonA); 00026 uBit.messageBus.listen(MICROBIT_ID_BUTTON_B, MICROBIT_BUTTON_EVT_CLICK, onButtonB); 00027 00028 // If main exits, there may still be other fibers running or registered event handlers etc. 00029 // Simply release this fiber, which will mean we enter the scheduler. Worse case, we then 00030 // sit in the idle task forever, in a power efficient sleep. 00031 release_fiber(); 00032 } 00033
Generated on Fri Jul 15 2022 20:39:09 by
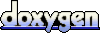