aitendo SX032QVGA008
Dependencies: SPI_TFT_ILI9341ext
SX032QVGA008.h
00001 /* mbed library for aitendo SX032QVGA08 TFT module with resistive touch panel. 00002 * 00003 * Original library is "SpeedStudioTFT" by 2014 Copyright (c) Seeed Technology Inc. 00004 * I made by it to the reference. 00005 * 00006 * (1) Because there was some mistake in the source, I was corrected. 00007 * (2) Also, I changed to the source you have to assume FRDM 00008 * (3) I changed BackLight is PWM controlled. 00009 */ 00010 00011 #ifndef SX032QVGA008_H 00012 #define SX032QVGA008_H 00013 00014 #include "mbed.h" 00015 #include "SPI_TFT_ILI9341ext.h" 00016 #ifdef USE_SDCARD 00017 #include "SDFileSystem.h" // import the SDFileSystem library 00018 #endif 00019 00020 struct point { 00021 int x; 00022 int y; 00023 }; 00024 00025 class SX032QVGA008 : public 00026 #ifdef USE_SDCARD 00027 SDFileSystem, 00028 #endif 00029 SPI_TFT_ILI9341ext 00030 { 00031 public: 00032 /** create a TFT with touch object connected to the pins: 00033 * 00034 * @param pin xp resistiv touch x+ 00035 * @param pin xm resistiv touch x- 00036 * @param pin yp resistiv touch y+ 00037 * @param pin ym resistiv touch y- 00038 * @param mosi,miso,sclk SPI connection to TFT 00039 * @param cs pin connected to CS of display 00040 * @param rs pin connected to RS(DC) of display 00041 * @param reset pin connected to RESET of display 00042 * @param backlight pin connected to bkl of display 00043 * ( You must drive N-MOSFET.pin is connected to NMOS gate terminal.) 00044 * @param sdcard_cs pin connected to CS of sdcard 00045 * based on my SPI_TFT lib 00046 */ 00047 SX032QVGA008(PinName xp, PinName xm, PinName yp, PinName ym, 00048 PinName mosi, PinName miso, PinName sclk, 00049 PinName csTft, PinName dcTft, PinName resTft, PinName blTft, 00050 PinName csSd); 00051 00052 /** Backlight PWM controll 00053 * 00054 * @param duty is PWM duty (0 - 1.0) 00055 * period is 0.01 fixed. 00056 */ 00057 void setBacklight(float duty); 00058 00059 /** calibrate the touch display 00060 * 00061 * User is asked to touch on two points on the screen 00062 */ 00063 void calibrate(void); 00064 00065 /** read x and y coord on screen 00066 * 00067 * @returns point(x,y) 00068 */ 00069 bool 00070 getPixel(point& p); 00071 00072 /** calculate coord on screen 00073 * 00074 * @param a_point point(analog x, analog y) 00075 * @returns point(pixel x, pixel y) 00076 * 00077 */ 00078 point toPixel(point p); 00079 00080 protected: 00081 PinName _xm; 00082 PinName _ym; 00083 PinName _xp; 00084 PinName _yp; 00085 PwmOut bl; 00086 00087 typedef enum { YES, MAYBE, NO } TOUCH; 00088 TOUCH getTouch(point& p); 00089 int readTouch(PinName p, PinName m, PinName a, PinName i); 00090 00091 int x_off,y_off; 00092 int pp_tx,pp_ty; 00093 }; 00094 00095 #endif
Generated on Wed Jul 13 2022 04:42:58 by
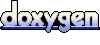