KSM edits to RA8875
Embed:
(wiki syntax)
Show/hide line numbers
GraphicsDisplay.h
00001 /// @page GraphicDisplay_Copyright GraphicDisplay Library Base Class 00002 /// 00003 /// mbed GraphicsDisplay Display Library Base Class 00004 /// @copyright © 2007-2009 sford 00005 /// Released under the MIT License: http://mbed.org/license/mit 00006 /// 00007 /// A library for providing a common base class for Graphics displays 00008 /// To port a new display, derive from this class and implement 00009 /// the constructor (setup the display), pixel (put a pixel 00010 /// at a location), width and height functions. Everything else 00011 /// (locate, printf, putc, cls, window, putp, fill, blit, blitbit) 00012 /// will come for free. You can also provide a specialised implementation 00013 /// of window and putp to speed up the results 00014 /// 00015 #ifndef MBED_GRAPHICSDISPLAY_H 00016 #define MBED_GRAPHICSDISPLAY_H 00017 #include "Bitmap.h" 00018 #include "TextDisplay.h" 00019 #include "GraphicsDisplayJPEG.h" 00020 #include "GraphicsDisplayGIF.h" 00021 00022 /// The GraphicsDisplay class 00023 /// 00024 /// This graphics display class supports both graphics and text operations. 00025 /// Typically, a subclass is derived from this which has localizations to 00026 /// adapt to a specific hardware platform (e.g. a display controller chip), 00027 /// that overrides methods in here to either add more capability or perhaps 00028 /// to improve performance, by leveraging specific hardware capabilities. 00029 /// 00030 class GraphicsDisplay : public TextDisplay 00031 { 00032 public: 00033 /// The constructor 00034 GraphicsDisplay(const char* name); 00035 00036 //~GraphicsDisplay(); 00037 00038 /// Draw a pixel in the specified color. 00039 /// 00040 /// @note this method must be supported in the derived class. 00041 /// 00042 /// @param[in] x is the horizontal offset to this pixel. 00043 /// @param[in] y is the vertical offset to this pixel. 00044 /// @param[in] color defines the color for the pixel. 00045 /// @returns success/failure code. @see RetCode_t. 00046 /// 00047 virtual RetCode_t pixel(loc_t x, loc_t y, color_t color) = 0; 00048 00049 /// Write a stream of pixels to the display. 00050 /// 00051 /// @note this method must be supported in the derived class. 00052 /// 00053 /// @param[in] p is a pointer to a color_t array to write. 00054 /// @param[in] count is the number of pixels to write. 00055 /// @param[in] x is the horizontal position on the display. 00056 /// @param[in] y is the vertical position on the display. 00057 /// @returns success/failure code. @see RetCode_t. 00058 /// 00059 virtual RetCode_t pixelStream(color_t * p, uint32_t count, loc_t x, loc_t y) = 0; 00060 00061 /// Get a pixel from the display. 00062 /// 00063 /// @note this method must be supported in the derived class. 00064 /// 00065 /// @param[in] x is the horizontal offset to this pixel. 00066 /// @param[in] y is the vertical offset to this pixel. 00067 /// @returns the pixel. @see color_t 00068 /// 00069 virtual color_t getPixel(loc_t x, loc_t y) = 0; 00070 00071 /// Get a stream of pixels from the display. 00072 /// 00073 /// @note this method must be supported in the derived class. 00074 /// 00075 /// @param[out] p is a pointer to a color_t array to accept the stream. 00076 /// @param[in] count is the number of pixels to read. 00077 /// @param[in] x is the horizontal offset to this pixel. 00078 /// @param[in] y is the vertical offset to this pixel. 00079 /// @returns success/failure code. @see RetCode_t. 00080 /// 00081 virtual RetCode_t getPixelStream(color_t * p, uint32_t count, loc_t x, loc_t y) = 0; 00082 00083 /// get the screen width in pixels 00084 /// 00085 /// @note this method must be supported in the derived class. 00086 /// 00087 /// @returns screen width in pixels. 00088 /// 00089 virtual uint16_t width() = 0; 00090 00091 /// get the screen height in pixels 00092 /// 00093 /// @note this method must be supported in the derived class. 00094 /// 00095 /// @returns screen height in pixels. 00096 /// 00097 virtual uint16_t height() = 0; 00098 00099 /// Prepare the controller to write binary data to the screen by positioning 00100 /// the memory cursor. 00101 /// 00102 /// @note this method must be supported in the derived class. 00103 /// 00104 /// @param[in] x is the horizontal position in pixels (from the left edge) 00105 /// @param[in] y is the vertical position in pixels (from the top edge) 00106 /// @returns success/failure code. @see RetCode_t. 00107 /// 00108 virtual RetCode_t SetGraphicsCursor(loc_t x, loc_t y) = 0; 00109 00110 00111 /// Prepare the controller to write binary data to the screen by positioning 00112 /// the memory cursor. 00113 /// 00114 /// @param[in] p is the point representing the cursor position to set 00115 /// @returns @ref RetCode_t value. 00116 /// 00117 virtual RetCode_t SetGraphicsCursor(point_t p) = 0; 00118 00119 /// Read the current graphics cursor position as a point. 00120 /// 00121 /// @returns the graphics cursor as a point. 00122 /// 00123 virtual point_t GetGraphicsCursor(void) = 0; 00124 00125 /// Prepare the controller to read binary data from the screen by positioning 00126 /// the memory read cursor. 00127 /// 00128 /// @param[in] x is the horizontal position in pixels (from the left edge) 00129 /// @param[in] y is the vertical position in pixels (from the top edge) 00130 /// @returns success/failure code. @see RetCode_t. 00131 /// 00132 virtual RetCode_t SetGraphicsCursorRead(loc_t x, loc_t y) = 0; 00133 00134 /// Draw a filled rectangle in the specified color 00135 /// 00136 /// @note As a side effect, this changes the current 00137 /// foreground color for subsequent operations. 00138 /// 00139 /// @note this method must be supported in the derived class. 00140 /// 00141 /// @param[in] x1 is the horizontal start of the line. 00142 /// @param[in] y1 is the vertical start of the line. 00143 /// @param[in] x2 is the horizontal end of the line. 00144 /// @param[in] y2 is the vertical end of the line. 00145 /// @param[in] color defines the foreground color. 00146 /// @param[in] fillit is optional to NOFILL the rectangle. default is FILL. 00147 /// @returns success/failure code. @see RetCode_t. 00148 /// 00149 virtual RetCode_t fillrect(loc_t x1, loc_t y1, loc_t x2, loc_t y2, 00150 color_t color, fill_t fillit = FILL) = 0; 00151 00152 /// Select the drawing layer for subsequent commands. 00153 /// 00154 /// If the screen configuration is 480 x 272, or if it is 800 x 480 00155 /// and 8-bit color, the the display supports two layers, which can 00156 /// be independently drawn on and shown. Additionally, complex 00157 /// operations involving both layers are permitted. 00158 /// 00159 /// @code 00160 /// //lcd.SetLayerMode(OnlyLayer0); // default is layer 0 00161 /// lcd.rect(400,130, 475,155,Brown); 00162 /// lcd.SelectDrawingLayer(1); 00163 /// lcd.circle(400,25, 25, BrightRed); 00164 /// wait(1); 00165 /// lcd.SetLayerMode(ShowLayer1); 00166 /// @endcode 00167 /// 00168 /// @attention The user manual refers to Layer 1 and Layer 2, however the 00169 /// actual register values are value 0 and 1. This API as well as 00170 /// others that reference the layers use the values 0 and 1 for 00171 /// cleaner iteration in the code. 00172 /// 00173 /// @param[in] layer is 0 or 1 to select the layer for subsequent 00174 /// commands. 00175 /// @param[out] prevLayer is an optiona pointer to where the previous layer 00176 /// will be written, making it a little easer to restore layers. 00177 /// Writes 0 or 1 when the pointer is not NULL. 00178 /// @returns @ref RetCode_t value. 00179 /// 00180 virtual RetCode_t SelectDrawingLayer(uint16_t layer, uint16_t * prevLayer = NULL) = 0; 00181 00182 00183 /// Get the currently active drawing layer. 00184 /// 00185 /// This returns a value, 0 or 1, based on the screen configuration 00186 /// and the currently active drawing layer. 00187 /// 00188 /// @code 00189 /// uint16_t prevLayer; 00190 /// lcd.SelectDrawingLayer(x, &prevLayer); 00191 /// lcd.circle(400,25, 25, BrightRed); 00192 /// lcd.SelectDrawingLayer(prevLayer); 00193 /// @endcode 00194 /// 00195 /// @attention The user manual refers to Layer 1 and Layer 2, however the 00196 /// actual register values are value 0 and 1. This API as well as 00197 /// others that reference the layers use the values 0 and 1 for 00198 /// cleaner iteration in the code. 00199 /// 00200 /// @returns the current drawing layer; 0 or 1. 00201 /// 00202 virtual uint16_t GetDrawingLayer(void) = 0; 00203 00204 /// a function to write the command and data to the RA8875 chip. 00205 /// 00206 /// @param command is the RA8875 instruction to perform 00207 /// @param data is the optional data to the instruction. 00208 /// @returns success/failure code. @see RetCode_t. 00209 /// 00210 virtual RetCode_t WriteCommand(unsigned char command, unsigned int data = 0xFFFF) = 0; 00211 00212 00213 /// a function to write the data to the RA8875 chip. 00214 /// 00215 /// This is typically used after a command has been initiated, and where 00216 /// there may be a data stream to follow. 00217 /// 00218 /// @param data is the optional data to the instruction. 00219 /// @returns success/failure code. @see RetCode_t. 00220 /// 00221 virtual RetCode_t WriteData(unsigned char data) = 0; 00222 00223 /// Set the window, which controls where items are written to the screen. 00224 /// 00225 /// When something hits the window width, it wraps back to the left side 00226 /// and down a row. 00227 /// 00228 /// @note If the initial write is outside the window, it will 00229 /// be captured into the window when it crosses a boundary. It may 00230 /// be appropriate to SetGraphicsCursor() to a point in the window. 00231 /// 00232 /// @param[in] r is the rect_t rect to define the window. 00233 /// @returns success/failure code. @see RetCode_t. 00234 /// 00235 virtual RetCode_t window(rect_t r); 00236 00237 /// Set the window, which controls where items are written to the screen. 00238 /// 00239 /// When something hits the window width, it wraps back to the left side 00240 /// and down a row. 00241 /// 00242 /// @note If the initial write is outside the window, it will 00243 /// be captured into the window when it crosses a boundary. It may 00244 /// be appropriate to SetGraphicsCursor() to a point in the window. 00245 /// 00246 /// @note if no parameters are provided, it restores the window to full screen. 00247 /// 00248 /// @param[in] x is the left edge in pixels. 00249 /// @param[in] y is the top edge in pixels. 00250 /// @param[in] w is the window width in pixels. 00251 /// @param[in] h is the window height in pixels. 00252 /// @returns success/failure code. @see RetCode_t. 00253 /// 00254 virtual RetCode_t window(loc_t x = 0, loc_t y = 0, dim_t w = (dim_t)-1, dim_t h = (dim_t)-1); 00255 00256 /// method to set the window region to the full screen. 00257 /// 00258 /// This restores the 'window' to the full screen, so that 00259 /// other operations (@see cls) would clear the whole screen. 00260 /// 00261 /// @returns success/failure code. @see RetCode_t. 00262 /// 00263 virtual RetCode_t WindowMax(void); 00264 00265 /// Clear the screen. 00266 /// 00267 /// The behavior is to clear the whole screen. 00268 /// 00269 /// @param[in] layers is ignored, but supports maintaining the same 00270 /// API for the graphics layer. 00271 /// @returns success/failure code. @see RetCode_t. 00272 /// 00273 virtual RetCode_t cls(uint16_t layers = 0); 00274 00275 /// method to put a single color pixel to the screen. 00276 /// 00277 /// This method may be called as many times as necessary after 00278 /// @see _StartGraphicsStream() is called, and it should be followed 00279 /// by _EndGraphicsStream. 00280 /// 00281 /// @param[in] pixel is a color value to be put on the screen. 00282 /// @returns success/failure code. @see RetCode_t. 00283 /// 00284 virtual RetCode_t _putp(color_t pixel); 00285 00286 /// method to fill a region. 00287 /// 00288 /// This method fills a region with the specified color. It essentially 00289 /// is an alias for fillrect, however this uses width and height rather 00290 /// than a second x,y pair. 00291 /// 00292 /// @param[in] x is the left-edge of the region. 00293 /// @param[in] y is the top-edge of the region. 00294 /// @param[in] w specifies the width of the region. 00295 /// @param[in] h specifies the height of the region. 00296 /// @param[in] color is the color value to use to fill the region 00297 /// @returns success/failure code. @see RetCode_t. 00298 /// 00299 virtual RetCode_t fill(loc_t x, loc_t y, dim_t w, dim_t h, color_t color); 00300 00301 /// method to stream bitmap data to the display 00302 /// 00303 /// This method fills a region from a stream of color data. 00304 /// 00305 /// @param[in] x is the left-edge of the region. 00306 /// @param[in] y is the top-edge of the region. 00307 /// @param[in] w specifies the width of the region. 00308 /// @param[in] h specifies the height of the region. 00309 /// @param[in] color is a pointer to a color stream with w x h values. 00310 /// @returns success/failure code. @see RetCode_t. 00311 /// 00312 virtual RetCode_t blit(loc_t x, loc_t y, dim_t w, dim_t h, const int * color); 00313 00314 /// This method returns the width in pixels of the chosen character 00315 /// from the previously selected external font. 00316 /// 00317 /// @param[in] c is the character of interest. 00318 /// @param[in, out] width is a pointer to where the width will be stored. 00319 /// This parameter is NULL tested and will only be written if not null 00320 /// which is convenient if you only want the height. 00321 /// @param[in, out] height is a pointer to where the height will be stored. 00322 /// This parameter is NULL tested and will only be written if not null 00323 /// which is convenient if you only want the width. 00324 /// @returns a pointer to the raw character data or NULL if not found. 00325 /// 00326 virtual const uint8_t * getCharMetrics(const unsigned char c, dim_t * width, dim_t * height); 00327 00328 /// This method transfers one character from the external font data 00329 /// to the screen. 00330 /// 00331 /// The font being used has already been set with the SelectUserFont 00332 /// API. 00333 /// 00334 /// @note the font data is in a special format as generate by 00335 /// the mikroe font creator. 00336 /// See http://www.mikroe.com/glcd-font-creator/ 00337 /// 00338 /// @param[in] x is the horizontal pixel coordinate 00339 /// @param[in] y is the vertical pixel coordinate 00340 /// @param[in] c is the character to render 00341 /// @returns how far the cursor should advance to the right in pixels. 00342 /// @returns zero if the character could not be rendered. 00343 /// 00344 virtual int fontblit(loc_t x, loc_t y, const unsigned char c); 00345 00346 /// This method returns the color value from a palette. 00347 /// 00348 /// This method accepts a pointer to a Bitmap color palette, which 00349 /// is a table in memory composed of RGB Quad values (r, g, b, 0), 00350 /// and an index into that table. It then extracts the color information 00351 /// and downsamples it to a color_t value which it returns. 00352 /// 00353 /// @note This method probably has very little value outside of 00354 /// the internal methods for reading BMP files. 00355 /// 00356 /// @param[in] colorPaletteArray is the handle to the color palette array to use. 00357 /// @param[in] index is the index into the color palette. 00358 /// @returns the color in color_t format. 00359 /// 00360 color_t RGBQuadToRGB16(RGBQUAD * colorPaletteArray, uint16_t index); 00361 00362 /// This method converts a 16-bit color value into a 24-bit RGB Quad. 00363 /// 00364 /// @param[in] c is the 16-bit color. @see color_t. 00365 /// @returns an RGBQUAD value. @see RGBQUAD 00366 /// 00367 RGBQUAD RGB16ToRGBQuad(color_t c); 00368 00369 /// This method attempts to render a specified graphics image file at 00370 /// the specified screen location. 00371 /// 00372 /// This supports several variants of the following file types: 00373 /// \li Bitmap file format, 00374 /// \li Icon file format. 00375 /// 00376 /// @note The specified image width and height, when adjusted for the 00377 /// x and y origin, must fit on the screen, or the image will not 00378 /// be shown (it does not clip the image). 00379 /// 00380 /// @note The file extension is tested, and if it ends in a supported 00381 /// format, the appropriate handler is called to render that image. 00382 /// 00383 /// @param[in] x is the horizontal pixel coordinate 00384 /// @param[in] y is the vertical pixel coordinate 00385 /// @param[in] FileName refers to the fully qualified path and file on 00386 /// a mounted file system. 00387 /// @returns success or error code. 00388 /// 00389 RetCode_t RenderImageFile(loc_t x, loc_t y, const char *FileName); 00390 00391 /// This method reads a disk file that is in jpeg format and 00392 /// puts it on the screen. 00393 /// 00394 /// @param[in] x is the horizontal pixel coordinate 00395 /// @param[in] y is the vertical pixel coordinate 00396 /// @param[in] Name_JPG is the filename on the mounted file system. 00397 /// @returns success or error code. 00398 /// 00399 RetCode_t RenderJpegFile(loc_t x, loc_t y, const char *Name_JPG); 00400 00401 /// This method reads a disk file that is in bitmap format and 00402 /// puts it on the screen. 00403 /// 00404 /// Supported formats: 00405 /// \li 1-bit color format (2 colors) 00406 /// \li 4-bit color format (16 colors) 00407 /// \li 8-bit color format (256 colors) 00408 /// \li 16-bit color format (65k colors) 00409 /// \li 24-bit color format (16M colors) 00410 /// \li compression: no. 00411 /// 00412 /// @note This is a slow operation, typically due to the use of 00413 /// the file system, and partially because bmp files 00414 /// are stored from the bottom up, and the memory is written 00415 /// from the top down; as a result, it constantly 'seeks' 00416 /// on the file system for the next row of information. 00417 /// 00418 /// As a performance test, a sample picture was timed. A family picture 00419 /// was converted to Bitmap format; shrunk to 352 x 272 pixels and save 00420 /// in 8-bit color format. The resulting file size was 94.5 KByte. 00421 /// The SPI port interface was set to 20 MHz. 00422 /// The original bitmap rendering software was purely in software, 00423 /// pushing 1 pixel at a time to the write function, which did use SPI 00424 /// hardware (not pin wiggling) to transfer commands and data to the 00425 /// display. Then, the driver was improved to leverage the capability 00426 /// of the derived display driver. As a final check, instead of the 00427 /// [known slow] local file system, a randomly chosen USB stick was 00428 /// used. The performance results are impressive (but depend on the 00429 /// listed factors). 00430 /// 00431 /// \li 34 seconds, LocalFileSystem, Software Rendering 00432 /// \li 9 seconds, LocalFileSystem, Hardware Rending for RA8875 00433 /// \li 3 seconds, MSCFileSystem, Hardware Rendering for RA8875 00434 /// 00435 /// @param[in] x is the horizontal pixel coordinate 00436 /// @param[in] y is the vertical pixel coordinate 00437 /// @param[in] Name_BMP is the filename on the mounted file system. 00438 /// @returns success or error code. 00439 /// 00440 RetCode_t RenderBitmapFile(loc_t x, loc_t y, const char *Name_BMP); 00441 00442 00443 /// This method reads a disk file that is in ico format and 00444 /// puts it on the screen. 00445 /// 00446 /// Reading the disk is slow, but a typical icon file is small 00447 /// so it should be ok. 00448 /// 00449 /// @note An Icon file can have more than one icon in it. This 00450 /// implementation only processes the first image in the file. 00451 /// 00452 /// @param[in] x is the horizontal pixel coordinate 00453 /// @param[in] y is the vertical pixel coordinate 00454 /// @param[in] Name_ICO is the filename on the mounted file system. 00455 /// @returns success or error code. 00456 /// 00457 RetCode_t RenderIconFile(loc_t x, loc_t y, const char *Name_ICO); 00458 00459 00460 /// Render a GIF file on screen. 00461 /// 00462 /// This function reads a GIF file, and places it onscreen at the specified 00463 /// coordinates. 00464 /// 00465 /// @param[in] x is the left edge of the on-screen coordinates. 00466 /// @param[in] y is the top edge of the on-screen coordinates. 00467 /// @param[in] Name_GIF is a pointer to the fully qualified filename. 00468 /// @returns noerror, or a variety of error codes. 00469 /// 00470 RetCode_t RenderGIFFile(loc_t x, loc_t y, const char *Name_GIF); 00471 00472 00473 /// GetGIFMetrics 00474 /// 00475 /// Get the GIF Image metrics. 00476 /// 00477 /// @param[out] imageDescriptor contains the image metrics on success. 00478 /// @param[in] Name_GIF is the filename of the GIF file of interest. 00479 /// @returns noerror, or a variety of error codes. 00480 /// 00481 RetCode_t GetGIFMetrics(gif_screen_descriptor_t * imageDescriptor, const char * Name_GIF); 00482 00483 00484 /// prints one character at the specified coordinates. 00485 /// 00486 /// This will print the character at the specified pixel coordinates. 00487 /// 00488 /// @param[in] x is the horizontal offset in pixels. 00489 /// @param[in] y is the vertical offset in pixels. 00490 /// @param[in] value is the character to print. 00491 /// @returns number of pixels to index to the right if a character was printed, 0 otherwise. 00492 /// 00493 virtual int character(int x, int y, int value); 00494 00495 /// get the number of colums based on the currently active font 00496 /// 00497 /// @returns number of columns. 00498 /// 00499 virtual int columns(void); 00500 00501 /// get the number of rows based on the currently active font 00502 /// 00503 /// @returns number of rows. 00504 /// 00505 virtual int rows(void); 00506 00507 /// Select a User Font for all subsequent text. 00508 /// 00509 /// @note Tool to create the fonts is accessible from its creator 00510 /// available at http://www.mikroe.com. 00511 /// For version 1.2.0.0, choose the "Export for TFT and new GLCD" 00512 /// format. 00513 /// 00514 /// @param[in] font is a pointer to a specially formed font resource. 00515 /// @returns @ref RetCode_t value. 00516 /// 00517 virtual RetCode_t SelectUserFont(const uint8_t * font = NULL); 00518 00519 00520 protected: 00521 00522 /// Pure virtual method indicating the start of a graphics stream. 00523 /// 00524 /// This is called prior to a stream of pixel data being sent. 00525 /// This may cause register configuration changes in the derived 00526 /// class in order to prepare the hardware to accept the streaming 00527 /// data. 00528 /// 00529 /// @note this method must be supported in the derived class. 00530 /// 00531 /// @returns @ref RetCode_t value. 00532 /// 00533 virtual RetCode_t _StartGraphicsStream(void) = 0; 00534 00535 /// Pure virtual method indicating the end of a graphics stream. 00536 /// 00537 /// This is called to conclude a stream of pixel data that was sent. 00538 /// This may cause register configuration changes in the derived 00539 /// class in order to stop the hardware from accept the streaming 00540 /// data. 00541 /// 00542 /// @note this method must be supported in the derived class. 00543 /// 00544 /// @returns @ref RetCode_t value. 00545 /// 00546 virtual RetCode_t _EndGraphicsStream(void) = 0; 00547 00548 /// Protected method to render an image given a file handle and 00549 /// coordinates. 00550 /// 00551 /// @param[in] x is the horizontal pixel coordinate 00552 /// @param[in] y is the vertical pixel coordinate 00553 /// @param[in] fileOffset is the offset into the file where the image data starts 00554 /// @param[in] fh is the file handle to the stream already opened for the data. 00555 /// @returns success or error code. 00556 /// 00557 RetCode_t _RenderBitmap(loc_t x, loc_t y, uint32_t fileOffset, FILE * fh); 00558 00559 00560 /// Protected method to render a GIF given a file handle and 00561 /// coordinates. 00562 /// 00563 /// @param[in] x is the horizontal pixel coordinate 00564 /// @param[in] y is the vertical pixel coordinate 00565 /// @param[in] fh is the file handle to the stream already opened for the data. 00566 /// @returns success or error code. 00567 /// 00568 RetCode_t _RenderGIF(loc_t x, loc_t y, FILE * fh); 00569 00570 00571 private: 00572 00573 loc_t img_x; /// x position of a rendered jpg 00574 loc_t img_y; /// y position of a rendered jpg 00575 00576 /// Analyze the jpeg data in preparation for decompression. 00577 /// 00578 JRESULT jd_prepare(JDEC * jd, uint16_t(* infunc)(JDEC * jd, uint8_t * buffer, uint16_t bufsize), void * pool, uint16_t poolsize, void * filehandle); 00579 00580 /// Decompress the jpeg and render it. 00581 /// 00582 JRESULT jd_decomp(JDEC * jd, uint16_t(* outfunct)(JDEC * jd, void * stream, JRECT * rect), uint8_t scale); 00583 00584 /// helper function to read data from the file system 00585 /// 00586 uint16_t privInFunc(JDEC * jd, uint8_t * buff, uint16_t ndata); 00587 00588 /// helper function to read data from the file system 00589 /// 00590 uint16_t getJpegData(JDEC * jd, uint8_t *buff, uint16_t ndata); 00591 00592 /// helper function to write data to the display 00593 /// 00594 uint16_t privOutFunc(JDEC * jd, void * bitmap, JRECT * rect); 00595 00596 JRESULT mcu_output ( 00597 JDEC * jd, /* Pointer to the decompressor object */ 00598 uint16_t (* outfunc)(JDEC * jd, void * stream, JRECT * rect), /* RGB output function */ 00599 uint16_t x, /* MCU position in the image (left of the MCU) */ 00600 uint16_t y /* MCU position in the image (top of the MCU) */ 00601 ); 00602 00603 int16_t bitext ( /* >=0: extracted data, <0: error code */ 00604 JDEC * jd, /* Pointer to the decompressor object */ 00605 uint16_t nbit /* Number of bits to extract (1 to 11) */ 00606 ); 00607 00608 int16_t huffext ( /* >=0: decoded data, <0: error code */ 00609 JDEC * jd, /* Pointer to the decompressor object */ 00610 const uint8_t * hbits, /* Pointer to the bit distribution table */ 00611 const uint16_t * hcode, /* Pointer to the code word table */ 00612 const uint8_t * hdata /* Pointer to the data table */ 00613 ); 00614 00615 JRESULT restart ( 00616 JDEC * jd, /* Pointer to the decompressor object */ 00617 uint16_t rstn /* Expected restert sequense number */ 00618 ); 00619 00620 JRESULT mcu_load ( 00621 JDEC * jd /* Pointer to the decompressor object */ 00622 ); 00623 00624 gif_screen_descriptor_t screen_descriptor; // attributes for the whole screen 00625 bool screen_descriptor_isvalid; // has been read 00626 color_t * global_color_table; 00627 int global_color_table_size; 00628 color_t * local_color_table; 00629 int local_color_table_size; 00630 RetCode_t GetGIFHeader(FILE * fh); 00631 bool hasGIFHeader(FILE * fh); 00632 int process_gif_extension(FILE * fh); 00633 RetCode_t process_gif_image_descriptor(FILE * fh, uint8_t ** uncompress_gifed_data, int width, int height); 00634 int read_gif_sub_blocks(FILE * fh, unsigned char **data); 00635 RetCode_t uncompress_gif(int code_length, const unsigned char *input, int input_length, unsigned char *out); 00636 size_t read_filesystem_bytes(void * buffer, int numBytes, FILE * fh); 00637 RetCode_t readGIFImageDescriptor(FILE * fh, gif_image_descriptor_t * gif_image_descriptor); 00638 RetCode_t readColorTable(color_t * colorTable, int colorTableSize, FILE * fh); 00639 00640 00641 protected: 00642 /// Pure virtual method to write a boolean stream to the display. 00643 /// 00644 /// This takes a bit stream in memory and using the current color settings 00645 /// it will stream it to the display. Along the way, each bit is translated 00646 /// to either the foreground or background color value and then that pixel 00647 /// is pushed onward. 00648 /// 00649 /// This is similar, but different, to the @ref pixelStream API, which is 00650 /// given a stream of color values. 00651 /// 00652 /// @param[in] x is the horizontal position on the display. 00653 /// @param[in] y is the vertical position on the display. 00654 /// @param[in] w is the width of the rectangular region to fill. 00655 /// @param[in] h is the height of the rectangular region to fill. 00656 /// @param[in] boolStream is the inline memory image from which to extract 00657 /// the bitstream. 00658 /// @returns @ref RetCode_t value. 00659 /// 00660 virtual RetCode_t booleanStream(loc_t x, loc_t y, dim_t w, dim_t h, const uint8_t * boolStream) = 0; 00661 00662 00663 const unsigned char * font; ///< reference to an external font somewhere in memory 00664 00665 // pixel location 00666 short _x; ///< keeps track of current X location 00667 short _y; ///< keeps track of current Y location 00668 00669 uint8_t fontScaleX; ///< tracks the font scale factor for Soft fonts. Range: 1 .. 4 00670 uint8_t fontScaleY; ///< tracks the font scale factor for soft fonts. Range: 1 .. 4 00671 00672 rect_t windowrect; ///< window commands are held here for speed of access 00673 }; 00674 00675 #endif 00676
Generated on Tue Jul 26 2022 07:46:24 by
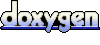