
Dependencies: PID Serialservo TCS3200 XQ_servo encoder encoder2 mbed moter
Fork of nhk_2018_undercarry_test09 by
main.cpp
00001 #include "mbed.h" 00002 #include "config.h" 00003 #include "moter.h" 00004 #include "PID.h" 00005 #include "encoder.h" 00006 #include "encoder2.h" 00007 #include "Serialservo.h" 00008 #include "XQ_servo.h" 00009 00010 Serial pc(USBTX, USBRX); 00011 Serial kbtpc(PC_12 ,PD_2);//serial5 00012 I2C master(sda, scl); 00013 DigitalOut led2(LED2);//チェック用 00014 PID S_pid(SP, SI, SD, 0.0); 00015 PID R_pid(RP, RI, RD, 0.0); 00016 PID L_pid(LP, LI, LD, 0.0); 00017 00018 Encoder Enc_Carry_Y(PA_15,PA_14,PC_1); 00019 Encoder Enc_Carry_X(PA_4,PB_0,PB_7); 00020 Encoder2 Enc_Arm(PF_1,PA_0,PA_1); 00021 00022 DigitalOut buzzer(PA_10); 00023 DigitalOut lamp(PC_3); 00024 Timer timer; 00025 Serialservo servo1(PC_10, PC_11);//アームの肘 00026 Serialservo servo2(PC_10, PC_11);//回転軸 00027 XQ_servo servo_hand(PB_5); 00028 DigitalOut throwing_relay(PC_9); 00029 DigitalIn distance(PC_2); 00030 DigitalIn redIn(PC_4); 00031 DigitalIn blueIn(PB_2); 00032 DigitalIn micro_switch(PC_0); 00033 DigitalOut air(PB_4); 00034 00035 DigitalOut motor_dir1(PA_8); 00036 DigitalOut motor_dir2(PA_9); 00037 PwmOut motor_pwm(PB_3); 00038 00039 PwmOut pwm_pins[] = { 00040 PwmOut( PC_8 ), 00041 PwmOut( PC_6 ), 00042 PwmOut( PB_14 ), 00043 PwmOut( PB_13 ) 00044 }; 00045 00046 DigitalOut dir_pins_1[] = { 00047 DigitalOut( PC_5 ), 00048 DigitalOut( PA_7 ), 00049 DigitalOut( PB_6 ), 00050 DigitalOut( PB_1) 00051 }; 00052 00053 DigitalOut dir_pins_2[] = { 00054 DigitalOut( PB_12 ), 00055 DigitalOut( PA_6 ), 00056 DigitalOut( PC_7 ), 00057 DigitalOut( PB_15) 00058 }; 00059 00060 00061 void linecheck(char *buff ,int data[2]); 00062 void LineCheck(int dmode); 00063 void Linecount(void); 00064 void syokikanomai(void); 00065 void syokikanomai2(void); 00066 void touteki(float armpower, int pos, bool thro_mode); 00067 void yellow_throwing(void); 00068 void Linecount2(void); 00069 00070 bool set_pos_flag_2 = false; 00071 bool set_pos_flag = false; 00072 bool redthrowing = false; 00073 bool yellow_throw_flag = false; 00074 bool taiki_flag = false; 00075 int crosscount = 0; 00076 bool mode = true;//trueでラジコン、falseでオート 00077 bool stopflag = true;//trueで機体停止 00078 double power = POWER; 00079 int kbtread = 0; 00080 int count = 0; 00081 int counter = 0; 00082 int linedata_1[2] = {0}; 00083 int linedata_2[2] = {0}; 00084 int linedata_3[2] = {0}; 00085 int prelinedata = 0; 00086 double output = 0.0; 00087 char buff1[2]; 00088 char buff2[2]; 00089 char buff3[2]; 00090 float x_dist = 0.0; 00091 float y_dist = 0.0; 00092 float tar_x_dist = 0.0; 00093 float tar_x_dist_2 = 0.0; 00094 float tar_x_dist_3 = 0.0; 00095 float tar_y_dist = 0.0; 00096 bool ontheline = false; 00097 int directmode = STRAIGHT; 00098 int state = START; 00099 int times = 0; 00100 int redcount = 0; 00101 int bluecount = 0; 00102 int yellowcount = 0; 00103 bool syokika_flag=false; 00104 bool set_flag = false; 00105 int encoder_count = 1000; 00106 int linecountcheck = false; 00107 00108 int main() { 00109 timer.reset(); 00110 timer.stop(); 00111 throwing_relay=0; 00112 lamp=1; 00113 air=0.0; 00114 buzzer = 0.0; 00115 micro_switch.mode(PullUp); 00116 motor_pwm=0; 00117 motor_dir1=1; 00118 motor_dir2=1; 00119 servo1.init(0);//アーム 00120 servo2.init(1);//回転軸 00121 S_pid.init(); 00122 R_pid.init(); 00123 L_pid.init(); 00124 pwm_pins[0].period(0.00005); 00125 pwm_pins[1].period(0.00005); 00126 pwm_pins[2].period(0.00005); 00127 pwm_pins[3].period(0.00005); 00128 motor_pwm.period(0.00005); 00129 //------通信スピード---------------------------------------------- 00130 pc.baud(115200); 00131 kbtpc.baud(9600); 00132 master.frequency(100000); 00133 lamp = 1.0; 00134 //-------初期化------------------------------------------------- 00135 syokikanomai();//初期位置 00136 buzzer = 0; 00137 //-----------メインループ---------------------------------------- 00138 while (true) { 00139 if(directmode == STRAIGHT){ 00140 master.read(addr1,buff1,2); 00141 linecheck(buff1,linedata_1); 00142 }else if(directmode == RIGHT){ 00143 master.read(addr2,buff2,2); 00144 linecheck(buff2,linedata_2); 00145 }else if(directmode == LEFT){ 00146 master.read(addr3,buff3,2); 00147 linecheck(buff3,linedata_3); 00148 } 00149 00150 x_dist = Enc_Carry_X.read_rotate(); 00151 y_dist = Enc_Carry_Y.read_rotate(); 00152 00153 //PCからの命令 00154 kbtpc.putc(state); 00155 if(kbtpc.readable()){ 00156 kbtread = kbtpc.getc(); 00157 } 00158 if(kbtread == 255){ 00159 mode = true;// 00160 }else if(kbtread == 150){ 00161 mode = false; 00162 }else if(kbtread == 100){ 00163 stopflag = true; 00164 }else if(kbtread == 200){ 00165 stopflag = false; 00166 }else if(kbtread == STRAIGHT){ 00167 directmode = STRAIGHT; 00168 }else if(kbtread == RIGHT){ 00169 directmode = RIGHT; 00170 }else if(kbtread == LEFT){ 00171 directmode = LEFT; 00172 }else if(kbtread == START){ 00173 state = START; 00174 }else if(kbtread == STAND_BY_1){ 00175 state = STAND_BY_1; 00176 }else if(kbtread == YELLOW_RECEIVE){ 00177 state = YELLOW_RECEIVE; 00178 crosscount = 5; 00179 air = 1.0; 00180 }else if(kbtread == STAND_BY_2){ 00181 state = STAND_BY_2; 00182 crosscount = 0;; 00183 } 00184 00185 //--------------手動-------------------------------------- 00186 if(mode == true){ 00187 led2 = 1.0; 00188 if(kbtread == STRAIGHT){ 00189 Straight(power, power, pwm_pins, dir_pins_1, dir_pins_2); 00190 }else if(kbtread == BACK){ 00191 Back(power, power, pwm_pins, dir_pins_1, dir_pins_2); 00192 }else if(kbtread == RIGHT){ 00193 Right(power, power, pwm_pins, dir_pins_1, dir_pins_2); 00194 }else if(kbtread == LEFT){ 00195 Left(power, power, pwm_pins, dir_pins_1, dir_pins_2); 00196 }else{ 00197 Stop(pwm_pins, dir_pins_1, dir_pins_2); 00198 } 00199 //--------------自動--------------------------------- 00200 }else if(mode == false){ 00201 led2 = 0.0; 00202 if(stopflag == true){ 00203 Stop(pwm_pins, dir_pins_1, dir_pins_2); 00204 }else{ 00205 LineCheck(directmode); 00206 switch(state){ 00207 //------------------初期位置から待機位置1------------------------------------------ 00208 case START: 00209 if(crosscount == 0){ 00210 directmode = LEFT; 00211 Linecount2(); 00212 }else if(crosscount == 1){ 00213 directmode = STRAIGHT; 00214 }else if(crosscount == 2){ 00215 if(directmode == STRAIGHT){ 00216 directmode = LEFT; 00217 prelinedata = 0; 00218 }else if(directmode == LEFT){ 00219 directmode = LEFT; 00220 Linecount(); 00221 } 00222 }else if(crosscount == 3){ 00223 directmode = STOP; 00224 state = STAND_BY_1; 00225 crosscount = 0; 00226 prelinedata = 0; 00227 } 00228 break; 00229 //------------------待機位置1---------------------------------------------------- 00230 case STAND_BY_1: 00231 crosscount = 0; 00232 directmode = STOP; 00233 00234 if(distance == 1.0){ 00235 if(redthrowing == false){ 00236 if(redIn == 1.0){ 00237 buzzer = 1.0; 00238 redcount++; 00239 }else { 00240 buzzer = 0.0; 00241 } 00242 }else if(redthrowing == true){ 00243 if(redIn == 1.0){ 00244 buzzer = 1.0; 00245 bluecount++; 00246 }else { 00247 buzzer = 0.0; 00248 } 00249 } 00250 if(redcount >= 200 ){ 00251 buzzer = 0; 00252 servo_hand.move(hand_tsukami_degree); 00253 servo1.move(hiji_tsukami_degree); 00254 servo1.move(hiji_tsukami_degree); 00255 }else if(bluecount >= 200){ 00256 servo_hand.move(hand_tsukami_degree); 00257 servo1.move(hiji_tsukami_degree); 00258 servo1.move(hiji_tsukami_degree); 00259 } 00260 } 00261 if(distance == 0.0 && redcount >= 200){ 00262 led2 = 1.0; 00263 servo2.move(koshi_red_nage_degree); 00264 servo2.move(koshi_red_nage_degree); 00265 state = RED_RECEIVE; 00266 directmode = STOP; 00267 redcount = 0; 00268 crosscount = 0; 00269 }else if(distance == 0.0 && bluecount >= 200){ 00270 servo2.move(koshi_blue_nage_degree); 00271 servo2.move(koshi_blue_nage_degree); 00272 state = BLUE_RECEIVE_1; 00273 directmode = STOP; 00274 bluecount = 0; 00275 crosscount = 0; 00276 } 00277 break; 00278 //------------------待機位置1から投てき位置1---------------------------------------- 00279 case RED_RECEIVE: 00280 led2 = 1.0; 00281 if(crosscount == 0){ 00282 directmode = LEFT; 00283 }else if(crosscount == 1){ 00284 directmode = STOP; 00285 prelinedata = 0; 00286 touteki(1.0,RED_TOUTEKI,0); 00287 //syokikanomai2(); 00288 if(set_flag == false){ 00289 directmode = RIGHT; 00290 Linecount(); 00291 } 00292 }else if(crosscount == 2){ 00293 directmode = STOP; 00294 syokikanomai2(); 00295 if(set_flag == true){ 00296 state = STAND_BY_1; 00297 //taiki_flag = false; 00298 crosscount = 0; 00299 redthrowing = true; 00300 } 00301 } 00302 break; 00303 //------------------待機位置1から投てき位置2---------------------------------------- 00304 case BLUE_RECEIVE_1: 00305 if(crosscount == 0){ 00306 directmode = RIGHT; 00307 }else if(crosscount == 1){ 00308 directmode = STRAIGHT; 00309 }else if(crosscount == 2){ 00310 directmode = LEFT; 00311 Linecount(); 00312 }else if(crosscount == 3){ 00313 directmode = STOP; 00314 prelinedata = 0; 00315 state = BLUE_RECEIVE_2; 00316 crosscount = 0; 00317 } 00318 break; 00319 //------------------待機位置2---------------------------------------------------- 00320 case STAND_BY_2: 00321 crosscount = 0; 00322 directmode = STOP; 00323 if(distance == 1.0){ 00324 if(redIn == 1.0 && micro_switch == 1){ 00325 buzzer = 1.0; 00326 bluecount++; 00327 }else if(micro_switch == 0.0){ 00328 buzzer = 1.0; 00329 yellowcount++; 00330 }else{ 00331 buzzer = 0.0; 00332 } 00333 if(bluecount >= 200){ 00334 servo_hand.move(hand_tsukami_degree); 00335 servo1.move(hiji_tsukami_degree); 00336 servo1.move(hiji_tsukami_degree); 00337 }else if(yellowcount >= 500){ 00338 air = 1.0; 00339 } 00340 }else if(distance == 0.0 && bluecount >= 200){ 00341 servo2.move(koshi_blue_nage_degree); 00342 servo2.move(koshi_blue_nage_degree); 00343 state = BLUE_RECEIVE_2; 00344 directmode = STOP; 00345 bluecount = 0; 00346 crosscount = 0; 00347 buzzer = 0.0; 00348 } 00349 if(yellowcount >= 1000){ 00350 state = YELLOW_RECEIVE; 00351 buzzer = 0.0; 00352 directmode = STOP; 00353 yellowcount = 0; 00354 crosscount = 0; 00355 } 00356 break; 00357 //------------------投てき位置2--------------------------------------------------- 00358 case BLUE_RECEIVE_2: 00359 if(crosscount == 0){ 00360 directmode = LEFT; 00361 }else if(crosscount == 1){ 00362 directmode = STOP; 00363 prelinedata = 0; 00364 touteki(1.0,BLUE_TOUTEKI,1); 00365 //syokikanomai2(); 00366 if(set_flag == false){ 00367 directmode = RIGHT; 00368 Linecount(); 00369 } 00370 }else if(crosscount == 2){ 00371 directmode = STOP; 00372 syokikanomai2(); 00373 if(set_flag == true){ 00374 state = STAND_BY_2; 00375 //taiki_flag = false; 00376 crosscount = 0; 00377 } 00378 } 00379 break; 00380 //------------------投てき位置3--------------------------------------------------- 00381 case YELLOW_RECEIVE: 00382 if(crosscount != 5){ 00383 directmode = LEFT; 00384 servo2.move(koshi_uke_degree); 00385 power = POWER + 0.1; 00386 }else{ 00387 directmode = STOP; 00388 if(yellow_throw_flag == false){ 00389 yellow_throwing(); 00390 }else{ 00391 touteki(1.0,YELLOW_TOUTEKI,1.0); 00392 syokikanomai2(); 00393 } 00394 } 00395 break; 00396 default: 00397 break; 00398 } 00399 //--------------進行方向--------------------------------------------------------- 00400 switch(directmode){ 00401 case STOP: 00402 Stop(pwm_pins, dir_pins_1, dir_pins_2); 00403 break; 00404 case STRAIGHT: 00405 output = S_pid.compute((double)linedata_1[0]); 00406 if(output >= 0){ 00407 Straight(power, power + output, pwm_pins, dir_pins_1, dir_pins_2); 00408 }else { 00409 Straight(power + (-1 * output), power, pwm_pins, dir_pins_1, dir_pins_2); 00410 } 00411 break; 00412 case RIGHT: 00413 output = R_pid.compute((double)linedata_2[0]); 00414 if(output >= 0){ 00415 Right(power, power + output, pwm_pins, dir_pins_1, dir_pins_2); 00416 }else { 00417 Right(power + (-1 * output), power, pwm_pins, dir_pins_1, dir_pins_2); 00418 } 00419 break; 00420 case LEFT: 00421 output = L_pid.compute((double)linedata_3[0]); 00422 if(output >= 0){ 00423 Left(power, power + output, pwm_pins, dir_pins_1, dir_pins_2); 00424 }else { 00425 Left(power + (-1 * output), power, pwm_pins, dir_pins_1, dir_pins_2); 00426 } 00427 break; 00428 default: 00429 break; 00430 } 00431 } 00432 } 00433 pc.printf("xdist = %f \r",x_dist); 00434 pc.printf("ydist = %f \r",y_dist); 00435 pc.printf("output = %lf \n" ,output); 00436 } 00437 } 00438 00439 //---------------初期化---------------------------------------------------------- 00440 00441 void yellow_throwing(){ 00442 if(yellow_throw_flag == false){ 00443 timer.start(); 00444 } 00445 int times = timer.read(); 00446 if(times >= 2.0 && times < 3.0){ 00447 servo_hand.move(hand_uke_degree); 00448 }else if(times >= 3.0 && times < 4.0){ 00449 servo1.move(hiji_yellow_uke_degree); 00450 }else if(times >= 4.0 && times < 5.0){ 00451 servo_hand.move(hand_tsukami_degree); 00452 }else if(times >=5.0 && times <5.5){ 00453 servo2.move(koshi_nage_degree); 00454 }else if(times >= 5.5){ 00455 yellow_throw_flag = true; 00456 timer.stop(); 00457 timer.reset(); 00458 } 00459 } 00460 00461 void syokikanomai(void){ 00462 syokika_flag = false; 00463 while (set_flag==false) { 00464 encoder_count= Enc_Arm.read_rotate(); 00465 servo_hand.move(hand_uke_degree); 00466 servo1.move(hiji_nage_degree);//退避 00467 servo2.move(koshi_uke_degree); 00468 servo1.move(hiji_nage_degree);//退避 00469 servo2.move(koshi_uke_degree); 00470 motor_dir1=0; 00471 motor_dir2=1; 00472 motor_pwm=0.12; 00473 if(Enc_Arm.read_z() == 1){ 00474 syokika_flag=true; 00475 buzzer = 1.0; 00476 } 00477 00478 if (syokika_flag==true) { 00479 if(encoder_count == SYOKIITI){ 00480 motor_pwm=0; 00481 motor_dir1=1; 00482 motor_dir2=1; 00483 set_flag=true; //投てき後,falseにする. 00484 } 00485 } 00486 } 00487 servo1.move(hiji_uke_degree); 00488 servo1.move(hiji_uke_degree); 00489 } 00490 00491 void syokikanomai2(void){ 00492 if(set_flag==false) { 00493 encoder_count= Enc_Arm.read_rotate(); 00494 servo_hand.move(hand_uke_degree); 00495 servo1.move(hiji_nage_degree);//退避 00496 servo1.move(hiji_nage_degree);//退避 00497 if(yellow_throw_flag == false){ 00498 servo2.move(koshi_uke_degree); 00499 servo2.move(koshi_uke_degree); 00500 } 00501 motor_dir1=0; 00502 motor_dir2=1; 00503 motor_pwm=0.12; 00504 if(Enc_Arm.read_z() == 1) { 00505 syokika_flag = true; 00506 buzzer = 1.0; 00507 } 00508 if(syokika_flag==true) { 00509 int set_pos; 00510 if(yellow_throw_flag == true) set_pos = YELLOW_SYOKIITI; 00511 else set_pos = SYOKIITI; 00512 if(encoder_count == set_pos){ 00513 buzzer = 0.0; 00514 motor_pwm=0; 00515 motor_dir1=1; 00516 motor_dir2=1; 00517 if(yellow_throw_flag == false){ 00518 servo_hand.move(hand_uke_degree); 00519 servo1.move(hiji_uke_degree); 00520 servo1.move(hiji_uke_degree); 00521 servo1.move(hiji_uke_degree); 00522 servo1.move(hiji_uke_degree); 00523 }else{ 00524 servo_hand.move(hand_tsukami_degree); 00525 servo2.move(koshi_uke_degree); 00526 servo2.move(koshi_uke_degree); 00527 } 00528 set_flag = true; //投てき後,falseにする. 00529 taiki_flag = true; 00530 yellow_throw_flag = false; 00531 } 00532 } 00533 } 00534 } 00535 00536 void touteki(float armpower ,int pos, bool throwing_mode){ 00537 if(set_flag == true){ 00538 timer.start(); 00539 } 00540 int times = timer.read(); 00541 if(times >= 1.0 && times < 4.0){ 00542 if(yellow_throw_flag == true){ 00543 if(set_pos_flag_2 == false){ 00544 if(Enc_Arm.read_rotate() < 180) { 00545 motor_dir1 = 0; 00546 motor_dir2 = 1; 00547 motor_pwm = 0.15; 00548 }else{ 00549 motor_dir1=1; 00550 motor_dir2=1; 00551 motor_pwm=0; 00552 set_pos_flag_2 = true; 00553 } 00554 } 00555 } 00556 } 00557 if(times >= 4.0 && times < 5.0){ 00558 servo_hand.move(hand_uke_degree); 00559 } 00560 if(times >= 5.0 && times < 6.0){ 00561 servo1.move(hiji_nage_degree); 00562 servo1.move(hiji_nage_degree); 00563 } 00564 if(times >= 6.0 && times < 9.0){ 00565 lamp = 0.0; 00566 if(set_pos_flag == false){ 00567 if(Enc_Arm.read_rotate() < pos) { 00568 motor_dir1 = 0; 00569 motor_dir2 = 1; 00570 motor_pwm = 0.15; 00571 }else{ 00572 motor_dir1=1; 00573 motor_dir2=1; 00574 motor_pwm=0; 00575 set_pos_flag = true; 00576 } 00577 } 00578 } 00579 00580 //----投てき------------------------- 00581 if(throwing_mode == 0.0){ 00582 if(times >= 9.0 && times < 10.0){ 00583 motor_dir1 = 0; 00584 motor_dir2 = 1; 00585 motor_pwm = armpower; 00586 } 00587 }else if(throwing_mode == 1.0){ 00588 if(times >= 9.0 && times < 9.1){ 00589 throwing_relay = 1.0; 00590 }else{ 00591 throwing_relay = 0.0; 00592 } 00593 } 00594 if(times >= 10.0 && times <11.0){ 00595 motor_pwm = 0.0; 00596 motor_dir1 = 1; 00597 motor_dir2 = 1; 00598 buzzer = 0; 00599 lamp = 1.0; 00600 syokika_flag = false; 00601 }else if(times >= 11.0){ 00602 set_flag = false; 00603 set_pos_flag = false; 00604 set_pos_flag_2 = false; 00605 timer.reset(); 00606 timer.stop(); 00607 } 00608 } 00609 00610 void Linecount(void){ 00611 if(directmode == RIGHT){ 00612 if(linecountcheck == false){ 00613 tar_x_dist_2 = x_dist; 00614 linecountcheck = true; 00615 }else{ 00616 if((x_dist - tar_x_dist_2) > X_STOP_DIST_3){ 00617 crosscount++; 00618 linecountcheck = false; 00619 } 00620 } 00621 }else if(directmode == LEFT){ 00622 if(linecountcheck == false){ 00623 tar_x_dist_2 = x_dist; 00624 linecountcheck = true; 00625 }else{ 00626 if((tar_x_dist_2 - x_dist) >= X_STOP_DIST_2){ 00627 crosscount++; 00628 linecountcheck = false; 00629 } 00630 } 00631 } 00632 } 00633 00634 void Linecount2(void){ 00635 if(directmode == LEFT){ 00636 if(linecountcheck == false){ 00637 tar_x_dist_3 = x_dist; 00638 linecountcheck = true; 00639 }else{ 00640 if((tar_x_dist_3 - x_dist) >= X_STOP_DIST_4){ 00641 crosscount++; 00642 linecountcheck = false; 00643 } 00644 } 00645 } 00646 } 00647 void LineCheck(int dmode){ 00648 if(dmode == STRAIGHT){ 00649 if(linedata_1[1] == 1){ 00650 ontheline = true; 00651 tar_y_dist = y_dist; 00652 } 00653 if(ontheline == true){ 00654 if((y_dist - tar_y_dist) >= Y_STOP_DIST){ 00655 ontheline = false; 00656 crosscount++; 00657 } 00658 } 00659 }else if(dmode == RIGHT){ 00660 if(linedata_2[1] == 1){ 00661 ontheline = true; 00662 tar_x_dist = x_dist; 00663 } 00664 if(ontheline == true){ 00665 if((x_dist - tar_x_dist) >= X_STOP_DIST){ 00666 ontheline = false; 00667 crosscount++; 00668 } 00669 } 00670 }else if(dmode == LEFT){ 00671 if(linedata_3[1] == 1){ 00672 ontheline = true; 00673 tar_x_dist = x_dist; 00674 } 00675 if(ontheline == true){ 00676 if((tar_x_dist - x_dist) >= X_STOP_DIST){ 00677 ontheline = false; 00678 crosscount++; 00679 } 00680 } 00681 } 00682 } 00683 00684 void linecheck(char *buff, int data[2]){ 00685 if(buff[0] == 24){ 00686 data[0] = 0; 00687 data[1] = 0; 00688 }else if(buff[0] == 56){ 00689 data[0] = 1; 00690 data[1] = 0; 00691 }else if(buff[0] == 48){ 00692 data[0] = 2; 00693 data[1] = 0; 00694 }else if(buff[0] == 112){ 00695 data[0] = 3; 00696 data[1] = 0; 00697 }else if(buff[0] == 96){ 00698 data[0] = 4; 00699 data[1] = 0; 00700 }else if(buff[0] == 224){ 00701 data[0] = 5; 00702 data[1] = 0; 00703 }else if(buff[0] == 192){ 00704 data[0] = 6; 00705 data[1] = 0; 00706 }else if(buff[0] == 128){ 00707 data[0] = 7; 00708 data[1] = 0; 00709 }else if(buff[0] == 28){ 00710 data[0] = -1; 00711 data[1] = 0; 00712 }else if(buff[0] == 12){ 00713 data[0] = -2; 00714 data[1] = 0; 00715 }else if(buff[0] == 14){ 00716 data[0] = -3; 00717 data[1] = 0; 00718 }else if(buff[0] == 6){ 00719 data[0] = -4; 00720 data[1] = 0; 00721 }else if(buff[0] == 7){ 00722 data[0] = -5; 00723 data[1] = 0; 00724 }else if(buff[0] == 3){ 00725 data[0] = -6; 00726 data[1] = 0; 00727 }else if(buff[0] == 1){ 00728 data[0] = -7; 00729 data[1] = 0; 00730 }else if(buff[0] == 255){ 00731 data[0] = prelinedata; 00732 data[1] = 1; 00733 }else if(buff[0] == 0){ 00734 data[0] = prelinedata; 00735 data[1] = 0; 00736 }else{ 00737 data[0] = prelinedata; 00738 data[1] = 0; 00739 } 00740 prelinedata = data[0]; 00741 }
Generated on Sun Jul 31 2022 14:34:49 by
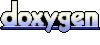