Enter Low Power mode not to use sd_power_system_off() subroutine
Dependents: BLE_EddystoneBeacon_w_ACC_TY51822 BLE_LoopbackUART_low_pwr_w_RTC1 BLE_Paired_Server BLE_Paired_Client
nRF51_lowpwr.cpp
00001 /* 00002 * mbed library program 00003 * Set low power condition only for nRF51822 BLE chip 00004 * 00005 * Copyright (c) 2016 Kenji Arai / JH1PJL 00006 * http://www.page.sannet.ne.jp/kenjia/index.html 00007 * http://mbed.org/users/kenjiArai/ 00008 * Started: April 30th, 2016 00009 * Revised: June 11th, 2016 00010 * 00011 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, 00012 * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00013 * AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00014 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00015 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00016 */ 00017 00018 #include "mbed.h" 00019 #include "nRF51_lowpwr.h" 00020 #include "nrf_delay.h" 00021 00022 LowPwr::LowPwr(const nRF51_LOWPWR_TypeDef *param){ 00023 if ((param->std_printf_function == false) && (param->serial_function == false)){ 00024 //#warning "Disable Serial function (cannot use printf)!" 00025 NRF_UART0->ENABLE = 0; 00026 nrf_delay_us(5); 00027 NRF_UART0->EVENTS_RXDRDY = 0; 00028 nrf_delay_us(5); 00029 //NRF_UART0->INTENCLR = 0x00020287; 00030 NRF_UART0->INTENCLR = 0xffffffff; 00031 nrf_delay_us(5); 00032 NRF_UART0->TASKS_STOPRX = 1; 00033 nrf_delay_us(5); 00034 NRF_UART0->POWER = 0; 00035 nrf_delay_us(5); 00036 } 00037 if (param->i2c_function == false){ 00038 //#warning "Disable I2C function!" 00039 NRF_TWI0->EVENTS_ERROR = 0; 00040 nrf_delay_us(5); 00041 NRF_TWI0->INTENCLR = 0xffffffff; 00042 nrf_delay_us(5); 00043 NRF_TWI0->ENABLE = 0; 00044 nrf_delay_us(5); 00045 NRF_TWI0->POWER = 0; 00046 nrf_delay_us(5); 00047 NRF_TWI1->EVENTS_ERROR = 0; 00048 nrf_delay_us(5); 00049 NRF_TWI1->INTENCLR = 0xffffffff; 00050 nrf_delay_us(5); 00051 NRF_TWI1->ENABLE = 0; 00052 nrf_delay_us(5); 00053 NRF_TWI1->POWER = 0; 00054 } 00055 if ((param->spi_function == false) && (param->spi_slave_function == false)){ 00056 //#warning "Disable SPI function!" 00057 NRF_SPI0->INTENCLR = 0xffffffff; 00058 nrf_delay_us(5); 00059 NRF_SPI0->ENABLE = 0; 00060 nrf_delay_us(5); 00061 NRF_SPI0->POWER = 0; 00062 nrf_delay_us(5); 00063 NRF_SPI1->INTENCLR = 0xffffffff; 00064 nrf_delay_us(5); 00065 NRF_SPI1->ENABLE = 0; 00066 nrf_delay_us(5); 00067 NRF_SPI1->POWER = 0; 00068 nrf_delay_us(5); 00069 NRF_SPIS1->INTENCLR = 0xffffffff; 00070 nrf_delay_us(5); 00071 NRF_SPIS1->ENABLE = 0; 00072 nrf_delay_us(5); 00073 NRF_SPIS1->POWER = 0; 00074 } 00075 if (param->pwm_functions == false){ 00076 //#warning "Disable PWM function!" 00077 // Timer0,1,2 00078 NRF_TIMER0->INTENCLR = 0xffffffff; 00079 nrf_delay_us(5); 00080 NRF_TIMER0->TASKS_STOP = 1; 00081 nrf_delay_us(5); 00082 NRF_TIMER0->POWER = 0; 00083 nrf_delay_us(5); 00084 NRF_TIMER1->INTENCLR = 0xffffffff; 00085 nrf_delay_us(5); 00086 NRF_TIMER1->TASKS_STOP = 1; 00087 nrf_delay_us(5); 00088 NRF_TIMER1->POWER = 0; 00089 nrf_delay_us(5); 00090 NRF_TIMER2->INTENCLR = 0xffffffff; 00091 nrf_delay_us(5); 00092 NRF_TIMER2->TASKS_STOP = 1; 00093 nrf_delay_us(5); 00094 NRF_TIMER2->POWER = 0; 00095 nrf_delay_us(5); 00096 // PPI 00097 NRF_PPI->TASKS_CHG[0].DIS = 1; 00098 NRF_PPI->TASKS_CHG[1].DIS = 1; 00099 NRF_PPI->TASKS_CHG[2].DIS = 1; 00100 NRF_PPI->TASKS_CHG[3].DIS = 1; 00101 for (uint8_t n = 0; n <16 ; n++){ 00102 NRF_PPI->CH[n].TEP = 0; 00103 NRF_PPI->CH[n].EEP = 0; 00104 } 00105 } 00106 if (param->analog_function == false){ 00107 //#warning "Disable ADC function!" 00108 NRF_ADC->TASKS_STOP = 1; 00109 nrf_delay_us(5); 00110 NRF_ADC->CONFIG = 0x18; 00111 nrf_delay_us(5); 00112 NRF_ADC->ENABLE = 0; 00113 nrf_delay_us(5); 00114 NRF_ADC->POWER = 0; 00115 nrf_delay_us(5); 00116 } 00117 }
Generated on Mon Aug 22 2022 20:34:32 by
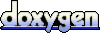