Reciprocal Frequency counter only for STM32F401、F411 and F466. Reciprocal Mode -> Pulse width measurement
Dependents: Frequency_Counter_Recipro_for_STM32F4xx
fc_recipro.h
00001 /* 00002 * mbed Library / Frequency Counter / Recipro type 00003 * Frequency Counter program 00004 * Only for Nucleo-F401RE,-F411RE,-F446RE 00005 * 00006 * Copyright (c) 2014,'15,'16,'20 Kenji Arai / JH1PJL 00007 * http://www7b.biglobe.ne.jp/~kenjia/ 00008 * https://os.mbed.com/users/kenjiArai/ 00009 * Started: October 18th, 2014 00010 * Revised: January 19th, 2020 00011 * 00012 */ 00013 00014 #ifndef MBED_FRQ_CUNTR 00015 #define MBED_FRQ_CUNTR 00016 00017 #include "mbed.h" 00018 00019 #define DEBUG 0 // use Communication with PC(UART) & port output 00020 00021 typedef struct { 00022 float freq_rise2rise; 00023 float freq_fall2fall; 00024 float time_us_rise2fall; 00025 float time_us_fall2rise; 00026 } Recipro_result_TypeDef; 00027 00028 typedef struct { 00029 uint8_t rise_cnt; 00030 uint8_t fall_cnt; 00031 uint8_t input_level; 00032 float passed_time; 00033 } Recipro_status_TypeDef; 00034 00035 namespace Frequency_counter 00036 { 00037 /** Frequency Counter program 00038 * Only for Nucleo-F411RE & F446RE 00039 * 00040 * @code 00041 * #include "mbed.h" 00042 * #include "fc_recipro.h" 00043 * 00044 * using namespace Frequency_counter; 00045 * 00046 * // frequency input -> PA_0 & PA_1 00047 * // PA_0(for reciprocal) Timer2 Input Capture #1 00048 * // PA_1(for reciprocal) Timer2 Input Capture #2 00049 * 00050 * FRQ_CUNTR fc; 00051 * 00052 * Recipro_result_TypeDef freq; 00053 * 00054 * int main() 00055 * { 00056 * //pc.printf("\r\nStart Frequency Counter\r\n"); 00057 * fc.recipro_start_measurement(); 00058 * while(true) { 00059 * t.reset(); 00060 * t.start(); 00061 * if (fc.recipro_check_status(&status) == true) { 00062 * fc.recipro_get_result(&freq); 00063 * pc.printf("freq = %.3f [Hz], time(rise_fall) = %.6f [mS]\r\n", 00064 * freq.freq_rise2rise, freq.time_us_rise2fall); 00065 * } else { 00066 * pc.printf("------ data is NOT ready -------\r\n"); 00067 * } 00068 * uint32_t pass = t.read_ms(); 00069 * if (pass < 990) { 00070 * WAIT(1000 - pass); 00071 * } 00072 * } 00073 * } 00074 * @endcode 00075 */ 00076 00077 class FRQ_CUNTR 00078 { 00079 00080 public: 00081 00082 /** Configure counter 00083 * @param pin (Fixed A0 & A1) No other choice!! 00084 */ 00085 FRQ_CUNTR(PinName pin = A0); 00086 00087 //----------- Reciprocal measurement ------------------------------------------- 00088 /** start Reciprocal measurement 00089 * @param none 00090 * @return none 00091 */ 00092 void recipro_start_measurement(void); 00093 00094 /** stop Reciprocal measurement 00095 * @param none 00096 * @return none 00097 */ 00098 void recipro_stop_measurement(void); 00099 00100 /** check status 00101 * @param pointer for saving status 00102 * @return ready = true, not ready = false 00103 */ 00104 bool recipro_check_status(Recipro_status_TypeDef *status); 00105 00106 /** read data 00107 * @param pointer for saving data 00108 * @return none 00109 */ 00110 void recipro_get_result(Recipro_result_TypeDef *fq); 00111 00112 /** read raw data 00113 * @param pointer for saving raw data 00114 * @return none 00115 */ 00116 void recipro_get_raw_data(int64_t *buf); 00117 00118 /** Check input frequency on TIM2 00119 * @param none 00120 * @return frequency data 00121 */ 00122 uint32_t read_base_clock_frequency(void); 00123 00124 /** Check overflow counter data on TIM2 00125 * @param none 00126 * @return overflow data 00127 */ 00128 uint32_t read_tm2_overflow(void); 00129 00130 protected: 00131 Timer _t; 00132 DigitalIn _input_pin; 00133 00134 void start_action(void); // Start trigger for reciprocal 00135 void stop_action(void); // Stop reciprocal 00136 void initialize_TIM2(void); // Initialize Timer_2 (32bit) 00137 uint64_t get_diff(uint64_t new_dt, uint64_t old_dt); 00138 00139 private: 00140 int64_t _data_buf[4]; 00141 int64_t _tp0; 00142 int64_t _tp1; 00143 int64_t _tp2; 00144 int64_t _tp3; 00145 00146 float _base_clock; 00147 00148 float _freq_rise2rise; 00149 float _freq_fall2fall; 00150 float _time_us_rise2fall; 00151 float _time_us_fall2rise; 00152 float _passed_time; 00153 uint8_t _sample_num; 00154 bool _data_ready; 00155 00156 }; 00157 00158 /* 00159 Interrupt handler does NOT work following code 00160 NVIC_SetVector(TIM2_IRQn, (uint32_t)FRQ_CUNTR::irq_ic_TIM2); 00161 From this reason, I wrote below code and set interrupt handler 00162 out side "FRQ_CUNTR" class 00163 NVIC_SetVector(TIM2_IRQn, (uint32_t)irq_ic_TIM2); 00164 */ 00165 void irq_ic_TIM2(void); // TIM2(F4xx) IC1 & IC2 Interrupt 00166 00167 } // Frequency_counter 00168 00169 #endif // MBED_FRQ_CUNTR
Generated on Tue Jul 19 2022 04:30:27 by
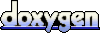