
TYBLE16 serial communication (UART) for both "Central" and "Peripheral".
Fork of TYBLE16_BME280_data_sender by
main.cpp
00001 /* 00002 * Mbed Application program / Using Akizuki BLE Module AE-TYBLE16 00003 * on-board module : TAIYO YUDEN BLE 4.2 TYSA-B (EYSGJNAWY-WX) 00004 * 00005 * http://akizukidenshi.com/catalog/g/gK-12339/ 00006 * 00007 * Refernce document 00008 * https://www.yuden.co.jp/wireless_module/document/datareport2/jp/ 00009 * TY_BLE_EYSGJNAWY_WX_BriefDataReport_V1_3_20170925J.pdf 00010 * 00011 * Copyright (c) 2017 Kenji Arai / JH1PJL 00012 * http://www.page.sannet.ne.jp/kenjia/index.html 00013 * http://mbed.org/users/kenjiArai/ 00014 * Created: October 27th, 2017 00015 * Revised: November 19th, 2017 00016 */ 00017 00018 /* 00019 Tested condition 00020 mbed-os-5.6.4 & mbed Rev.157(release 155?) 00021 Nucleo-F446RE TYBLE16 /also F411RE 00022 PA_9 (D8) pin5 / P0.03(UART_RX) 00023 PA_10(D2) pin6 / P0.01(UART_TX) 00024 pin7 / P0.02(UART_CTS) connected to pin8 / P0.00(UART_RTS) 00025 PB_3 (D3) pin15 / P0.23(MODE) Mode indication 00026 +3.3v pin14 / +V 00027 GND pin13 / GND 00028 */ 00029 00030 /* 00031 --- HOW TO ESTABLISH CENTRAL AND PERIPHERAL UART --- 00032 1) Prepare one peripheral module/TYBLE16 00033 2) Prepare another TYBLE16 module which was programed as "Central mode" 00034 Please refer "TYBLE16_set_Central_or_ Peripheral" program 00035 3) Programing "TYBLE16_Uart_Central_and_Peripheral" into Mbed boards 00036 (same program into both "Central" and "Peripheral" Mbed board) 00037 4) If you turn on both the "Central" and "Peripheral" power at the same time, 00038 the connection is automatically started 00039 */ 00040 00041 // Include -------------------------------------------------------------------- 00042 #include "mbed.h" 00043 #include "RingBuffer.h" 00044 00045 // Definition ----------------------------------------------------------------- 00046 #define TIME_OUT 5 // 5 sec 00047 #define BF_SIZE_BLE 256 00048 #define BF_SIZE_PC 256 00049 #define BF_SIZE_LINE 32 00050 00051 // Object/ Constructor -------------------------------------------------------- 00052 Serial pc(USBTX,USBRX); 00053 Serial tyble16(D8, D2); 00054 DigitalIn tyble16_mode(D3); 00055 Ticker t; 00056 RingBuffer ble_bf(BF_SIZE_BLE); 00057 RingBuffer pc_bf(BF_SIZE_PC); 00058 00059 // RAM ------------------------------------------------------------------------ 00060 00061 // ROM / Constant data -------------------------------------------------------- 00062 00063 // Function prototypes -------------------------------------------------------- 00064 void check_connection(void); 00065 void excute_command(char *); 00066 void ble_rx(void); 00067 void pc_rx(void); 00068 00069 //------------------------------------------------------------------------------ 00070 // Control Program 00071 //------------------------------------------------------------------------------ 00072 int main() 00073 { 00074 static char rcv_bf[BF_SIZE_LINE]; 00075 static uint8_t n = 0; 00076 char c = 0; 00077 00078 tyble16.attach(&ble_rx, Serial::RxIrq); // Set interrupt handler 00079 pc.attach(&pc_rx, Serial::RxIrq); // Set interrupt handler 00080 pc.printf("\r\nUART/TYBLE16(%s, %s UTC)\r\n", __DATE__, __TIME__); 00081 tyble16.printf("I'm TYBLE16\r\n"); 00082 t.attach(check_connection, 1); 00083 while(true){ 00084 while(ble_bf.check() != 0){ 00085 //----- receive data ----------------------------------------------- 00086 c = ble_bf.read(); // data received 00087 pc.putc(c); // show to console 00088 rcv_bf[n++] = c; // save int buf 00089 // avoid buffer overflow 00090 if (n >= (BF_SIZE_LINE - 5)){ n = (BF_SIZE_LINE - 5);} 00091 if (c == '\n'){ // end one line 00092 pc.putc('\r'); 00093 rcv_bf[n] = 0; 00094 if (n >3){ // command ~?\r\n ?=specified one character 00095 if ((rcv_bf[0] == '~') && (rcv_bf[2] == '\r')){ 00096 excute_command(rcv_bf); 00097 } 00098 } 00099 n = 0; // Clear buffer 00100 } 00101 } 00102 while(pc_bf.check() != 0){ 00103 //----- send data -------------------------------------------------- 00104 char c = pc_bf.read(); 00105 tyble16.putc(c); 00106 pc.putc(c); // echo back 00107 if(c == '\r'){ // if CR then put LF 00108 tyble16.putc('\n'); 00109 pc.putc('\n'); 00110 } 00111 } 00112 } 00113 } 00114 00115 // special command (You can make your own commands) 00116 void excute_command(char *buf) 00117 { 00118 char c = buf[1]; 00119 switch (c){ 00120 case 'x': // corrent -> nothing to do 00121 tyble16.printf("Accept 'x' Command\r\n"); 00122 break; 00123 case '?': // Help 00124 tyble16.printf("Command not available\r\n"); 00125 tyble16.printf("Please set your own command\r\n"); 00126 break; 00127 default: // no available command 00128 tyble16.printf("Command not available\r\n"); 00129 break; 00130 } 00131 } 00132 00133 // Interrupt routine / Data from BLE 00134 void ble_rx() 00135 { 00136 ble_bf.save(tyble16.getc()); // BLE RX data save into ring buffer 00137 } 00138 00139 // Interrupt routine / Data from PC 00140 void pc_rx() 00141 { 00142 pc_bf.save(pc.getc()); // PC RX data save into ring buffer 00143 } 00144 00145 // Every one second, check communication status 00146 void check_connection(void) 00147 { 00148 static int8_t counter = 0; 00149 00150 if (tyble16_mode == 0){ // Not connected 00151 if (++counter >= TIME_OUT){ 00152 // Software reset 00153 tyble16.puts("BRS\r\n"); 00154 counter = 0; 00155 } 00156 } else { 00157 counter = 0; 00158 } 00159 }
Generated on Wed Jul 13 2022 11:37:34 by
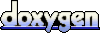