Not work original Lib on F446RE & L432KC . Change usage prime() & setNext() for both TX and RX function due to unexpected behavior (maybe os TimerEvent function problem)
Dependents: BufferedSoftSerial
SoftSerial.h
00001 /* 00002 Original Library by Erik- & SonyPony 00003 https://os.mbed.com/users/Sissors/code/SoftSerial/ 00004 00005 Modified by K.Arai / JH1PJL May 12th, 2020 00006 00007 modified functions 00008 tx_handler() 00009 prepare_tx() 00010 prime() 00011 rx_gpio_irq_handler() and others 00012 */ 00013 00014 #ifndef SOFTSERIAL_H 00015 #define SOFTSERIAL_H 00016 00017 #include "mbed.h" 00018 #include "SoftSerial_Ticker.h" 00019 00020 #define DEBUG_TIMING 0 // 0=no debug, 1=tx, 2=rx 00021 00022 #if (MBED_MAJOR_VERSION == 2) 00023 # define YIELD {;} 00024 #elif (MBED_MAJOR_VERSION == 5) 00025 # define YIELD {ThisThread::yield();} 00026 #endif 00027 00028 /** A software serial implementation 00029 * 00030 */ 00031 class SoftSerial: public Stream 00032 { 00033 00034 public: 00035 /** 00036 * Constructor 00037 * 00038 * @param TX Name of the TX pin, NC for not connected 00039 * @param RX Name of the RX pin, NC for not connected, 00040 * must be capable of being InterruptIn 00041 * @param name Name of the connection 00042 */ 00043 SoftSerial(PinName TX, PinName RX, const char* name = NULL); 00044 virtual ~SoftSerial(); 00045 00046 /** Set the baud rate of the serial port 00047 * 00048 * @param baudrate The baudrate of the serial port (default = 9600). 00049 */ 00050 void baud(int baudrate); 00051 00052 enum Parity { 00053 None = 0, 00054 Odd, 00055 Even, 00056 Forced1, 00057 Forced0 00058 }; 00059 00060 enum IrqType { 00061 RxIrq = 0, 00062 TxIrq 00063 }; 00064 00065 /** Set the transmission format used by the serial port 00066 * 00067 * @param bits The number of bits in a word (default = 8) 00068 * @param parity The parity used 00069 * (SoftSerial::None, SoftSerial::Odd, SoftSerial::Even, 00070 * SoftSerial::Forced1, SoftSerial::Forced0; default = SoftSerial::None) 00071 * @param stop The number of stop bits (default = 1) 00072 */ 00073 void format(int bits=8, Parity parity=SoftSerial::None, int stop_bits=1); 00074 00075 /** Determine if there is a character available to read 00076 * 00077 * @returns 00078 * 1 if there is a character available to read, 00079 * 0 otherwise 00080 */ 00081 int readable(); 00082 00083 /** Determine if there is space available to write a character 00084 * 00085 * @returns 00086 * 1 if there is space to write a character, 00087 * 0 otherwise 00088 */ 00089 int writeable(); 00090 00091 /** Attach a function to call whenever a serial interrupt is generated 00092 * 00093 * @param fptr A pointer to a void function, or 0 to set as none 00094 * @param type Which serial interrupt to attach the member function 00095 * to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00096 */ 00097 void attach(void (*fptr)(void), IrqType type=RxIrq) 00098 { 00099 fpointer[type] = Callback<void()>(fptr); 00100 } 00101 00102 /** Attach a member function to call 00103 * whenever a serial interrupt is generated 00104 * 00105 * @param tptr pointer to the object to call the member function on 00106 * @param mptr pointer to the member function to be called 00107 * @param type Which serial interrupt to attach the member function 00108 * to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00109 */ 00110 template<typename T> 00111 void attach(T* tptr, void (T::*mptr)(void), IrqType type=RxIrq) 00112 { 00113 fpointer[type] = Callback<void()>(tptr, mptr); 00114 } 00115 00116 /** Generate a break condition on the serial line 00117 */ 00118 void send_break(); 00119 00120 protected: 00121 DigitalOut *tx; 00122 InterruptIn *rx; 00123 00124 bool tx_en, rx_en; 00125 int bit_period; 00126 int _bits, _stop_bits, _total_bits; 00127 Parity _parity; 00128 00129 Callback<void()> fpointer[2]; 00130 00131 //rx 00132 void rx_gpio_irq_handler(void); 00133 void rx_handler(void); 00134 int read_buffer, rx_bit; 00135 volatile int out_buffer; 00136 volatile bool out_valid; 00137 bool rx_error; 00138 FlexTicker rxticker; 00139 00140 bool rx_1st; 00141 uint32_t rx_st_time; 00142 00143 //tx 00144 void tx_handler(void); 00145 void prepare_tx(int c); 00146 FlexTicker txticker; 00147 int _char; 00148 volatile int tx_bit; 00149 00150 virtual int _getc(); 00151 virtual int _putc(int c); 00152 }; 00153 00154 #endif
Generated on Thu Jul 21 2022 01:12:39 by
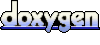