RX-8025NB Real Time Clock Module by EPSON
Dependents: TYBLE16_simple_data_logger Check_external_RTC
RX8025NB.h
00001 /* 00002 * mbed library program 00003 * Control RX-8025NB Real Time Clock Module 00004 * EPSON 00005 * 00006 * Copyright (c) 2015,'16,'17,'19,'20 Kenji Arai / JH1PJL 00007 * http://www7b.biglobe.ne.jp/~kenjia/ 00008 * https://os.mbed.com/users/kenjiArai/ 00009 * Created: June 3rd, 2015 00010 * Revised: August 7th, 2020 00011 */ 00012 00013 /* 00014 *---------------- REFERENCE --------------------------------------------------- 00015 * Original Information 00016 * http://www5.epsondevice.com/ja/products/rtc/rx8025nb.html 00017 * Akizuki 00018 * http://akizukidenshi.com/catalog/g/gK-08585/ 00019 */ 00020 00021 #ifndef RX8025_H 00022 #define RX8025_H 00023 00024 #include "mbed.h" 00025 00026 // RTC EPSON RX8025 00027 // 7bit address = 0b0110010(No other choice) 00028 #define RX8025ADDR (0x32 << 1) 00029 00030 // Register definition 00031 #define RX8025_REG_SEC 0x0 00032 #define RX8025_REG_MIN 0x1 00033 #define RX8025_REG_HOUR 0x2 00034 #define RX8025_REG_WDAY 0x3 00035 #define RX8025_REG_DAY 0x4 00036 #define RX8025_REG_MON 0x5 00037 #define RX8025_REG_YEAR 0x6 00038 #define RX8025_REG_OFFSET 0x7 00039 #define RX8025_REG_ALARMW_MIN 0x8 00040 #define RX8025_REG_ALARMW_HOUR 0x9 00041 #define RX8025_REG_ALARMW_WDAY 0xa 00042 #define RX8025_REG_ALARMD_MIN 0xb 00043 #define RX8025_REG_ALARMD_HOUR 0xc 00044 #define RX8025_REG_RESERVED 0xd 00045 #define RX8025_REG_CONTL1 0xe 00046 #define RX8025_REG_CONTL2 0xf 00047 00048 // Buffer size 00049 #define RTC_BUF_SIZ (RX8025_REG_CONTL2 + 5) 00050 00051 typedef enum { 00052 RTC_SQW_NONE = 0, 00053 RTC_SQW_32KHZ 00054 } sq_wave_t; 00055 00056 /** Interface for RTC (I2C Interface) EPSON RX8025 00057 * 00058 * Standalone type RTC via I2C interface 00059 * 00060 * @code 00061 * #include "mbed.h" 00062 * #include "RX8025NB.h" 00063 * 00064 * // I2C Communication 00065 * RX8025 RX8025(dp5,dp27); // RTC(RX8025) SDA, SCL (Fixed address) 00066 * // If you connected I2C line not only this device but also other devices, 00067 * // you need to declare following method. 00068 * I2C i2c(dp5,dp27); // SDA, SCL 00069 * RX8025 RX8025(i2c); // RTC(RX8025) (Fixed address) 00070 * 00071 * int main() { 00072 * tm t; 00073 * time_t seconds; 00074 * char buf[40]; 00075 * 00076 * while(1){ 00077 * RX8025.get_time_rtc(&t); // read RTC data 00078 * seconds = mktime(&t); 00079 * strftime(buf, 40, "%I:%M:%S %p (%Y/%m/%d)", localtime(&seconds)); 00080 * printf("Date: %s\r\n", buf); 00081 * } 00082 * } 00083 * @endcode 00084 */ 00085 00086 class RX8025 00087 { 00088 public: 00089 00090 typedef struct { // BCD format 00091 uint8_t rtc_seconds; 00092 uint8_t rtc_minutes; 00093 uint8_t rtc_hours; 00094 uint8_t rtc_weekday; 00095 uint8_t rtc_date; 00096 uint8_t rtc_month; 00097 uint8_t rtc_year_raw; 00098 uint16_t rtc_year; 00099 } rtc_time; 00100 00101 /** Configure data pin (with other devices on I2C line) 00102 * @param data SDA and SCL pins 00103 */ 00104 RX8025(PinName p_sda, PinName p_scl); 00105 00106 /** Configure data pin (with other devices on I2C line) 00107 * @param I2C previous definition 00108 */ 00109 RX8025(I2C& p_i2c); 00110 00111 /** Read RTC data with Standard C "struct tm" format 00112 * @param tm (data save area) 00113 * @return none but all data in tm 00114 */ 00115 void read_rtc_std(tm *); 00116 void get_time_rtc(tm *); 00117 00118 /** Write data to RTC data with Standard C "struct tm" format 00119 * @param tm (save writing data) 00120 * @return none but all data in tm 00121 */ 00122 void write_rtc_std(tm *); 00123 void set_time_rtc(tm *); 00124 00125 /** Set Alarm-D / INTA time 00126 * @param next time (unit: minutes) from now on minimum = 2 minutes!! 00127 * @return none 00128 */ 00129 void set_next_alarmD_INTA(uint16_t); 00130 void set_next_IRQ(uint16_t); 00131 00132 /** Clear Alarm-D / INTA interrupt 00133 * @param none 00134 * @return none 00135 */ 00136 void clear_alarmD_INTA(void); 00137 void clear_IRQ(void); 00138 00139 /** Read one byte from specific register 00140 * @param register address 00141 * @return register data 00142 */ 00143 uint8_t read_reg_byte(uint8_t); 00144 00145 /** Write one byte into specific register 00146 * @param register address, data 00147 * @return register saved data 00148 */ 00149 uint8_t write_reg_byte(uint8_t, uint8_t); 00150 00151 /** Read RTC data with own format 00152 * @param tm (data save area) 00153 * @return none but all data in tm 00154 */ 00155 void read_rtc_direct(rtc_time *); 00156 00157 /** Read RTC data with own format 00158 * @param tm (save writing data) 00159 * @return none but all data in tm 00160 */ 00161 void write_rtc_direct(rtc_time *); 00162 00163 /** Set I2C clock frequency 00164 * @param freq. 00165 * @return none 00166 */ 00167 void frequency(int); 00168 00169 /** Control FOUT output port (FOE pin must high when 32.768kHz output) 00170 * @param output_mode 00171 * @return none 00172 */ 00173 void set_sq_wave(sq_wave_t); 00174 00175 protected: 00176 I2C *_i2c_p; 00177 I2C &_i2c; 00178 00179 uint8_t bin2bcd(uint8_t); 00180 uint8_t bcd2bin(uint8_t); 00181 void set_alarmD_reg (uint16_t); 00182 void init(void); 00183 00184 private: 00185 uint8_t RX8025_addr; 00186 uint8_t rtc_buf[RTC_BUF_SIZ]; // buffer for RTC 00187 00188 }; 00189 00190 #endif // RX8025_H
Generated on Fri Jul 15 2022 07:35:33 by
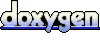