
Check RTC LSE(External Xtal, 32.768kHz) mode
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * mbed Application program 00003 * 00004 * Copyright (c) 2010-2015 Kenji Arai / JH1PJL 00005 * http://www.page.sannet.ne.jp/kenjia/index.html 00006 * http://mbed.org/users/kenjiArai/ 00007 * Created: March 27th, 2010 00008 * Revised: May 16th, 2015 00009 * 00010 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, 00011 * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00012 * AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00013 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00014 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00015 */ 00016 00017 // Include --------------------------------------------------------------------------------------- 00018 #include "mbed.h" 00019 #include "CheckRTC.h" 00020 00021 // Object ---------------------------------------------------------------------------------------- 00022 Serial pc(USBTX, USBRX); 00023 DigitalOut myled1(LED1); // Assign LED1 output port 00024 Timer t; 00025 00026 // RAM ------------------------------------------------------------------------------------------- 00027 00028 // ROM / Constant data --------------------------------------------------------------------------- 00029 00030 // Function prototypes --------------------------------------------------------------------------- 00031 int set_time_and_check_time(void); 00032 void msg_hlp (void); 00033 void chk_and_set_time(char *ptr); 00034 int xatoi (char **str, int32_t *res); 00035 void get_line (char *buff, int len); 00036 00037 // Definition ------------------------------------------------------------------------------------ 00038 #define BAUD(x) pc.baud(x) 00039 #define GETC(x) pc.getc(x) 00040 #define PUTC(x) pc.putc(x) 00041 #define PUTS(x) pc.puts(x) 00042 #define PRINTF(...) pc.printf(__VA_ARGS__) 00043 #define READABLE(x) pc.readable(x) 00044 00045 #define STYLE1 00046 //#define STYLE2 00047 //#define STYLE_COM 00048 00049 //------------------------------------------------------------------------------------------------- 00050 // Control Program 00051 //------------------------------------------------------------------------------------------------- 00052 #if defined(TARGET_NUCLEO_F401RE) || defined(TARGET_NUCLEO_F411RE) || defined(TARGET_NUCLEO_L152RE) 00053 int main() 00054 { 00055 uint32_t state; 00056 00057 while(true) { 00058 PUTS("\r\n\r\nCurrent Nucleo mbed Power-on sequence does NOT consider "); 00059 PUTS("External Xtal start-up.\r\n"); 00060 PUTS("Setting time is 5 seconds (improved) "); 00061 PUTS("but sometimes External Xtal 32.768KHz (LSE) does NOT work!\r\n"); 00062 PUTS("Current setting: "); 00063 state = get_RTCSEL(); 00064 switch (state) { 00065 case 0: // no clock 00066 PUTS("No clock"); 00067 break; 00068 case 1: // LSE 00069 PUTS("Use LSE(external Xtal)=32.768KHz"); 00070 break; 00071 case 2: // LSI 00072 PUTS("Use LSI(internal RC/Low speed), RC = 17 to 47, typ.32KHz"); 00073 break; 00074 case 3: // HSE 00075 PUTS("Use HSE(with prescaler)"); 00076 break; 00077 default: // Not come here 00078 PRINTF("No clock"); 00079 break; 00080 } 00081 PUTS("\r\n\r\n"); 00082 if (state == 1) { 00083 PUTS("LSE is using! No addtional effort but just in case please try follows.\r\n"); 00084 } else { 00085 PUTS("Please hit any key to use RTC external clock.\r\n"); 00086 while (!READABLE()) { 00087 wait(0.2); 00088 } 00089 PRINTF("\r\nStart measure\r\n"); 00090 t.reset(); 00091 t.start(); 00092 CheckRTC(); 00093 t.stop(); 00094 PRINTF("Start-up time was %f sec. (Time-out setting:%f sec.)\r\n", 00095 t.read(), (float)(float)TIMEOUT/1000); 00096 PUTS("\r\n"); 00097 state = get_RTCSEL(); 00098 switch (state) { 00099 case 0: // no clock 00100 PRINTF("No clock"); 00101 break; 00102 case 1: // LSE 00103 PRINTF("Use LSE"); 00104 break; 00105 case 2: // LSI 00106 PRINTF("Use LSI"); 00107 break; 00108 case 3: // HSE 00109 PRINTF("Use HSE"); 00110 break; 00111 default: // Not come here 00112 PRINTF("?"); 00113 break; 00114 } 00115 PRINTF("\r\n"); 00116 CheckRTC(); 00117 if (state == 1) { 00118 PUTS("Looks okay right now but always okay or not?!\r\n"); 00119 } 00120 } 00121 PUTS("If you would like to masure again,"); 00122 PUTS("Please remove JP6/IDO on the mbed board then re-plug it again!.\r\n\r\n"); 00123 set_time_and_check_time(); 00124 } 00125 } 00126 00127 #else 00128 #warning "Cannot use this function. Please comment out #define CHECK_RTC and use #define SET_RTC 00129 #endif 00130 00131 int set_time_and_check_time() 00132 { 00133 char *ptr; 00134 char linebuf[64]; 00135 char buf[40]; 00136 time_t seconds; 00137 00138 if (READABLE()) { 00139 GETC(); 00140 } 00141 seconds = time(NULL); 00142 PRINTF("Current time is "); 00143 #ifdef STYLE_COM 00144 PRINTF("Time: %s\r\n", ctime(&seconds)); 00145 #else 00146 strftime(buf,40, "%I:%M:%S %p (%Y/%m/%d)", localtime(&seconds)); 00147 PRINTF("Time: %s\r\n", buf); 00148 #endif 00149 PRINTF("Is it correct time?\r\n"); 00150 PRINTF("YES -> please enter '/'\r\n"); 00151 PRINTF("NO -> please enter t yy mm dd hh mm ss <ret> "); 00152 PRINTF("e.g. >t 15 5 17 10 5 15<ret>\r\n"); 00153 for (;;) { 00154 PUTC('\r'); 00155 PUTC('>'); 00156 ptr = linebuf; 00157 get_line(ptr, sizeof(linebuf)); 00158 switch (*ptr++) { 00159 //--------------------------------------------------------------------------------- 00160 // check and set RTC 00161 //--------------------------------------------------------------------------------- 00162 case 't' : 00163 PUTC('\r'); 00164 chk_and_set_time(ptr); 00165 break; 00166 //--------------------------------------------------------------------------------- 00167 // check and set RTC 00168 //--------------------------------------------------------------------------------- 00169 case '/' : 00170 PUTC('\r'); 00171 PRINTF("Current Time -> Plese see LCD also\r\n"); 00172 while (1) { 00173 if (READABLE()) { 00174 break; 00175 } 00176 while ( seconds == time(NULL)) ; 00177 seconds = time(NULL); 00178 myled1 = !myled1; 00179 #ifdef STYLE1 00180 // 17 May 2015 13:24:00 00181 strftime(buf,40, "%x %X ", localtime(&seconds)); 00182 #endif 00183 #ifdef STYLE2 00184 // 13:24:00 PM (2015/05/17) 00185 strftime(buf,40, "%I:%M:%S %p (%Y/%m/%d)", localtime(&seconds)); 00186 #endif 00187 #ifdef STYLE_COM 00188 PRINTF("Time: %s\r\n", ctime(&seconds)); 00189 #else 00190 PRINTF("Time: %s\r\n", buf); 00191 #endif 00192 } 00193 break; 00194 //--------------------------------------------------------------------------------- 00195 // check and set RTC 00196 //--------------------------------------------------------------------------------- 00197 case '?' : 00198 default : 00199 PUTC('\r'); 00200 msg_hlp(); 00201 break; 00202 } 00203 } 00204 } 00205 00206 // Help Massage 00207 void msg_hlp (void) 00208 { 00209 PUTS("t - Check and set RTC\r\n"); 00210 PUTS("/ - Show time every second (Esc -> hit any key)\r\n"); 00211 PUTS("? - Help\r\n"); 00212 } 00213 00214 // Change string -> number 00215 int xatoi (char **str, int32_t *res) 00216 { 00217 unsigned long val; 00218 unsigned char c, radix, s = 0; 00219 00220 while ((c = **str) == ' ') { 00221 (*str)++; 00222 } 00223 if (c == '-') { 00224 s = 1; 00225 c = *(++(*str)); 00226 } 00227 if (c == '0') { 00228 c = *(++(*str)); 00229 if (c <= ' ') { 00230 *res = 0; 00231 return 1; 00232 } 00233 if (c == 'x') { 00234 radix = 16; 00235 c = *(++(*str)); 00236 } else { 00237 if (c == 'b') { 00238 radix = 2; 00239 c = *(++(*str)); 00240 } else { 00241 if ((c >= '0')&&(c <= '9')) { 00242 radix = 8; 00243 } else { 00244 return 0; 00245 } 00246 } 00247 } 00248 } else { 00249 if ((c < '1')||(c > '9')) { 00250 return 0; 00251 } 00252 radix = 10; 00253 } 00254 val = 0; 00255 while (c > ' ') { 00256 if (c >= 'a') { 00257 c -= 0x20; 00258 } 00259 c -= '0'; 00260 if (c >= 17) { 00261 c -= 7; 00262 if (c <= 9) { 00263 return 0; 00264 } 00265 } 00266 if (c >= radix) { 00267 return 0; 00268 } 00269 val = val * radix + c; 00270 c = *(++(*str)); 00271 } 00272 if (s) { 00273 val = -val; 00274 } 00275 *res = val; 00276 return 1; 00277 } 00278 00279 // Get key input data 00280 void get_line (char *buff, int len) 00281 { 00282 char c; 00283 int idx = 0; 00284 00285 for (;;) { 00286 c = GETC(); 00287 if (c == '\r') { 00288 buff[idx++] = c; 00289 break; 00290 } 00291 if ((c == '\b') && idx) { 00292 idx--; 00293 PUTC(c); 00294 PUTC(' '); 00295 PUTC(c); 00296 } 00297 if (((uint8_t)c >= ' ') && (idx < len - 1)) { 00298 buff[idx++] = c; 00299 PUTC(c); 00300 } 00301 } 00302 buff[idx] = 0; 00303 PUTC('\n'); 00304 } 00305 00306 // RTC related subroutines 00307 void chk_and_set_time(char *ptr) 00308 { 00309 int32_t p1; 00310 struct tm t; 00311 time_t seconds; 00312 char buf[40]; 00313 00314 if (xatoi(&ptr, &p1)) { 00315 t.tm_year = (uint8_t)p1 + 100; 00316 PRINTF("Year:%d ",p1); 00317 xatoi( &ptr, &p1 ); 00318 t.tm_mon = (uint8_t)p1 - 1; 00319 PRINTF("Month:%d ",p1); 00320 xatoi( &ptr, &p1 ); 00321 t.tm_mday = (uint8_t)p1; 00322 PRINTF("Day:%d ",p1); 00323 xatoi( &ptr, &p1 ); 00324 t.tm_hour = (uint8_t)p1; 00325 PRINTF("Hour:%d ",p1); 00326 xatoi( &ptr, &p1 ); 00327 t.tm_min = (uint8_t)p1; 00328 PRINTF("Min:%d ",p1); 00329 xatoi( &ptr, &p1 ); 00330 t.tm_sec = (uint8_t)p1; 00331 PRINTF("Sec: %d\r\n",p1); 00332 seconds = mktime(&t); 00333 set_time(seconds); 00334 } 00335 seconds = time(NULL); 00336 strftime(buf, 40, "%B %d,'%y, %H:%M:%S", localtime(&seconds)); 00337 PRINTF("Time: %s\r\n", buf); 00338 }
Generated on Sun Jul 17 2022 00:30:58 by
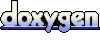